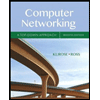
edit and finish class authenticate below do not give a solution (example copying from another source and giving it as a solution) that is not part of my code below. Also provided is user class.
This is what I have so far please help me finish it. The task is listed below
//Authenticate.java
import java.util.Scanner;
import java.io.File;
Class Authenticate {
private final int SIZE = 100;
private User() users = new User[SIZE];
public Authenticator (String fileName) throws Exception;
Scanner sc = new Scanner(new File(fileName));
int i = 0;
While(sc.hasNext() && i < SIZE) {
users[i] = Users.read(sc);
i++
}
}
public void authenticate(String username, String password) throws Exception{
try {
User u = null;
for(User X : users) {
if(x.getUsername().equals(username) && x.verifyPassword(password){
return ;
_________________________________________________________________
//User.java
import java.io.File;
import java.io.FileNotFoundException;
import java.util.Scanner;
public class User
{
private String username;
private String password;
private String hint;
public User(String username, String password, String hint)
{
this.username = username;
this.password = password;
this.hint = hint;
}
public boolean verifyPassword(String Password)
{
return Password.equals(password);
}
public String toString()
{
return "User "+username;
}
public static User read(Scanner scanner)
{
if(scanner.hasNext()){ }else {return null;}
return new User(scanner.next(), scanner.next(), scanner.next());
}
public String getUsername()
{
return username;
}
public String getHint()
{
return hint;
}
}
TASK
Implement the following class name Authenticator:
State
An array of type User (use a capacity of 100 — I would recommend using a class constant the way I did in the 06-Array class of Lecture 2).
An integer size
Behavior
A constructor accepting a file name, that opens a Scanner on the file and reads in User objects
A method named authenticate that accepts a username and password and attempts to authenticate them against the User array (by doing a search).
Not finding the username in the array causes an exception to be thrown
finding the username, but not matching the password (via verifyPassword) causes an exception with a different message to be thrown (this one with the password hint included).
See below for the exact exception messages expected
The return type of the method is void, i.e., the method returns nothing if the username and password are matched; otherwise an exception is thrown, as described above. (This is a common pattern for authentication methods — if everything is fine, the method simply returns, otherwise it throws an exception.)
The name of your class should be Authenticator. Please remove the public attribute from the class header.
Your class is tested by a AuthenticatorApp class that reads in Users from a file using your read method, loads them into an array and prompts the keyboard for a login sequence (username/password).
For example, if the file users.data contains:
weiss puppy2 woof-woof
arnow java cuppa
sokol brooklyn college
here are some sample excutions of the program:
username? arnow
password? java
Welcome to the system
Sample Test Run #2
Given the same users.data file as above, execution of the program should look like:
username? weiss
password? dontremember
*** Invalid password - hint: woof-woof
username? weiss
password? puppy2
Welcome to the system
Sample Test Run #3
Given the same users.data file as above, execution of the program should look like:
username? sokol
password? CUNY
*** Invalid password - hint: college
username? sokol
password? SUNY
*** Invalid password - hint: college
username? sokol
password? BC
*** Invalid password - hint: college
Too many failed attempts... please try again later

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- Statement that increases numPeople by 5. Ex: If numPeople is initially 10, the output is: There are 15 people.arrow_forwardWrite in Javaarrow_forwardSee ERROR in Java file 2: Java file 1: import java.util.Random;import java.util.Scanner; public class Account {// Declare the variablesprivate String customerName;private String accountNumber;private double balance;private int type;private static int numObjects;// constructorpublic Account(String customerName, double balance, int type) { this.customerName = customerName;this.balance = balance;this.type = type;setaccountNumber(); // set account number functionnumObjects += 1;}private void setaccountNumber() // definition of set account number{Random rand = new Random();accountNumber = customerName.substring(0, 3).toUpperCase();accountNumber += type;accountNumber += "#";accountNumber += (rand.nextInt(100) + 100);}// function to makedepositvoid makeDeposit(double amount){if (amount > 0){balance += amount;}}// function for withdrawboolean makeWithdrawal(double amount) {if (amount < balance)balance -= amount;elsereturn false;return true;}public String toString(){return accountNumber +…arrow_forward
