Write a code in Java programming: Suppose you have a functional interface like the following: interface MyFunction { List modify(List list); } Your colleague is sick, and he cannot finish his task. He gave you an unfinished code like the following: public static void main(String[] args) { List numbers = new ArrayList<>(Arrays.asList(1, 2, 3, 4, 5)); System.out.println("Before: " + numbers); // MyFunction mf = // TODO List modifiedList = mf.modify(numbers); System.out.println("After: " + modifiedList); } Your job is to complete the functional interface. The function takes a List of Integers and modifies it by performing the following operations: Remove any even numbers from the list. Square each number in the list. Thus if you complete the above code correctly, you should have an output like the below: Before: [1, 2, 3, 4, 5] After: [1, 9, 25]
Write a code in Java
Suppose you have a functional interface like the following:
interface MyFunction {
List<Integer> modify(List<Integer> list);
}
Your colleague is sick, and he cannot finish his task. He gave you an unfinished code like the following:
public static void main(String[] args) {
List<Integer> numbers = new ArrayList<>(Arrays.asList(1, 2, 3, 4, 5)); System.out.println("Before: " + numbers);
// MyFunction mf = // TODO
List<Integer> modifiedList = mf.modify(numbers); System.out.println("After: " + modifiedList);
}
Your job is to complete the functional interface. The function takes a List of Integers and modifies it by performing the following operations:
- Remove any even numbers from the list.
- Square each number in the list.
Thus if you complete the above code correctly, you should have an output like the below:
Before: [1, 2, 3, 4, 5]
After: [1, 9, 25]
Note that you should not change the method signature of the functional interface.

Step by step
Solved in 4 steps with 3 images

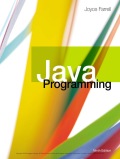
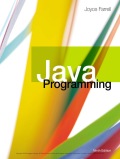