JAVA PROGRAM Please Modify this program with further modifications as listed below: The program must pass the test case when uploaded to Hypergrade. ALSO, change the following in the program: System.out.print("Enter a name (or 'QUIT' to exit): "); to: Enter a name to search or type QUIT to exit and take out this: System.out.println(filename + " is missing. Exiting..."); import java.io.*; import java.util.*; public class Main { public static void main(String[] args) { List girlsNames = loadNames("GirlNames.txt"); List boysNames = loadNames("BoyNames.txt"); Scanner scanner = new Scanner(System.in); while (true) { System.out.print("Enter a name (or 'QUIT' to exit): "); String input = scanner.nextLine(); if (input.equalsIgnoreCase("QUIT")) { break; } searchAndDisplay(input, girlsNames, boysNames); } scanner.close(); } private static List loadNames(String filename) { List names = new ArrayList<>(); try (BufferedReader reader = new BufferedReader(new FileReader(filename))) { String line; while ((line = reader.readLine()) != null) { names.add(line.trim()); } } catch (IOException e) { System.out.println(filename + " is missing. Exiting..."); System.exit(1); // Exit the program if a file is missing } return names; } private static void searchAndDisplay(String name, List girlsNames, List boysNames) { int girlIndex = searchName(name, girlsNames); int boyIndex = searchName(name, boysNames); String capitalizedName = name.substring(0, 1).toUpperCase() + name.substring(1).toLowerCase(); if (girlIndex == -1 && boyIndex == -1) { System.out.println("The name '" + capitalizedName + "' was not found in either list."); } else if (girlIndex != -1 && boyIndex == -1) { System.out.println("The name '" + capitalizedName + "' was found in popular girl names list (line " + (girlIndex + 1) + ")."); } else if (girlIndex == -1 && boyIndex != -1) { System.out.println("The name '" + capitalizedName + "' was found in popular boy names list (line " + (boyIndex + 1) + ")."); } else { System.out.println("The name '" + capitalizedName + "' was found in both lists: boy names (line " + (boyIndex + 1) + ") and girl names (line " + (girlIndex + 1) + ")."); } } private static int searchName(String name, List namesList) { for (int i = 0; i < namesList.size(); i++) { if (namesList.get(i).equalsIgnoreCase(name)) { return i; } } return -1; } } THE TEXT FILES ARE LOCATED IN HYPERGRADE I provided them and the failed test case as a screenshot. Thank you. Test Case 1 Enter a name to search or type QUIT to exit:\n AnnabelleENTER The name 'Annabelle' was not found in either list.\n Enter a name to search or type QUIT to exit:\n xavierENTER The name 'Xavier' was found in popular boy names list (line 81).\n Enter a name to search or type QUIT to exit:\n AMANDAENTER The name 'Amanda' was found in popular girl names list (line 63).\n Enter a name to search or type QUIT to exit:\n jOrdAnENTER The name 'Jordan' was found in both lists: boy names (line 38) and girl names (line 75).\n Enter a name to search or type QUIT to exit:\n quitENTER
System.out.print("Enter a name (or 'QUIT' to exit): "); to: Enter a name to search or type QUIT to exit and take out this: System.out.println(filename + " is missing. Exiting...");
import java.io.*;
import java.util.*;
public class Main {
public static void main(String[] args) {
List<String> girlsNames = loadNames("GirlNames.txt");
List<String> boysNames = loadNames("BoyNames.txt");
Scanner scanner = new Scanner(System.in);
while (true) {
System.out.print("Enter a name (or 'QUIT' to exit): ");
String input = scanner.nextLine();
if (input.equalsIgnoreCase("QUIT")) {
break;
}
searchAndDisplay(input, girlsNames, boysNames);
}
scanner.close();
}
private static List<String> loadNames(String filename) {
List<String> names = new ArrayList<>();
try (BufferedReader reader = new BufferedReader(new FileReader(filename))) {
String line;
while ((line = reader.readLine()) != null) {
names.add(line.trim());
}
} catch (IOException e) {
System.out.println(filename + " is missing. Exiting...");
System.exit(1); // Exit the program if a file is missing
}
return names;
}
private static void searchAndDisplay(String name, List<String> girlsNames, List<String> boysNames) {
int girlIndex = searchName(name, girlsNames);
int boyIndex = searchName(name, boysNames);
String capitalizedName = name.substring(0, 1).toUpperCase() + name.substring(1).toLowerCase();
if (girlIndex == -1 && boyIndex == -1) {
System.out.println("The name '" + capitalizedName + "' was not found in either list.");
} else if (girlIndex != -1 && boyIndex == -1) {
System.out.println("The name '" + capitalizedName + "' was found in popular girl names list (line " + (girlIndex + 1) + ").");
} else if (girlIndex == -1 && boyIndex != -1) {
System.out.println("The name '" + capitalizedName + "' was found in popular boy names list (line " + (boyIndex + 1) + ").");
} else {
System.out.println("The name '" + capitalizedName + "' was found in both lists: boy names (line " + (boyIndex + 1) + ") and girl names (line " + (girlIndex + 1) + ").");
}
}
private static int searchName(String name, List<String> namesList) {
for (int i = 0; i < namesList.size(); i++) {
if (namesList.get(i).equalsIgnoreCase(name)) {
return i;
}
}
return -1;
}
}
THE TEXT FILES ARE LOCATED IN HYPERGRADE I provided them and the failed test case as a screenshot. Thank you.
Test Case 1
Enter a name to search or type QUIT to exit:\n
AnnabelleENTER
The name 'Annabelle' was not found in either list.\n
Enter a name to search or type QUIT to exit:\n
xavierENTER
The name 'Xavier' was found in popular boy names list (line 81).\n
Enter a name to search or type QUIT to exit:\n
AMANDAENTER
The name 'Amanda' was found in popular girl names list (line 63).\n
Enter a name to search or type QUIT to exit:\n
jOrdAnENTER
The name 'Jordan' was found in both lists: boy names (line 38) and girl names (line 75).\n
Enter a name to search or type QUIT to exit:\n
quitENTER



Step by step
Solved in 3 steps with 1 images

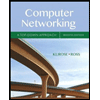
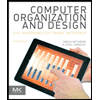
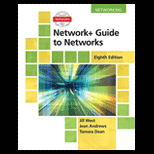
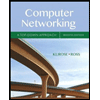
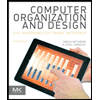
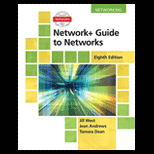
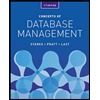
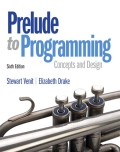
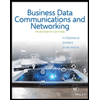