Continuing from the example, modify the program so that it outputs the average grade in each course, and also shows which course has the highest average grade. import java.util.Scanner; public class Main { publicstaticvoid main(String[] args) { Scanner s = new Scanner(System.in); int numCourses, numTests; double[][] testScores; System.out.print("How many courses are you taking? "); numCourses = s.nextInt(); testScores = new double[numCourses][]; // allocate an array of references to 1-D arrays (rows) for(int i = 0; i < numCourses; i++) { System.out.print("How many tests are in course number " + (i+1) + "? "); numTests = s.nextInt(); // allocate this row: a 1-D array of doubles: testScores[i] = new double[numTests]; } for(int courseId=0; courseId < testScores.length; courseId++) { for(int testId=0; testId < testScores[courseId].length; testId++) { System.out.print("What was your grade on test " + (testId+1) + " for course " + (courseId+1) + "? "); testScores[courseId][testId] = s.nextDouble(); } System.out.println(); // blank line to separate courses } } } /* Sample Output: How many courses are you taking? 5 How many tests are in course number 1? 4 How many tests are in course number 2? 2 How many tests are in course number 3? 1 How many tests are in course number 4? 3 How many tests are in course number 5? 2 What was your grade on test 1 for course 1? 80 What was your grade on test 2 for course 1? 88 What was your grade on test 3 for course 1? 92 What was your grade on test 4 for course 1? 79 What was your grade on test 1 for course 2? 78 What was your grade on test 2 for course 2? 77 What was your grade on test 1 for course 3? 81 What was your grade on test 1 for course 4? 69 What was your grade on test 2 for course 4? 75 What was your grade on test 3 for course 4? 79 What was your grade on test 1 for course 5? 90 What was your grade on test 2 for course 5? 95 */
Continuing from the example, modify the program so that it outputs the average grade in each course, and also shows which course has the highest average grade. import java.util.Scanner; public class Main { publicstaticvoid main(String[] args) { Scanner s = new Scanner(System.in); int numCourses, numTests; double[][] testScores; System.out.print("How many courses are you taking? "); numCourses = s.nextInt(); testScores = new double[numCourses][]; // allocate an array of references to 1-D arrays (rows) for(int i = 0; i < numCourses; i++) { System.out.print("How many tests are in course number " + (i+1) + "? "); numTests = s.nextInt(); // allocate this row: a 1-D array of doubles: testScores[i] = new double[numTests]; } for(int courseId=0; courseId < testScores.length; courseId++) { for(int testId=0; testId < testScores[courseId].length; testId++) { System.out.print("What was your grade on test " + (testId+1) + " for course " + (courseId+1) + "? "); testScores[courseId][testId] = s.nextDouble(); } System.out.println(); // blank line to separate courses } } } /* Sample Output: How many courses are you taking? 5 How many tests are in course number 1? 4 How many tests are in course number 2? 2 How many tests are in course number 3? 1 How many tests are in course number 4? 3 How many tests are in course number 5? 2 What was your grade on test 1 for course 1? 80 What was your grade on test 2 for course 1? 88 What was your grade on test 3 for course 1? 92 What was your grade on test 4 for course 1? 79 What was your grade on test 1 for course 2? 78 What was your grade on test 2 for course 2? 77 What was your grade on test 1 for course 3? 81 What was your grade on test 1 for course 4? 69 What was your grade on test 2 for course 4? 75 What was your grade on test 3 for course 4? 79 What was your grade on test 1 for course 5? 90 What was your grade on test 2 for course 5? 95 */
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
100%
Continuing from the example, modify the program so that it outputs the average grade in each course, and also shows which course has the highest average grade.
import java.util.Scanner;
public class Main
{
publicstaticvoid main(String[] args)
{
Scanner s = new Scanner(System.in);
int numCourses, numTests;
double[][] testScores;
System.out.print("How many courses are you taking? ");
numCourses = s.nextInt();
testScores = new double[numCourses][];
// allocate an array of references to 1-D arrays (rows)
for(int i = 0; i < numCourses; i++)
{
System.out.print("How many tests are in course number "
+ (i+1) + "? ");
numTests = s.nextInt();
// allocate this row: a 1-D array of doubles:
testScores[i] = new double[numTests];
}
for(int courseId=0; courseId < testScores.length; courseId++)
{
for(int testId=0; testId < testScores[courseId].length; testId++)
{
System.out.print("What was your grade on test "
+ (testId+1) + " for course "
+ (courseId+1) + "? ");
testScores[courseId][testId] = s.nextDouble();
}
System.out.println(); // blank line to separate courses
}
}
}
/* Sample Output:
How many courses are you taking? 5
How many tests are in course number 1? 4
How many tests are in course number 2? 2
How many tests are in course number 3? 1
How many tests are in course number 4? 3
How many tests are in course number 5? 2
What was your grade on test 1 for course 1? 80
What was your grade on test 2 for course 1? 88
What was your grade on test 3 for course 1? 92
What was your grade on test 4 for course 1? 79
What was your grade on test 1 for course 2? 78
What was your grade on test 2 for course 2? 77
What was your grade on test 1 for course 3? 81
What was your grade on test 1 for course 4? 69
What was your grade on test 2 for course 4? 75
What was your grade on test 3 for course 4? 79
What was your grade on test 1 for course 5? 90
What was your grade on test 2 for course 5? 95
*/
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 1 images

Recommended textbooks for you
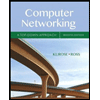
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
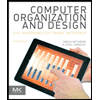
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
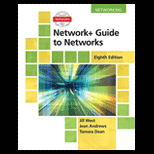
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
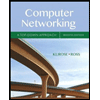
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
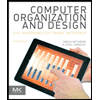
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
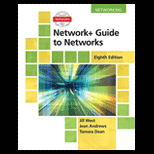
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
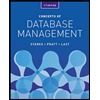
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
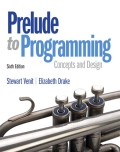
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
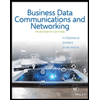
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY