Write a Java program that prompts a user for vehicle data and stores it in a linked list, and then sorts the list in ascending order based on miles-per-gallon and writes the sorted data to a text file, you can follow these steps: Create a class named Vehicle with private fields: make (String), model (String), and milesPerGallon (double). Include getters and setters for these fields. Implement the Comparable interface for Vehicle class and override the compareTo() method to compare vehicles based on their milesPerGallon. Create a main class (for example, VehicleDriver.java) to handle user input and perform the necessary operations. Inside the main method, create a BufferedReader object for user input. Prompt the user to enter the number of vehicle data they want to enter and store it in a variable (for example, nVehicles). Use a loop to iterate nVehicles times and prompt the user to enter make, model, and miles per gallon for each vehicle. Create Vehicle objects using the input data and add them to a LinkedList. Sort the LinkedList in ascending order based on miles-per-gallon using the Collections.sort() method. Prompt the user to enter a file name to write the sorted data to. Create a FileWriter object and iterate over the LinkedList, writing each vehicle's information to the file. Close the writer and display a success message. Please and thank you! Also, if it is not to much can you demonstrate/show a screenshot that the file can be seen/viewed in Notepad.
Write a Java program that prompts a user for vehicle data and stores it in a linked list, and then sorts the list in ascending order based on miles-per-gallon and writes the sorted data to a text file, you can follow these steps: Create a class named Vehicle with private fields: make (String), model (String), and milesPerGallon (double). Include getters and setters for these fields. Implement the Comparable interface for Vehicle class and override the compareTo() method to compare vehicles based on their milesPerGallon. Create a main class (for example, VehicleDriver.java) to handle user input and perform the necessary operations. Inside the main method, create a BufferedReader object for user input. Prompt the user to enter the number of vehicle data they want to enter and store it in a variable (for example, nVehicles). Use a loop to iterate nVehicles times and prompt the user to enter make, model, and miles per gallon for each vehicle. Create Vehicle objects using the input data and add them to a LinkedList. Sort the LinkedList in ascending order based on miles-per-gallon using the Collections.sort() method. Prompt the user to enter a file name to write the sorted data to. Create a FileWriter object and iterate over the LinkedList, writing each vehicle's information to the file. Close the writer and display a success message. Please and thank you! Also, if it is not to much can you demonstrate/show a screenshot that the file can be seen/viewed in Notepad.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
100%
Write a Java program that prompts a user for vehicle data and stores it in a linked list, and then sorts the list in ascending order based on miles-per-gallon and writes the sorted data to a text file, you can follow these steps:
- Create a class named Vehicle with private fields: make (String), model (String), and milesPerGallon (double). Include getters and setters for these fields.
- Implement the Comparable interface for Vehicle class and override the compareTo() method to compare vehicles based on their milesPerGallon.
- Create a main class (for example, VehicleDriver.java) to handle user input and perform the necessary operations.
- Inside the main method, create a BufferedReader object for user input.
- Prompt the user to enter the number of vehicle data they want to enter and store it in a variable (for example, nVehicles).
- Use a loop to iterate nVehicles times and prompt the user to enter make, model, and miles per gallon for each vehicle.
- Create Vehicle objects using the input data and add them to a LinkedList.
- Sort the LinkedList in ascending order based on miles-per-gallon using the Collections.sort() method.
- Prompt the user to enter a file name to write the sorted data to.
- Create a FileWriter object and iterate over the LinkedList, writing each vehicle's information to the file.
- Close the writer and display a success message.
Please and thank you! Also, if it is not to much can you demonstrate/show a screenshot that the file can be seen/viewed in Notepad.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 4 images

Follow-up Questions
Read through expert solutions to related follow-up questions below.
Follow-up Question
Is there a way to implement a simple graphical user interface that will allow the user to manually input a vehicle's make, model, and mpg and also be able to write the data to a text file of the user's choosing with a display message of success or failure?
Solution
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
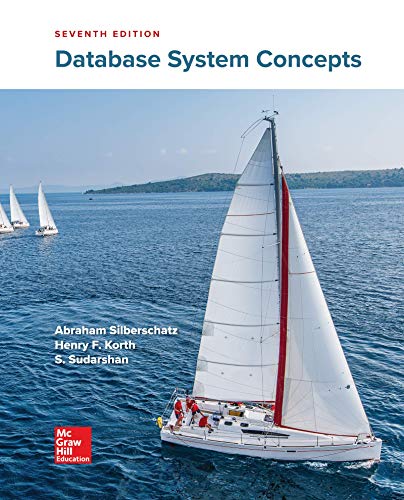
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
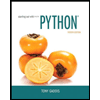
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
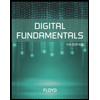
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
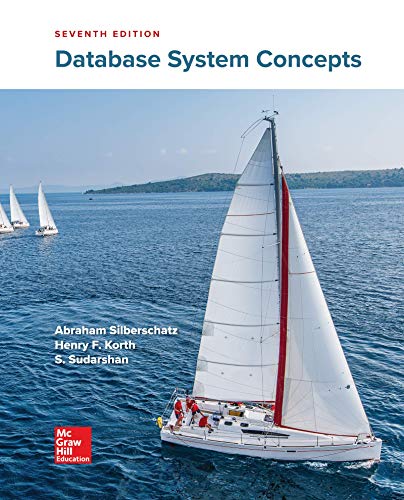
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
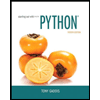
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
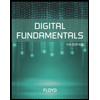
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
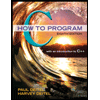
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
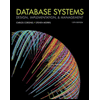
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
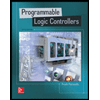
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education