Java programming language I have to create a remove method that removes the element at an index (ind) or space in an array and returns it. Thanks!
Java programming language
I have to create a remove method that removes the element at an index (ind) or space in an array and returns it. Thanks!
I have to write the remove method in the code below. i attached the part where i need to write it.
public class ourArrayList<T>{
private Node<T> Head = null;
private Node<T> Tail = null;
private int size = 0;
//default constructor
public ourArrayList() {
Head = Tail = null;
size = 0;
}
public int size() {
return size;
}
public boolean isEmpty() {
return (size == 0);
}
//implement the method add, that adds to the back of the list
public void add(T e) {
//HW TODO and TEST
//create a node and assign e to the data of that node.
Node<T> N = new Node<T>();//N.mData is null and N.next is null as well
N.setsData(e);
//chain the new node to the list
//check if the list is empty, then deal with the special case
if(this.isEmpty()) {
//head and tail refer to N
this.Head = this.Tail = N;
size++;
//return we are done.
return;
}
//The list is not empty
//if the list is not empty. Then update the link of the last element to refer to the
//new node then update Tail to refer to the new node.
Tail.setNext(N);
Tail = N;
//increase size.
size++;
}
//remove removes the first/head and returns it.
public T remove() {
//check if empty then return null
if(this.isEmpty()) {
return null;
}
//it is not empty
//save the returned item
T save = this.Head.getData();
//Head should refer to the next node
this.Head = this.Head.getNext();
if(this.Head == null) {
this.Tail = null;
}
size--;
return save;
}
//T get(intind) returns the element at index ind without removing it.
public T get(int ind) {
//if list is empty return null
////if index is smaller than 0 or larger than size-1 return null
if(this.isEmpty() || ind < 0 || ind > size - 1) {
return null;
}
//create a temp reference, starting at Head.
Node<T> temp = this.Head;
//loop through the list ind times and return the data at the node.
for(int i = 0; i < ind; i++) {
//move to the next
temp = temp.getNext();
}
//temp should refer to the node you are looking for. return the data in that node
return temp.getData();
}
public void add(T e, int ind) {
//if ind is not valid return
if(ind < 0 || ind > size) {
return;
}
//if ind == size just call add(e), add it to the back
if(ind == size) {
//insert at the end just call add(e)
this.add(e);
return;
}
//create a new node with e as data
Node<T> N = new Node<T>(e);
//if ind == 0; //add it ot the head as a special case and return
if(ind == 0) {
N.setNext(this.Head);
Head = N;
size++;
return;
}
//The index is somewhere in between.
//Go to node ind - 1 and insert your Node.
Node<T> temp = this.Head;
for(int i =0; i<ind-1; i++) {
//go to next node
temp = temp.getNext();
}
//Set the next opf new node to next of temp
N.setNext(temp.getNext());
//set next of temp to new node
temp.setNext(N);
size++;
}
//TODO HW: remove the element at index ind and returns it. Test it.
public T remove (int ind) {
return null;
}
public String toString() {
if(this.isEmpty())
return "[]";
String st = "[";
//create a temp reference to the first node.
Node<T> temp = this.Head;
while(temp.getNext() != null) {
//go through all nodes until the last and add the content to the string.
st += temp.toString() + ", ";
//go to the next node
temp = temp.getNext();
}
//temp should poinrt to the last element at this point
st += temp.toString() + "]";
return st;
}
}


Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 3 images

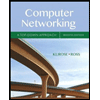
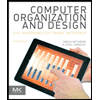
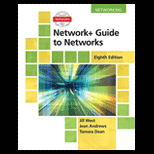
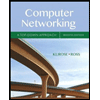
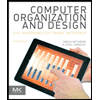
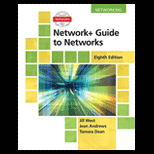
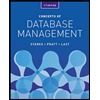
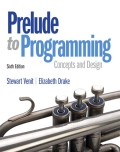
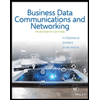