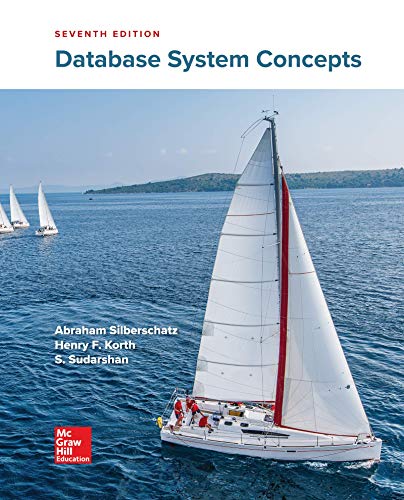
Concept explainers
Using switch statements, build a Java class file to calculate the area of a figure (call it Area.java). The figure may be a
circle or a square
or a rectangle. The user will enter the type of figure they want through a Scanner object. It they
enter “Circle”,
proceed to calculate the area of a Circle using values your request for radius. If they enter an
“Square”, then
calculate the area of the circle using values you request for side. If they enter an “Radius”, then
calculate using
values you request for height and width.

logic:-
read user’s choice.
Pass user’s choice within switch
define three cases circle, square and rectangle
Read radius for circle, side for square , and height and width for rectangle.
Write area formula for each case.
Display area.
End.
Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

- Challenge 2: InvalidNumbers.java Write a program InvalidNumbers that asks the user to enter a positive integer. The program will warn the user if a valid integer is not entered using the InputMismatchException and ask to enter again. The program should also throw an exception if the user enters negative numbers. Write the program and test it using negative numbers, doubles, and very large numbers.arrow_forwardIN JAVA "New Employee Gross Salary Calculator" Write a method that accepts an employee's yearly salary and start date and calculates their gross salary from the provided start date up through the end of the year Employees always start on the first of the month Throw an IllegalArgumentException if the provided start date is not the first of the month Employees qualify for a 3% pay raise 3 months after their start date and another 6% 3 months after that Use appropriate data typearrow_forwardCreate methods "read" and "write" methods to handle the input and output to files. in Javaarrow_forward
- Instructions: For each Exercise below, write your code in an IDE and run your code within the IDE as well. Once you have satisfied the Exercise requirements, paste your code below under the corresponding. Exercise 1:The Magic 8-Ball is a super popular toy used for fortune-telling or seeking advice developed in the 1950s! Write a magic8.cpp program that will output a random fortune each time it executes. The answers inside a standard Magic 8-Ball are: ● It is certain ● ● ● . ● ● ● ● ● It is decidedly so Without a doubt Yes - definitely You may rely on it As I see it, yes Most likely Outlook good Yes Signs point to yes Reply hazy, try again Ask again later Better not tell you now Cannot predict now Concentrate and ask again Don't count on it My reply is no . My sources say no ● Outlook not so good ● Very doubtfularrow_forwardJava Proram ASAP Please look closely at the circled parts in the failed test case. Improve and adjust the program which is down below with the futher moddifications because it does not pass the test cases in Hypergrade. Please remove /n from the program and for test case 4 after this line: Please re-enter the file name or type QUIT to exit:\n quitENTER there needs to be nothing. Also, I do not need file quit is no found in the program. import java.io.*;import java.util.Scanner;public class ConvertText { public static void main(String[] args) throws Exception { Scanner sc = new Scanner(System.in); System.out.println("Please enter the file name or type QUIT to exit:"); while (true) { String input = sc.next(); if (input.compareTo("QUIT") == 0) { break; } else { // Get the absolute path of the current directory String filePath = new File("").getAbsolutePath(); filePath =…arrow_forwardJava Program ASAP ************This program must work in hypergrade and pass all the test cases.********** The program down below does not work in Hypergrade and does not pass the test cases. For Test Case 1 first print out Please enter the file name or type QUIT to exit:\ then you type text1.txtENTER and it displays Stop And Smell The Roses./n there needs to be nothing after that. For test case 2 first print out Please enter the file name or type QUIT to exit: then you type txt1.txtENTER then it reads out File 'txt1.txt' is not found.\n Then it didplays Please re-enter the file name or type QUIT to exit:\n after the test file is not found. then you type in text1.txt and it displays stop and smell the roses.\n. For test case 3 first print out Please enter the file name or type QUIT to exit: then you type text2.txtENTER and it displays A true rebel you are! Everyone was impressed. You'll do well to continue in the same spirit.\nPlease explain a bit more in the way of…arrow_forward
