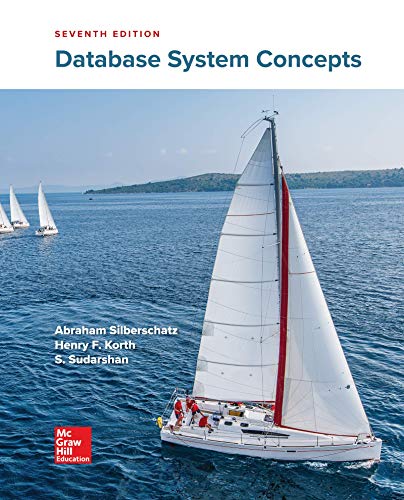
JAVA PROGRAM
PLEASE PLEASE PLEASE MODIFY THIS PROGRAM SO WHEN I UPLOAD IT TO HYPERGRADE IT PASSES THE TEST BECAUSE IT DOES NOT RUN IN HYPERGRADE. THEN TAKE OUT THE FOLLOWING FROM THE PROGRAM System.err.println("Error: " + filename + " is missing."); System.err.println("Error: Boynames.txt is missing."); and System.err.println("Error: Girlnames.txt is missing."); ALSO MAKE SURE IT PRINTS OUT THE FOLLOWING TEST CASE:
AnnabelleENTER
The name 'Annabelle' was not found in either list.\n
Enter a name to search or type QUIT to exit:\n
xavierENTER
The name 'Xavier' was found in popular boy names list (line 81).\n
Enter a name to search or type QUIT to exit:\n
AMANDAENTER
The name 'Amanda' was found in popular girl names list (line 63).\n
Enter a name to search or type QUIT to exit:\n
jOrdAnENTER
The name 'Jordan' was found in both lists: boy names (line 38) and girl names (line 75).\n
Enter a name to search or type QUIT to exit:\n
quitENTER
THE INPUTS ARE ALREADY IN HYPERGRADE SO MAKE SURE THIS PROGRAM PASSES THE TEST CASE WHEN I UPLOAD IT TO HYPERGRADE and take out the following from the program please: Boynames.txt is missing.\n Girlnames.txt is missing.\n Both data files are missing.\n THE TEST CASE DOES NOT ASK FOR IT. PLEASE REMOVE IT. I HAVE PROVIDED THE TEST CASE THAT FAILED AND THE INPUTS AS A SCREENSHOT.
import java.io.*;
import java.util.*;
public class NameSearcher {
private static Map<String, Integer> loadFileToMap(String filename) throws FileNotFoundException {
Map<String, Integer> namesMap = new HashMap<>();
File file = new File(filename);
try (Scanner scanner = new Scanner(file)) {
int lineNumber = 1;
while (scanner.hasNext()) {
String line = scanner.nextLine().trim().toLowerCase();
namesMap.put(line, lineNumber);
lineNumber++;
}
} catch (FileNotFoundException e) {
// Handle the exception properly
System.err.println("Error: " + filename + " is missing.");
}
return namesMap;
}
private static Integer searchNameInMap(String name, Map<String, Integer> namesMap) {
return namesMap.get(name.toLowerCase());
}
public static void main(String[] args) {
Map<String, Integer> boyNames = new HashMap<>();
Map<String, Integer> girlNames = new HashMap<>();
try {
boyNames = loadFileToMap("Boynames.txt");
} catch (FileNotFoundException e) {
// Handle the exception properly
System.err.println("Error: Boynames.txt is missing.");
}
try {
girlNames = loadFileToMap("Girlnames.txt");
} catch (FileNotFoundException e) {
// Handle the exception properly
System.err.println("Error: Girlnames.txt is missing.");
}
Scanner scanner = new Scanner(System.in);
while (true) {
System.out.print("Enter a name to search or type QUIT to exit:\n");
String input = scanner.nextLine().trim();
if (input.equalsIgnoreCase("QUIT")) {
break;
}
Integer boyIndex = searchNameInMap(input, boyNames);
Integer girlIndex = searchNameInMap(input, girlNames);
String capitalizedInput = Character.toUpperCase(input.charAt(0)) + input.substring(1).toLowerCase();
if (boyIndex == null && girlIndex == null) {
System.out.println("The name '" + capitalizedInput + "' was not found in either list.");
} else if (boyIndex != null && girlIndex == null) {
System.out.println("The name '" + capitalizedInput + "' was found in popular boy names list (line " + boyIndex + ").");
} else if (boyIndex == null && girlIndex != null) {
System.out.println("The name '" + capitalizedInput + "' was found in popular girl names list (line " + girlIndex + ").");
} else {
System.out.println("The name '" + capitalizedInput + "' was found in both lists: boy names (line " + boyIndex + ") and girl names (line " + girlIndex + ").");
}
}
scanner.close();
}
}
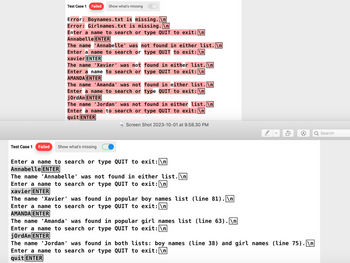
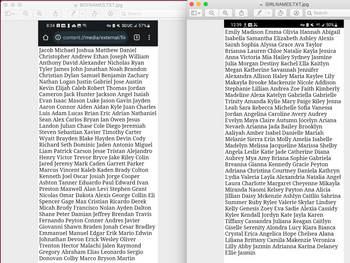

Step by stepSolved in 3 steps with 1 images

- Assume you are considering writing a method and are deciding what should happen when given input that is outside of perfect input. Which of the following is not a way to handle this? Group of answer choices 1. Use better JUnit testing 2. Throw an exception 3. Try to reasonably auto-correct 4. Prevent the errorarrow_forwardThe files provided in the code editor to the right contain syntax and/or logic errors. In each case, determine and fix the problem, remove all syntax and coding errors, and run the program to ensure it works properly. You also will use a file named DebugEmployeeIDException.java with the DebugTwelve4.java file. // An employee ID can't be more than 999 // Keep executing until user enters four valid employee IDs // This program throws a FixDebugEmployeeIDException import java.util.*; public class DebugTwelve4 { public static void main(String[] args) { Scanner input = new Scanner(System.in); String inStr, outString = ""; final int MAX = 999; int[] emps = new int[4]; for(int x = 0; x < emps.length; ++x) { System.out.println("Enter employee ID number"); inStr = input.next(); try { emps[x] = Integer.parseInt(inStr); if(emps[x] > MAX) { throw(new…arrow_forwardI have tried numerous codes to try to find a solution to this one. I continue to get error codes for the shopping cart. My guess it has something to do with the scanner? Here is the problem and what is pre-loaded into the program: Create a program using classes that does the following in the zyLabs developer below. For this lab, you will be working with two different class files. To switch files, look for where it says "Current File" at the top of the developer window. Click the current file name, then select the file you need.(1) Create two files to submit: ItemToPurchase.java - Class definition ShoppingCartPrinter.java - Contains main() method Build the ItemToPurchase class with the following specifications: Private fields String itemName - Initialized in default constructor to "none" int itemPrice - Initialized in default constructor to 0 int itemQuantity - Initialized in default constructor to 0 Default constructor Public member methods (mutators & accessors)…arrow_forward
- Please read all parts of this question before starting the question. Include the completed code for all parts of this question in a file called superheroes.py. NOTE: To implement encapsulation, all of the instance variables/fields should be considered private. Question 2 (a) Whenever folks on Earth get into trouble they look towards the Superheroes Federation to save them. Design and implement a Python class called Hero that includes the following class variable(s), instance variable(s) and method(s) for the class. Class variable(s): • TotalNumHeroes is the total number of heroes listed with the Federation • TotalNumChallengesMet is the total number of challenges that all the federation members have successfully overcome Attribute(s)/instance variable(s): • herolD is the unique ID number for the superhero (default value "N/A") • lifeName is the real-life name of the superhero (e.g. Bruce Wayne) (default value "N/A") • alterEgo is the secondary self or secret identity of the superhero…arrow_forwardJava Proram ASAP Please improve and adjust the program which is down below with the futher moddifications because it does not pass the test cases in Hypergrade. Please remove /n from the program and for test case 4 after this line: Please re-enter the file name or type QUIT to exit:\n quitENTER there needs to be nothing. import java.io.*;import java.util.Scanner;public class ConvertText { public static void main(String[] args) throws Exception { Scanner sc = new Scanner(System.in); System.out.println("Please enter the file name or type QUIT to exit:"); while (true) { String input = sc.next(); if (input.compareTo("QUIT") == 0) { break; } else { String filePath = new File("").getAbsolutePath(); filePath = filePath.concat("/"); filePath = filePath.concat(input); File file = new File(filePath); if (file.exists() && !file.isDirectory()) {…arrow_forward2. Modify the Fahrenheit program so that it displays a button that when pressed causes theconversion calculation to take place. The user will have the option of pressing Enter inthe text field or pressing the button. Have the listener that is already defined for the textfield also listen for the button push.arrow_forward
