JAVA Program ASAP Here is a Sort.java program. Create A FileSorting.java program so it runs and passes the test cases in Hypergrade. import java.util.ArrayList; public class Sort { public static > void insertionSort(E[] array) { for (int i = 1; i < array.length; i++) { for (int j = i; j > 0 && array[j].compareTo(array[j - 1]) < 0; j--) { swap(array, j, j - 1); } } } public static > void insertionSort(ArrayList arrayList) { for (int i = 1; i < arrayList.size(); i++) { for (int j = i; j > 0 && arrayList.get(j).compareTo(arrayList.get(j - 1)) < 0; j--) { swap(arrayList, j, j - 1); } } } public static > void bubbleSort(E[] array) { for (int i = 0; i < array.length - 1; i++) { for (int j = array.length - 1; j > i; j--) { if (array[j].compareTo(array[j - 1]) < 0) { swap(array, j, j - 1); } } } } public static > void bubbleSort(ArrayList arrayList) { for (int i = 0; i < arrayList.size() - 1; i++) { for (int j = arrayList.size() - 1; j > i; j--) { if (arrayList.get(j).compareTo(arrayList.get(j - 1)) < 0) { swap(arrayList, j, j - 1); } } } } public static > void selectionSort(E[] array) { for (int i = 0; i < array.length - 1; i++) { int lowindex = i; for (int j = array.length - 1; j > i; j--) if (array[j].compareTo(array[lowindex]) < 0) lowindex = j; swap(array, i, lowindex); } } public static > void selectionSort(ArrayList arrayList) { for (int i = 0; i < arrayList.size() - 1; i++) { int lowindex = i; for (int j = arrayList.size() - 1; j > i; j--) if (arrayList.get(j).compareTo(arrayList.get(lowindex)) < 0) lowindex = j; swap(arrayList, i, lowindex); } } private static void swap(E[] array, int p1, int p2) { E temp = array[p1]; array[p1] = array[p2]; array[p2] = temp; } private static void swap(ArrayList arrayList, int p1, int p2) { E temp = arrayList.get(p1); arrayList.set(p1, arrayList.get(p2)); arrayList.set(p2, temp); } } input2x2.csv -67,-11 -27,-70 input1.csv 10 input10x10.csv 56,-19,-21,-51,45,96,-46 -27,29,-58,85,8,71,34 50,51,40,50,100,-82,-87 -47,-24,-27,-32,-25,46,88 -47,95,-41,-75,85,-16,43 -78,0,94,-77,-69,78,-25 -80,-31,-95,82,-86,-32,-22 68,-52,-4,-68,10,-14,-89 26,33,-59,-51,-48,-34,-52 -47,-24,80,16,80,-66,-42 input0.csv Test Case 1 Please enter the file name or type QUIT to exit:\n input1.csvENTER 10\n Test Case 2 Please enter the file name or type QUIT to exit:\n input2x2.csvENTER -67,-11\n -70,-27\n Test Case 3 Please enter the file name or type QUIT to exit:\n input10x10.csvENTER -51,-46,-21,-19,45,56,96\n -58,-27,8,29,34,71,85\n -87,-82,40,50,50,51,100\n -47,-32,-27,-25,-24,46,88\n -75,-47,-41,-16,43,85,95\n -78,-77,-69,-25,0,78,94\n -95,-86,-80,-32,-31,-22,82\n -89,-68,-52,-14,-4,10,68\n -59,-52,-51,-48,-34,26,33\n -66,-47,-42,-24,16,80,80\n Test Case 4 Please enter the file name or type QUIT to exit:\n input0.csvENTER File input0.csv is empty.\n Test Case 5 Please enter the file name or type QUIT to exit:\n input2.csvENTER File input2.csv is not found.\n Please re-enter the file name or type QUIT to exit:\n input1.csvENTER 10\n Test Case 6 Please enter the file name or type QUIT to exit:\n quitENTER Test Case 7 Please enter the file name or type QUIT to exit:\n input2.csvENTER File input2.csv is not found.\n Please re-enter the file name or type QUIT to exit:\n QUITENTER
JAVA Program ASAP
Here is a Sort.java program. Create A FileSorting.java program so it runs and passes the test cases in Hypergrade.
