in java Re-implement the Rational class : class Rational { private int numerator; private int denominator; public Rational(int num, int den) { numerator = num; denominator = den; } public Rational add(Rational o) { int NumResult = numerator * o.denominator + denominator * o.numerator; int DenResult = denominator * o.denominator; return simplify(NumResult, DenResult); } public Rational sub(Rational o) { int NumResult = numerator * o.denominator - denominator * o.numerator; int DenResult = denominator * o.denominator; return simplify(NumResult, DenResult); } public Rational mul(Rational o) { int NumResult = numerator * o.numerator; int DenResult = denominator * o.denominator; return simplify(NumResult, DenResult); } public Rational div(Rational o) { int NumResult = numerator * o.denominator; int DenResult = denominator * o.numerator; return simplify(NumResult, DenResult); } private int gcd(int a, int b) { if (b == 0) return a; return gcd(b, a % b); } private Rational simplify(int num, int den) { int gcdValue = gcd(num, den); num /= gcdValue; den /= gcdValue; return new Rational(num, den); } public String toString() { return "(" + numerator + " / " + denominator + ")"; } } class RationalDriver { public static void main(String[] args) { Rational a = new Rational(6, 4); Rational b = new Rational(4, 6); System.out.println(a + " + " + b + " = " + a.add(b)); System.out.println(a + " - " + b + " = " + a.sub(b)); System.out.println(a + " * " + b + " = " + a.mul(b)); System.out.println(a + " / " + b + " = " + a.div(b)); } } Extend the Number class and implement the Comparable interface to introduce new functionality to your Rational class. The class definition should look like this: public class Rational extends Number implements Comparable { // code goes here } In addition a new private method gcd should be defined to ensure that the number is always represented in the lowest terms. Here is the Main Download Main class that I will use to test your Rational classes. Remember to only submit the Rational.java file! public class Main { public static void main(String[] args) { Rational a = new Rational(2, 4); Rational b = new Rational(2, 6); System.out.println(a + " + " + b + " = " + a.add(b)); System.out.println(a + " - " + b + " = " + a.sub(b)); System.out.println(a + " * " + b + " = " + a.mul(b)); System.out.println(a + " / " + b + " = " + a.div(b)); Rational[] arr = {new Rational(7, 1), new Rational(6, 1), new Rational(5, 1), new Rational(4, 1), new Rational(3, 1), new Rational(2, 1), new Rational(1, 1), new Rational(1, 2), new Rational(1, 3), new Rational(1, 4), new Rational(1, 5), new Rational(1, 6), new Rational(1, 7), new Rational(1, 8), new Rational(1, 9), new Rational(0, 1)}; selectSort(arr); for (Rational r : arr) { System.out.println(r); } Number n = new Rational(3, 2); System.out.println(n.doubleValue()); System.out.println(n.floatValue()); System.out.println(n.intValue()); System.out.println(n.longValue()); } public static > void selectSort(T[] array) { T temp; int mini; for (int i = 0; i < array.length - 1; ++i) { mini = i; for (int j = i + 1; j < array.length; ++j) { if (array[j].compareTo(array[mini]) < 0) { mini = j; } } if (i != mini) { temp = array[i]; array[i] = array[mini]; array[mini] = temp; } } }
in java
Re-implement the Rational class :
class Rational {
private int numerator;
private int denominator;
public Rational(int num, int den) {
numerator = num;
denominator = den;
}
public Rational add(Rational o) {
int NumResult = numerator * o.denominator + denominator * o.numerator;
int DenResult = denominator * o.denominator;
return simplify(NumResult, DenResult);
}
public Rational sub(Rational o) {
int NumResult = numerator * o.denominator - denominator * o.numerator;
int DenResult = denominator * o.denominator;
return simplify(NumResult, DenResult);
}
public Rational mul(Rational o) {
int NumResult = numerator * o.numerator;
int DenResult = denominator * o.denominator;
return simplify(NumResult, DenResult);
}
public Rational div(Rational o) {
int NumResult = numerator * o.denominator;
int DenResult = denominator * o.numerator;
return simplify(NumResult, DenResult);
}
private int gcd(int a, int b) {
if (b == 0)
return a;
return gcd(b, a % b);
}
private Rational simplify(int num, int den) {
int gcdValue = gcd(num, den);
num /= gcdValue;
den /= gcdValue;
return new Rational(num, den);
}
public String toString() {
return "(" + numerator + " / " + denominator + ")";
}
}
class RationalDriver {
public static void main(String[] args) {
Rational a = new Rational(6, 4);
Rational b = new Rational(4, 6);
System.out.println(a + " + " + b + " = " + a.add(b));
System.out.println(a + " - " + b + " = " + a.sub(b));
System.out.println(a + " * " + b + " = " + a.mul(b));
System.out.println(a + " / " + b + " = " + a.div(b));
}
}
Extend the Number class and implement the Comparable interface to
introduce new functionality to your Rational class. The class
definition should look like this:
public class Rational extends Number implements Comparable<Rational> { // code goes here }
In addition a new private method gcd should be
defined to ensure that the number is always represented in
the lowest terms.
