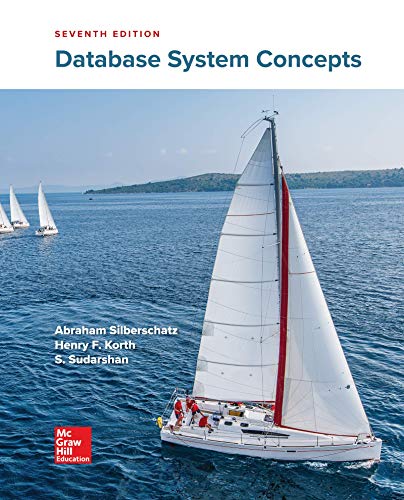
Java Program
Fix this Rock, Paper and scissor program so I can upload it to Hypergrade and it can pass all the test cases.
Here is the program:
import java.util.Random;
import java.util.Scanner;
public class RockPaperScissors {
public static void main(String[] args) {
if (args.length != 1) {
System.out.println("Please provide a seed as a command line argument.");
return;
}
long seed = Long.parseLong(args[0]);
Random random = new Random(seed);
Scanner scanner = new Scanner(System.in);
System.out.println("Enter 1 for rock, 2 for paper, and 3 for scissors.");
do {
int computerChoice = random.nextInt(3);
int userChoice = getUserChoice(scanner);
if (userChoice == -1) {
continue;
}
System.out.println("Your choice: " + choiceToString(userChoice) + ". Computer choice: " + choiceToString(computerChoice) + ".");
int result = determineWinner(userChoice, computerChoice);
if (result == 0) {
System.out.println("It's a draw.");
} else if (result == 1) {
System.out.println("Computer wins.");
} else {
System.out.println("You win.");
}
System.out.println("Would you like to play more? (yes/no)");
} while (scanner.next().equalsIgnoreCase("yes"));
System.out.println("Thanks for playing!");
scanner.close();
}
public static int getUserChoice(Scanner scanner) {
int choice;
while (true) {
System.out.print("Enter your choice: ");
if (scanner.hasNextInt()) {
choice = scanner.nextInt();
if (choice >= 1 && choice <= 3) {
break;
} else {
System.out.println("Please respond 1, 2, or 3.");
}
} else {
scanner.next(); // Consume invalid input
System.out.println("Please respond 1, 2, or 3.");
}
}
return choice - 1; // Map to 0, 1, 2 internally
}
public static String choiceToString(int choice) {
switch (choice) {
case 0:
return "rock";
case 1:
return "paper";
case 2:
return "scissors";
default:
return "invalid";
}
}
public static int determineWinner(int userChoice, int computerChoice) {
if (userChoice == computerChoice) {
return 0; // Draw
} else if ((userChoice + 1) % 3 == computerChoice) {
return 1; // Computer wins
} else {
return -1; // User wins
}
}
}
Test Case 1
1ENTER
Your choice: rock. Computer choice: paper.\n
Computer wins.\n
Would you like to play more?\n
nENTER
Test Case 2
2ENTER
Your choice: paper. Computer choice: paper.\n
It's a draw.\n
Would you like to play more?\n
nENTER
Test Case 3
3ENTER
Your choice: scissors. Computer choice: paper.\n
You win.\n
Would you like to play more?\n
nENTER
Test Case 4
0ENTER
Please respond 1, 2, or 3.\n
Enter 1 for rock, 2 for paper, and 3 for scissors.\n
1ENTER
Your choice: rock. Computer choice: paper.\n
Computer wins.\n
Would you like to play more?\n
nENTER

Algorithm: Rock, Paper, Scissors Game
1. Start the program.
2. Check if a seed is provided as a command line argument.
- If no seed is provided, print "Please provide a seed as a command line argument." and exit the program.
