Add the method below to the parking office.java class. Method getParkingCharges(ParkingPermit) : Money Parkingoffice.java public class ParkingOffice { String name; String address; String phone; List customers; List cars; List lots; List charges; public ParkingOffice(){ customers = new ArrayList<>(); cars = new ArrayList<>(); lots = new ArrayList<>(); charges = new ArrayList<>(); } public Customer register() { Customer cust = new Customer(name,address,phone); customers.add(cust); return cust; } public Car register(Customer c,String licence, CarType t) { Car car = new Car(c,licence,t); cars.add(car); return car; } public Customer getCustomer(String name) { for(Customer cust : customers) if(cust.getName().equals(name)) return cust; return null; } public double addCharge(ParkingCharge p) { charges.add(p); return p.amount; } public String[] getCustomerIds(){ String[] stringArray1 = new String[2]; for(int i=0;i<2;i++){ stringArray1[i] = customers.get(i).customerId; } return stringArray1; } public String [] getPermitID () { String[] stringArray2 = new String[1]; for(int i=0;i<1;i++){ stringArray2[i] = charges.get(i).permitID; } return stringArray2; } public List getPermitIds(Customer c){ List permitid = new ArrayList(); for(Car car:cars){ if(car.owner == c.customerId) permitid.add(car.permit); } return permitid; } }
Add the method below to the parking office.java class.
Method
- getParkingCharges(ParkingPermit) : Money
Parkingoffice.java
public class ParkingOffice {
String name;
String address;
String phone;
List<Customer> customers;
List<Car> cars;
List<ParkingLot> lots;
List<ParkingCharge> charges;
public ParkingOffice(){
customers = new ArrayList<>();
cars = new ArrayList<>();
lots = new ArrayList<>();
charges = new ArrayList<>();
}
public Customer register() {
Customer cust = new Customer(name,address,phone);
customers.add(cust);
return cust;
}
public Car register(Customer c,String licence, CarType t) {
Car car = new Car(c,licence,t);
cars.add(car);
return car;
}
public Customer getCustomer(String name) {
for(Customer cust : customers)
if(cust.getName().equals(name))
return cust;
return null;
}
public double addCharge(ParkingCharge p) {
charges.add(p);
return p.amount;
}
public String[] getCustomerIds(){
String[] stringArray1 = new String[2];
for(int i=0;i<2;i++){
stringArray1[i] = customers.get(i).customerId;
}
return stringArray1;
}
public String [] getPermitID () {
String[] stringArray2 = new String[1];
for(int i=0;i<1;i++){
stringArray2[i] = charges.get(i).permitID;
}
return stringArray2;
}
public List<String> getPermitIds(Customer c){
List<String> permitid = new ArrayList<String>();
for(Car car:cars){
if(car.owner == c.customerId)
permitid.add(car.permit);
}
return permitid;
}
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

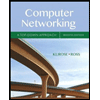
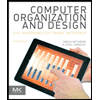
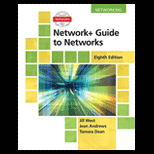
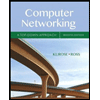
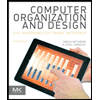
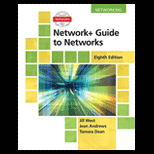
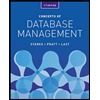
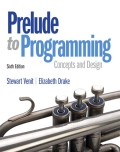
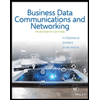