- The files provided in the code editor to the right contain syntax and/or logic errors. In each case, determine and fix the problem, remove all syntax and coding errors, and run the program to ensure it works properly. You also will use a file named DebugEmployeeIDException.java with the DebugTwelve4.java file. // An employee ID can't be more than 999 // Keep executing until user enters four valid employee IDs // This program throws a FixDebugEmployeeIDException import java.util.*; public class DebugTwelve4 { public static void main(String[] args) { Scanner input = new Scanner(System.in); String inStr, outString = ""; final int MAX = 999; int[] emps = new int[4]; for(int x = 0; x < emps.length; ++x) { System.out.println("Enter employee ID number"); inStr = input.next(); try { emps[x] = Integer.parseInt(inStr); if(emps[x] > MAX) { throw(new…arrow_forwardJAVA PPROGRAM Please Modify this program with further modifications as listed below: ALSO, take out and change the following in the program: System.out.println("Program terminated."); because the test case does not need it And change this in the program: Please enter the file name or type QUIT to exit:\n so it reperats once for every test case because it repeats twice in every case as shown in the screenshot. I only need it once. I have provided the failed the test cases and the inputs as a screenshot. The program must pass the test case when uploaded to Hypergrade. import java.io.*;import java.util.ArrayList;import java.util.Scanner;public class SymmetricalNameMatcher { public static void main(String[] args) { Scanner scanner = new Scanner(System.in); String fileName; do { // Prompt the user to enter a file name or 'QUIT' to exit. System.out.print("Please enter the file name or type QUIT to exit:\n"); fileName =…arrow_forwardWrite an application that prompts for and reads the numeratorand denominator of a fraction as integers and then prints the decimal equivalent of the fraction.arrow_forward
- For the following program in phyton : Pay CheckWindow CostWrite an interactive program to calculate the cost of glasswork and metalstripping for rectangular windows. All windows, no matter what size, use thesame quality of glass and aluminum strips. Prompt the user for his/her name,age, length, and width of a window, then calculate the costs shown based on theinformation read in from the windowinfo.txt file which contains the materialstypes and costs (you cannot change this file at all). There is a discount on thetotal cost of 10% if the total area is over 1000 square inches, and 15% if it isover 1500 square inches.*Be sure to include a try/except and any and all string methods you might need*No conditional loops can be used at all**This is the info you will see in the text file, remember you mustread this in and store it:Glass: 80 cents per square inch (unit area)Aluminum: $1.25 per inch (unit length) This is the sample output. Write it to both the screen and a text file…arrow_forwardX609: Magic Date A magic date is one when written in the following format, the month times the date equals the year e.g. 6/10/60. Write code that figures out if a user entered date is a magic date. The dates must be between 1 - 31, inclusive and the months between 1 - 12, inclusive. Let the user know whether they entered a magic date. If the input parameters are not valid, return false. Examples: magicDate(6, 10, 60) -> true magicDate(50, 12, 600) –> falsearrow_forwardWrite a java code that does the following: Opens a file named NumberList.txt, uses a loop to write the numbers 1 through 100 to the file, and then closes the file. Opens the NumberList.txt file and reads all of the numbers from the file and displays them, and then closes the file. Opens the NumberList.txt file and reads all of the numbers from the file, calculate the sum of these numbers and displays their total. Opens a file named NumberList.txt for writing, but does not erase the contents of the file if it already exists.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
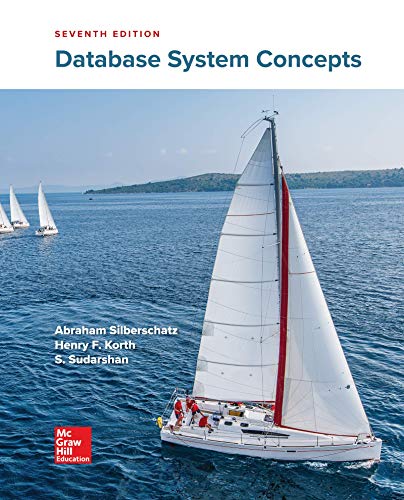
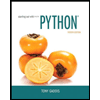
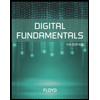
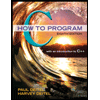
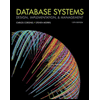
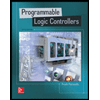