3. Parse the provided seed as a long integer.
4. Initialize a random number generator with the parsed seed.
5. Create a Scanner object to read user input.
6. Print "Enter 1 for rock, 2 for paper, and 3 for scissors."
7. Enter a loop to play the game until the user chooses to quit:
a. Generate a random choice for the computer (an integer between 1 and 3).
b. Get the user's choice by calling getUserChoice(scanner).
- Repeat until a valid choice (1, 2, or 3) is entered.
c. Print the user's choice and the computer's choice.
d. Determine the winner by calling determineWinner(userChoice, computerChoice):
- If userChoice == computerChoice, set result to 0 (draw).
- If (userChoice + 1) % 3 == computerChoice, set result to 1 (computer wins).
- Otherwise, set result to 2 (user wins).
e. Print the result:
- If result == 0, print "It's a draw."
- If result == 1, print "Computer wins."
- If result == 2, print "You win."
f. Ask the user if they want to play more (yes/no).
g. If the user enters "no" (case-insensitive), exit the loop.
8. Print "Thanks for playing!" to indicate the end of the game.
9. Close the Scanner object.
10. End the program.
Function getUserChoice(scanner):
1. Initialize choice to -1.
2. Enter a loop:
a. Print "Enter your choice: ".
b. Read an integer from the scanner.
c. If the input is an integer:
- If the input is between 1 and 3 (inclusive), break the loop.
- Otherwise, print "Please respond 1, 2, or 3."
d. If the input is not an integer, consume the invalid input and print "Please respond 1, 2, or 3."
3. Return the user's choice.
Function determineWinner(userChoice, computerChoice):
1. If userChoice equals computerChoice, return 0 (draw).
2. If (userChoice + 1) % 3 equals computerChoice, return 1 (computer wins).
3. Otherwise, return 2 (user wins).
Step by stepSolved in 4 steps with 3 images

- StringFun.java import java.util.Scanner; // Needed for the Scanner class 2 3 /** Add a class comment and @tags 4 5 */ 6 7 public class StringFun { /** * @param args not used 8 9 10 11 12 public static void main(String[] args) { Scanner in = new Scanner(System.in); System.out.print("Please enter your first name: "); 13 14 15 16 17 18 System.out.print("Please enter your last name: "); 19 20 21 //Output the welcome message with name 22 23 24 //Output the length of the name 25 26 27 //Output the username 28 29 30 //Output the initials 31 32 33 //Find and output the first name with switched characters 34 //All Done! } } 35 36 37arrow_forwardI need help with this java problem described below: import java.util.Scanner; import java.util.InputMismatchException; public class ExpirationMonth { public static void main(String[] args) { Scanner scnr = new Scanner(System.in); int expirationMonth; boolean valueFound = false; while (!valueFound) { try { expirationMonth = scnr.nextInt(); valueFound = true; System.out.println("Expiration month: " + expirationMonth); System.out.println("Processed one valid input value"); } /* Your code goes here */ } } }arrow_forwardImplement the following in the .NET Console App. Write the Bus class. A Bus has a length, a color, and a number of wheels. a. Implement data fields using auto-implemented Properies b. Include 3 constructors: default, the one that receives the Bus length, color and a number of wheels as input (utilize the Properties) and the Constructor that takes the Bus number (an integer) as input.arrow_forward
