// This file defines the Building class // and the Hotel class that inherits from it. // A demonstration program declares and uses a Hotel object. class Building Declarations private string streetAddress private num squareFeet public string getAddress() return streetAddress public void getSquareFeet() return squareFt public void setSquareFeet() squareFeet = feet return public void setStreetAddress(string address) address = streetAddress return endClass class Hotel inheritsFrom Building Declarations private num numberOfRooms public Hotel(string address, num sqFt, num rooms) streetAddress = address squareFeet = sqFt numberOfRooms = rooms return public num getNumberOfRooms return rooms endClass start Declarations Hotel hotel("100 Main Street", 8000, 20) output getAddress(), getSquareFeet(), getNumberOfRooms() stop
// This file defines the Building class
// and the Hotel class that inherits from it.
// A demonstration program declares and uses a Hotel object.
class Building
Declarations
private string streetAddress
private num squareFeet
public string getAddress()
return streetAddress
public void getSquareFeet()
return squareFt
public void setSquareFeet()
squareFeet = feet
return
public void setStreetAddress(string address)
address = streetAddress
return
endClass
class Hotel inheritsFrom Building
Declarations
private num numberOfRooms
public Hotel(string address, num sqFt, num rooms)
streetAddress = address
squareFeet = sqFt
numberOfRooms = rooms
return
public num getNumberOfRooms
return rooms
endClass
start
Declarations
Hotel hotel("100 Main Street", 8000, 20)
output getAddress(), getSquareFeet(),
getNumberOfRooms()
stop

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

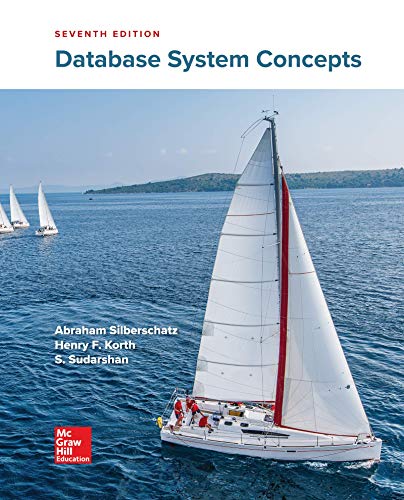
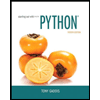
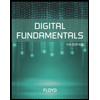
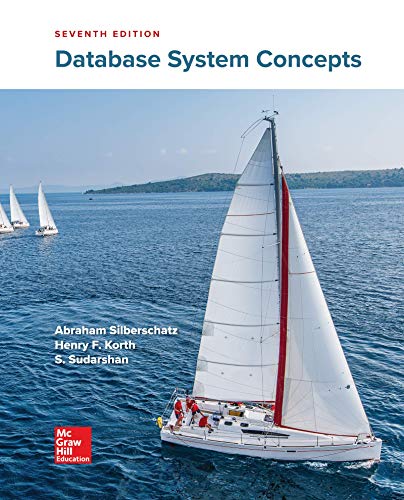
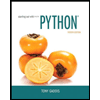
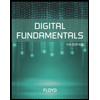
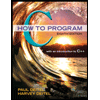
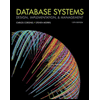
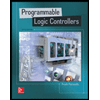