Here is the Main Download Main class that I will use to test your Rational classes. Remember to only submit the Rational.java file!
public class Main {
public static void main(String[] args) {
Rational a = new Rational(2, 4);
Rational b = new Rational(2, 6);
System.out.println(a + " + " + b + " = " + a.add(b));
System.out.println(a + " - " + b + " = " + a.sub(b));
System.out.println(a + " * " + b + " = " + a.mul(b));
System.out.println(a + " / " + b + " = " + a.div(b));
Rational[] arr = {new Rational(7, 1), new Rational(6, 1),
new Rational(5, 1), new Rational(4, 1),
new Rational(3, 1), new Rational(2, 1),
new Rational(1, 1), new Rational(1, 2),
new Rational(1, 3), new Rational(1, 4),
new Rational(1, 5), new Rational(1, 6),
new Rational(1, 7), new Rational(1, 8),
new Rational(1, 9), new Rational(0, 1)};
selectSort(arr);
for (Rational r : arr) {
System.out.println(r);
}
Number n = new Rational(3, 2);
System.out.println(n.doubleValue());
System.out.println(n.floatValue());
System.out.println(n.intValue());
System.out.println(n.longValue());
}
public static <T extends Comparable<? super T>> void selectSort(T[]
array) {
T temp;
int mini;
for (int i = 0; i < array.length - 1; ++i) {
mini = i;
for (int j = i + 1; j < array.length; ++j) {
if (array[j].compareTo(array[mini]) < 0) {
mini = j;
}
}
if (i != mini) {
temp = array[i];
array[i] = array[mini];
array[mini] = temp;
}
}
}

Step by step
Solved in 3 steps

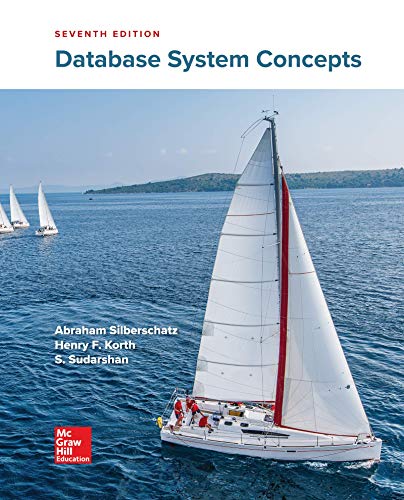
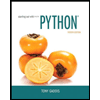
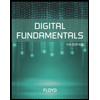
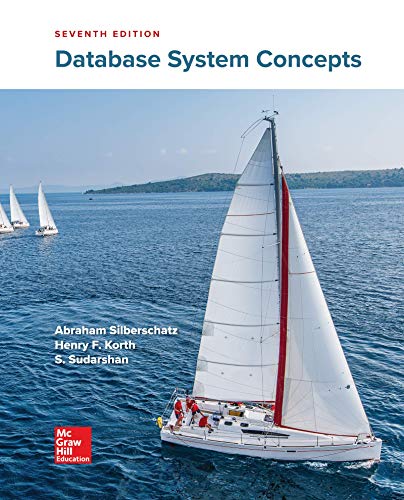
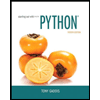
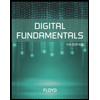
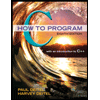
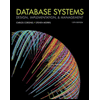
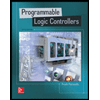