import java.util.ArrayList;
public class Sort {
public static <E extends Comparable<? super E>> void insertionSort(E[] array) {
for (int i = 1; i < array.length; i++) {
for (int j = i; j > 0 && array[j].compareTo(array[j - 1]) < 0; j--) {
swap(array, j, j - 1);
}
}
}
public static <E extends Comparable<? super E>> void insertionSort(ArrayList<E> arrayList) {
for (int i = 1; i < arrayList.size(); i++) {
for (int j = i; j > 0 && arrayList.get(j).compareTo(arrayList.get(j - 1)) < 0; j--) {
swap(arrayList, j, j - 1);
}
}
}
public static <E extends Comparable<? super E>> void bubbleSort(E[] array) {
for (int i = 0; i < array.length - 1; i++) {
for (int j = array.length - 1; j > i; j--) {
if (array[j].compareTo(array[j - 1]) < 0) {
swap(array, j, j - 1);
}
}
}
}
public static <E extends Comparable<? super E>> void bubbleSort(ArrayList<E> arrayList) {
for (int i = 0; i < arrayList.size() - 1; i++) {
for (int j = arrayList.size() - 1; j > i; j--) {
if (arrayList.get(j).compareTo(arrayList.get(j - 1)) < 0) {
swap(arrayList, j, j - 1);
}
}
}
}
public static <E extends Comparable<? super E>> void selectionSort(E[] array) {
for (int i = 0; i < array.length - 1; i++) {
int lowindex = i;
for (int j = array.length - 1; j > i; j--)
if (array[j].compareTo(array[lowindex]) < 0)
lowindex = j;
swap(array, i, lowindex);
}
}
public static <E extends Comparable<? super E>> void selectionSort(ArrayList<E> arrayList) {
for (int i = 0; i < arrayList.size() - 1; i++) {
int lowindex = i;
for (int j = arrayList.size() - 1; j > i; j--)
if (arrayList.get(j).compareTo(arrayList.get(lowindex)) < 0)
lowindex = j;
swap(arrayList, i, lowindex);
}
}
private static <E> void swap(E[] array, int p1, int p2) {
E temp = array[p1];
array[p1] = array[p2];
array[p2] = temp;
}
private static <E> void swap(ArrayList<E> arrayList, int p1, int p2) {
E temp = arrayList.get(p1);
arrayList.set(p1, arrayList.get(p2));
arrayList.set(p2, temp);
}
}
input2x2.csv
-67,-11
-27,-70
input1.csv
10
input10x10.csv
56,-19,-21,-51,45,96,-46
-27,29,-58,85,8,71,34
50,51,40,50,100,-82,-87
-47,-24,-27,-32,-25,46,88
-47,95,-41,-75,85,-16,43
-78,0,94,-77,-69,78,-25
-80,-31,-95,82,-86,-32,-22
68,-52,-4,-68,10,-14,-89
26,33,-59,-51,-48,-34,-52
-47,-24,80,16,80,-66,-42
input0.csv
Test Case 1
input1.csvENTER
10\n
Test Case 2
input2x2.csvENTER
-67,-11\n
-70,-27\n
Test Case 3
input10x10.csvENTER
-51,-46,-21,-19,45,56,96\n
-58,-27,8,29,34,71,85\n
-87,-82,40,50,50,51,100\n
-47,-32,-27,-25,-24,46,88\n
-75,-47,-41,-16,43,85,95\n
-78,-77,-69,-25,0,78,94\n
-95,-86,-80,-32,-31,-22,82\n
-89,-68,-52,-14,-4,10,68\n
-59,-52,-51,-48,-34,26,33\n
-66,-47,-42,-24,16,80,80\n
Test Case 4
input0.csvENTER
File input0.csv is empty.\n
Test Case 5
input2.csvENTER
File input2.csv is not found.\n
Please re-enter the file name or type QUIT to exit:\n
input1.csvENTER
10\n
Test Case 6
quitENTER
Test Case 7
input2.csvENTER
File input2.csv is not found.\n
Please re-enter the file name or type QUIT to exit:\n
QUITENTER

Step by step
Solved in 4 steps with 3 images

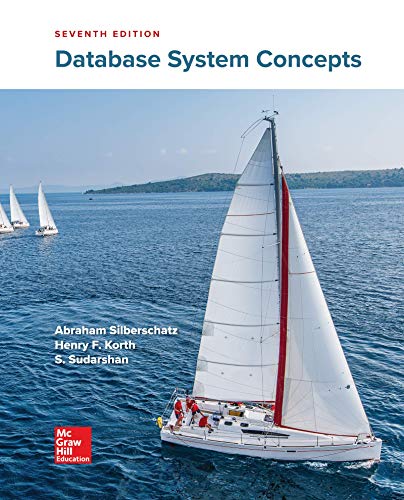
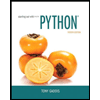
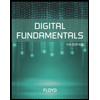
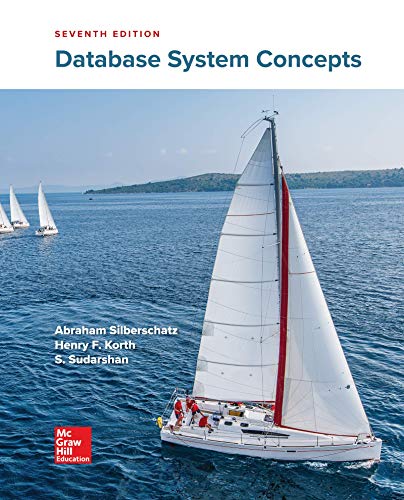
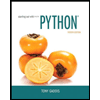
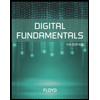
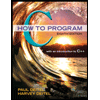
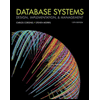
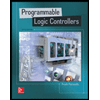