- Don't give me AI generated answer or plagiarised answer. If I see these things I'll give you multiple downvotes and will report immediately.arrow_forward6. Design a Java class with a main method that does the following. You must include a meaningful comment for main and each method a. Main invokes a method readScores to read input from a file (name of your choice) that contains the name of the bowlers (no more than 25) and their bowling scores (two scores per bowler). The program stops reading values when it has reached the end of the file. For example, the file might contain: Bob 203 176 Jane 210 253 Jack 300 189 Eileen 220 298 • As the method reads the data, it stores the name into a String array and the average of the two bowling scores into an array of double. • The method returns the actual number of bowlers read in b. Main then invokes a method computeOverallAvg to: compute (a) the overall average score of all the bowlers combined, (b) the average score and name of the bowler with the highest average score and (c) the average score and name of the bowler with the lowest average scores. print to the console (a) the overall average…arrow_forwardquestion 9 and 10arrow_forward
- In java ****arrow_forwardx = 3 + numbers[3]++; System.out.println(x); QUESTION 2: Class Rectangle has two data members, length (type of float) and width (type of float). The class Rectangle also has the following: //mutator methods void setlength (float len) } //B. length = len; H accessor methods float getLength() I return length; The following statement creates an array of Rectangle with size 2: Rectangle[] arrayRectangle= new Rectangle[2]; Is it correct if executing the following statements? What is the output? If it is not correct, write the correct one arrayRectangle. setLength (12.59); System.out.printin[rectangle Set.getLength()); arrayRectangle[1].setLength(4.15); System.out.println(arrayRectangle[1].getLength()): QUESTION 3: Use the following int array int[] numbers = { 42, 28, 36, 72, 17, 25, 81, 65, 23, 58} -Open file data.txt to write. to write the values of the array numbers to the file with the y numbers to get the valuesarrow_forward5. Declare a class called coordinate. This is to represent 3 dimensional Cartesian coordinates (x, y and z).Define the following methods cumulatively in Java cumulatively:a. Constructorb. Display, to print values of membersc. Add_coordinates, to add three such coordinate objects to produce a resultant coordinate object.Generate and handle exceptions if x, y and z coordinate of the result are zero.d. Main, to show use of the above methods.arrow_forward
- PLZ help with the following: True/False In java it is possible to throw an exception, catch it, then re-throw that same exception if it is desired GUIs are windowing interfaces that handle user input and output. An interface can contain defined constants as well as method headings or instead of method headings. When a recursive call is encountered, computation is temporarily suspended; all of the information needed to continue the computation is saved and the recursive call is evaluated. Can you have a static method in a nonstatic inner class?arrow_forwardCan you please help me with this, its in java. Thank you. Write classes in an inheritance hierarchy Implement a polymorphic method Create an ArrayList object Use ArrayList methods For this, please design and write a Java program to keep track of various menu items. Your program will store, display and modify salads, sandwiches and frozen yogurts. Present the user with the following menu options: Actions: 1) Add a salad2) Add a sandwich3) Add a frozen yogurt4) Display an item5) Display all items6) Add a topping to an item9) QuitIf the user does not enter one of these options, then display:Sorry, <NUMBER> is not a valid option.Where <NUMBER> is the user's input. All menu items have the following: Name (String) Price (double) Topping (StringBuilder or StringBuffer of comma separated values) In addition to everything that a menu item has, a main dish (salad or sandwich) also has: Side (String) A salad also has: Dressing (String) A sandwich also has: Bread type…arrow_forwardAnswer correctly with explaination No copy paste pleasearrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
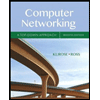
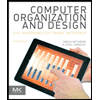
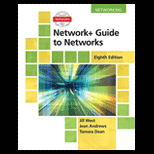
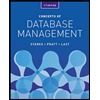
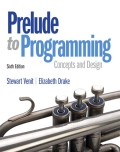
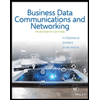