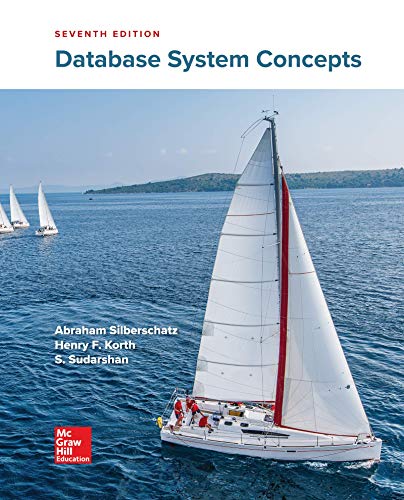
Part 1 is already done and here it is
public class Point {
protected double x;
protected double y;
public Point() {
}
public Point(double x, double y) {
this.x = x;
this.y = y;
}
public double getX() {
return x;
}
public double getY() {
return y;
}
public void setPoint(Point X){
x = X.x;
y = X.y;
}
void makeCopy(Point x){
setPoint(x);
}
Point getCopy(){
return this;
}
public void printPoint(){
System.out.println("["+this.x+", "+this.y+"]");
}
@Override
public String toString() {
return "x-Coordinate is " + x + "and y-coordinate is " + y;
}
public boolean equals(Point X) {
if (this.x != X.x)
return false;
if(this.y != X.y)
return false;
return true;
}
}
public class TestPoint {
public static void main(String[] args){
Point myPoint = new Point(5,4);
Point yourPoint = new Point(0,0);
myPoint.printPoint();
yourPoint.printPoint();
yourPoint.setPoint(new Point(5,45));
System.out.println(myPoint.equals(yourPoint));
myPoint.setPoint(new Point(6,9));
myPoint.printPoint();
yourPoint.makeCopy(myPoint);
yourPoint.printPoint();
}
NEED part 2
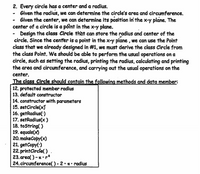
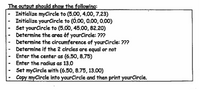

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 5 images

- public class Course { private String courseNumber, courseTitle; public Course() { } public Course(String courseNumber, String courseTitle) { this.courseNumber = courseNumber; this.courseTitle = courseTitle; } public void setCourseNumber(String courseNumber) { this.courseNumber = courseNumber; } public void setCourseTitle(String courseTitle) { this.courseTitle = courseTitle; } public String getCourseNumber() { return courseNumber; } public String getCourseTitle() { return courseTitle; } public void printInfo() { System.out.println("Course Information: "); System.out.println(" Course Number: " + courseNumber); System.out.println(" Course Title: " +…arrow_forwardConsider the following class definitions: public class Thing {public void string_method(String word) {word = word.substring(word.length() - 1);System.out.print(word);} }public class Thing2 extends Thing {public void string_method(String word) {super.string_method(word);System.out.print(word.substring(0,1));}} The following code segment appears in a class other than Thing or Thing2 Thing2 thing = new Thing2();thing.string_method("Tacky"); What is printed as a result of running the code segment? Group of answer choices yT yy Index Out of Bounds Error TT TackyTackyarrow_forwardpublic class Ex08FanTest { public static void main (String[] args){ Ex08Fan fan1= new Ex08Fan(); Ex08Fan fan2= new Ex08Fan(); fan1.setOn(true); fan1.setSpeed(3); fan1.setRadius(10); fan1.setColor("yellow"); fan2.setOn(true); fan2.setSpeed(2); fan2.setRadius(5); fan2.setColor("blue"); fan2.setOn(false); System.out.println("Fan 1:\n" + fan1.toString()); System.out.println("\nFan 2:\n" + fan2.toString()); }}arrow_forward
- Java programarrow_forwardGiven the following class definition: class Point2d { private int x, y; public Point2d () { X = 0; y = 0; } public Point2d (int i, int j) { X = i; y = j; } public void SetX (int i) { x = i; } public void SetY (int j) { y = j; } public int GetX () { return x; public int GetŸ () { return y; } public String toString() { return '[' + x + ", } + y + ']'; } } Use inheritance to create a class, Point3d, with any additional appropriate data and/or functions.arrow_forwardPLEASE ANSWER ALL QUESTIONS ON THE NEXT PAGE: class bookType { public: void setBookTitle(string s); //sets the bookTitle to s void setBookISBN(string ISBN); //sets the private member bookISBN to the parameter void setBookPrice(double cost); //sets the private member bookPrice to cost void setCopiesInStock(int noOfCopies); //sets the private member copiesInStock to noOfCopies void printInfo() const; //prints the bookTitle, bookISBN, the bookPrice and the copiesInStock string getBookISBN() const; //returns the bookISBN double getBookPrice() const; //returns the bookPrice int showQuantityInStock() const; //returns the quantity in stock void updateQuantity(int addBooks); //adds addBooks to the quantityInStock, so that the quantity in stock now has it original // value plus the parameter sent to this function private: string…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
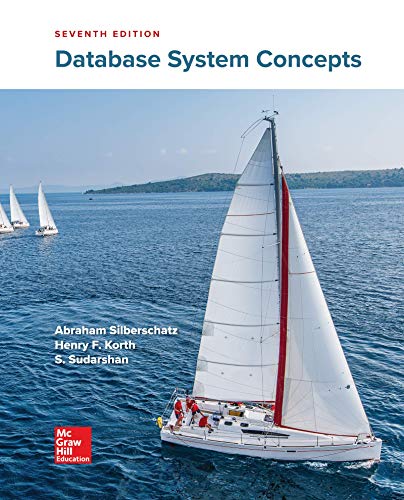
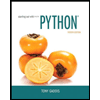
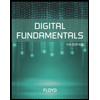
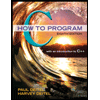
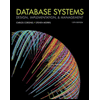
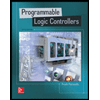