- need help finishing/fixing this java code so it prints out all the movie names that start with the first 2 characters the user inputs the code is as follows: Movie.java: import java.io.File;import java.io.FileNotFoundException;import java.util.ArrayList;import java.util.Scanner;public class Movie{public String name;public int year;public String genre;public static ArrayList<Movie> loadDatabase() throws FileNotFoundException {ArrayList<Movie> result=new ArrayList<>();File f=new File("db.txt");Scanner inputFile=new Scanner(f);while(inputFile.hasNext()){String name= inputFile.nextLine();int year=inputFile.nextInt();inputFile.nextLine();String genre= inputFile.nextLine();Movie m=new Movie(name, year, genre);//System.out.println(m);result.add(m);}return result;}public Movie(String name, int year, String genre){this.name=name;this.year=year;this.genre=genre;}public boolean equals(int year, String genre){return this.year==year&&this.genre.equals(genre);}public String…arrow_forwardI need help fixing this java program as shown below: import java.util.Scanner;import java.util.InputMismatchException; public class NameAgeChecker { public static void main(String[] args) { Scanner scnr = new Scanner(System.in); String inputName; int age; inputName = scnr.next(); while (!inputName.equals("-1")) { // FIXME: The following line will throw an InputMismatchException. // Insert a try/catch statement to catch the exception. age = scnr.nextInt(); System.out.println(inputName + " " + (age + 1)); inputName = scnr.next(); } }}arrow_forwardI need help with this Java Problem as described in the image below: import java.util.Scanner; public class LabProgram { public static int fibonacci(int n) { /* Type your code here. */ } public static void main(String[] args) { Scanner scnr = new Scanner(System.in); int startNum; startNum = scnr.nextInt(); System.out.println("fibonacci(" + startNum + ") is " + fibonacci(startNum)); }}arrow_forward
- Modify this code to make a moving animated house. import java.awt.Color;import java.awt.Font;import java.awt.Graphics;import java.awt.Graphics2D; /**** @author**/public class MyHouse { public static void main(String[] args) { DrawingPanel panel = new DrawingPanel(750, 500); panel.setBackground (new Color(65,105,225)); Graphics g = panel.getGraphics(); background(g); house (g); houseRoof (g); lawnRoof (g); windows (g); windowsframes (g); chimney(g); } /** * * @param g */ static public void background(Graphics g) { g.setColor (new Color (225,225,225));//clouds g.fillOval (14,37,170,55); g.fillOval (21,21,160,50); g.fillOval (351,51,170,55); g.fillOval (356,36,160,50); } static public void house (Graphics g){g.setColor (new Color(139,69,19)); //houseg.fillRect (100,250,400,200);g.fillRect (499,320,200,130);g.setColor(new Color(190,190,190)); //chimney and doorsg.fillRect (160,150,60,90);g.fillRect…arrow_forwardHow do I fix the result? import java.lang.*;import java.util.Scanner; // import the Scanner class public class Main { public static Scanner keyboard = new Scanner(System.in); public static void main(String[] args) { boolean doItAgain = false;do{doItAgain = false;calcType();System.out.println("Do you want to start over? (Y/N)");//Scanner keyboard = new Scanner(System.in);String decision = keyboard.next();if(decision.equals("Y")) {doItAgain = true;}}while(doItAgain);System.out.println("Goodbye");} public static void calcType() {String mode;System.out.println("Enter the calculator mode: Standard/Scientific?");//Scanner keyboard = new Scanner(System.in);mode = keyboard.next();if(mode.equals("Standard")) {standard();}else if (mode.equals("Scientific")) {scientific();}elseSystem.out.println("Unknown calculator type: " + mode);} public static void standard() {System.out.println("The calculator will operate in standard mode.");boolean doItAgain;do{doItAgain = false;System.out.println("Enter…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
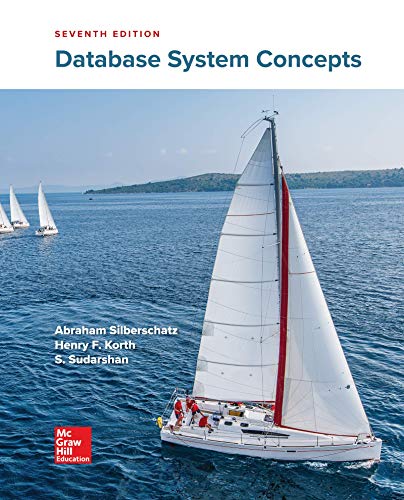
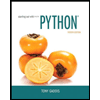
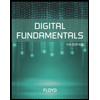
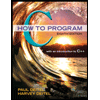
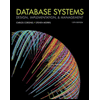
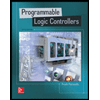