Hi, can i get assistance with this book class in Java please? The comments with the "TODO" is what I need assistance with, thank you! The author class is attached to the question in case there is any relevance for it. public class Book { private String title; private double averageRating; private String ISBN; private int numPages; // TODO: insert an appropriate collection for associating Authors and initialise within constructors public Book(){ } public Book(String title, double averageRating, String isbn, int numPages){ this.title = title; this.averageRating = averageRating; this.ISBN = isbn; this.numPages = numPages; } //accessors public String getTitle(){ return title; } public double getAverageRating(){ return averageRating; } public String getISBN(){ return ISBN; } public int getNumPages(){ return numPages; } // TODO: provide an accessor for the book collection public String getAuthorList(){ String authorList =""; // TODO: insert code to return all author names separated by commas if multiple authors return authorList; } //mutators public void setTitle(String title){ this.title = title; } public void setAverageRating(double averageRating){ this.averageRating = averageRating; } public void setISBN(String isbn){ this.ISBN = isbn; } public void setNumPages(int numPages){ this.numPages = numPages; } public void addAuthor(Author a){ // TODO: complete this method /* Inserts a new Author into the author list. No duplicates are allowed */ } public boolean isAuthoredBy(Author a){ // TODO: complete this method - /* Returns true if the Book is authored by the supplied author false otherwise */ return false; } //variety of String representations of a Book public String toStringTitleFirst(){ return getTitle() + ", " + getAuthorList() + " " + getAverageRating() + ", " + getISBN() + ", " + getNumPages() + " pages."; } public String toStringISBNFirst(){ return getISBN() + " " + getTitle() + ", " + getAuthorList() + " " + getAverageRating() + ", " + ", " + getNumPages() + " pages."; } public String toStringRatingFirst(){ return getAverageRating() + " " + getTitle() + ", " + getAuthorList() + " " + getISBN() + ", " + ", " + getNumPages() + " pages."; } public String toStringPagesFirst(){ return getNumPages() + " pages " + getTitle() + ", " + getAuthorList() + " " + getISBN() + ", " + ", " + getAverageRating() ; } }
Hi, can i get assistance with this book class in Java please? The comments with the "TODO" is what I need assistance with, thank you! The author class is attached to the question in case there is any relevance for it.
public class Book {
private String title;
private double averageRating;
private String ISBN;
private int numPages;
// TODO: insert an appropriate collection for associating Authors and initialise within constructors
public Book(){
}
public Book(String title, double averageRating, String isbn, int numPages){
this.title = title;
this.averageRating = averageRating;
this.ISBN = isbn;
this.numPages = numPages;
}
//accessors
public String getTitle(){
return title;
}
public double getAverageRating(){
return averageRating;
}
public String getISBN(){
return ISBN;
}
public int getNumPages(){
return numPages;
}
// TODO: provide an accessor for the book collection
public String getAuthorList(){
String authorList ="";
// TODO: insert code to return all author names separated by commas if multiple authors
return authorList;
}
//mutators
public void setTitle(String title){
this.title = title;
}
public void setAverageRating(double averageRating){
this.averageRating = averageRating;
}
public void setISBN(String isbn){
this.ISBN = isbn;
}
public void setNumPages(int numPages){
this.numPages = numPages;
}
public void addAuthor(Author a){
// TODO: complete this method
/* Inserts a new Author into the author list. No duplicates are allowed */
}
public boolean isAuthoredBy(Author a){
// TODO: complete this method -
/* Returns true if the Book is authored by the supplied author
false otherwise */
return false;
}
//variety of String representations of a Book
public String toStringTitleFirst(){
return getTitle() + ", " + getAuthorList() + " " + getAverageRating() + ", " + getISBN() + ", " + getNumPages() + " pages.";
}
public String toStringISBNFirst(){
return getISBN() + " " + getTitle() + ", " + getAuthorList() + " " + getAverageRating() + ", " + ", " + getNumPages() + " pages.";
}
public String toStringRatingFirst(){
return getAverageRating() + " " + getTitle() + ", " + getAuthorList() + " " + getISBN() + ", " + ", " + getNumPages() + " pages.";
}
public String toStringPagesFirst(){
return getNumPages() + " pages " + getTitle() + ", " + getAuthorList() + " " + getISBN() + ", " + ", " + getAverageRating() ;
}
}


Trending now
This is a popular solution!
Step by step
Solved in 2 steps

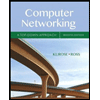
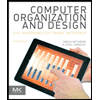
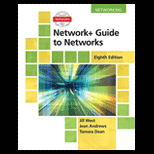
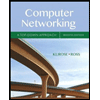
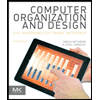
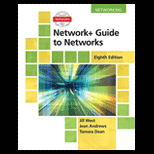
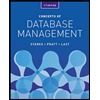
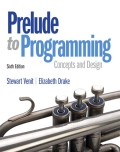
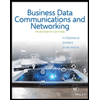