- JAVA PROGRAM Please Modify this program with further modifications as listed below: The program must pass the test case when uploaded to Hypergrade. ALSO, change the following in the program: System.out.print("Enter a name (or 'QUIT' to exit): "); to: Enter a name to search or type QUIT to exit and take out this: System.out.println(filename + " is missing. Exiting..."); import java.io.*;import java.util.*;public class Main { public static void main(String[] args) { List<String> girlsNames = loadNames("GirlNames.txt"); List<String> boysNames = loadNames("BoyNames.txt"); Scanner scanner = new Scanner(System.in); while (true) { System.out.print("Enter a name (or 'QUIT' to exit): "); String input = scanner.nextLine(); if (input.equalsIgnoreCase("QUIT")) { break; } searchAndDisplay(input, girlsNames, boysNames); } scanner.close(); } private static…arrow_forwardJava Proram ASAP There is an extra /n in the program in test case 1-3 and after Please re-enter the file name or type QUIT to exit:\n quitENTER in test case 4 there needs to be nothing as shown in the screenshot. The text files are located in Hypergrade. Please modify this code below so it passes the test cases. Also I have the correct test case as a screenshot. import java.io.*;import java.util.Scanner;public class ConvertText { public static void main(String[] args) throws Exception { Scanner sc = new Scanner(System.in); System.out.println("Please enter the file name or type QUIT to exit:"); while (true) { String input = sc.next(); if (input.compareTo("QUIT") == 0) { break; } else { String filePath = new File("").getAbsolutePath(); filePath = filePath.concat("/"); filePath = filePath.concat(input); File file = new File(filePath); if…arrow_forwardConsider the statementScanner a = new Scanner(System.in); Here Scanner is the class name, a is the name of object, new keyword is used to allocate the memory and System.in is the input stream.Following methods of Scanner classare used in the program below :-1) nextInt to input an integer2) nextFloat to input a float3) nextLine to input a stringJava programming source codearrow_forward
- Java Program ASAP ************This program must work in hypergrade and pass all the test cases.********** The program down below does not work in Hypergrade and does not pass the test cases. Please modify it even more or create a new program so when I upload to hypergrade it passes the test cases. Thank you! For Test Case 1 first print out Please enter the file name or type QUIT to exit:\ then you type text1.txtENTER and it displays Stop And Smell The Roses./n there needs to be nothing after that. For test case 2 first print out Please enter the file name or type QUIT to exit: then you type txt1.txtENTER then it reads out File 'txt1.txt' is not found.\n Then it didplays Please re-enter the file name or type QUIT to exit:\n after the test file is not found. then you type in text1.txt and it displays stop and smell the roses.\n. For test case 3 first print out Please enter the file name or type QUIT to exit: then you type text2.txtENTER and it displays A true rebel you are!…arrow_forwardStart with the code below and complete the getInt method. The method should prompt the user to enter an integer. Scan the input the user types. If the input is not an int, throw an IOException; otherwise, return the int. In the main program, invoke the getInt method, use try-catch block to catch the IOException. import java.util.*; import java.io.*; public class ReadInteger{ public static void main() { // your code goes here } public static int getInt() throws IOException { // your code goes here } }arrow_forwardneed main method that connects to a second method with user input and prints like the example below. the second method contains the followng, and both methods connect to an input txt file that has 45 games in the following format. number of games(blank line)game 1 namegame 1 platforms (separated by commas)game 1 year(blank line)etc. with game 2, game 3, and for number of games total gamesarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
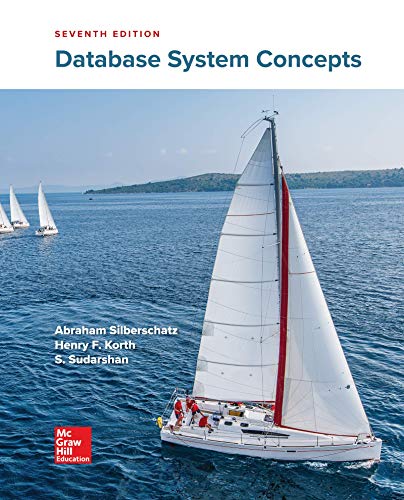
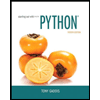
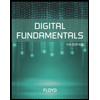
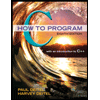
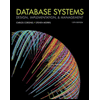
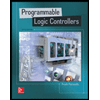