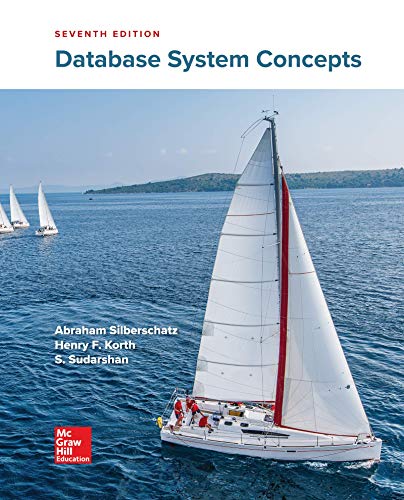
Concept explainers
USING THE FOLLOWING METHOD SWAP CODE:
import java.util.*;
class ListIndexOutOfBoundsException extends IndexOutOfBoundsException {
public ListIndexOutOfBoundsException(String s) {
super(s);
}
}
class ListUtil {
public static int maxValue(List<Integer> aList) {
if(aList == null || aList.size() == 0) {
throw new IllegalArgumentException("List cannot be null or empty");
}
int max = aList.get(0);
for(int i = 1; i < aList.size(); i++) {
if(aList.get(i) > max) {
max = aList.get(i);
}
}
return max;
}
public static void swap(List<Integer> aList, int i, int j) throws ListIndexOutOfBoundsException {
if(aList == null) {
throw new IllegalArgumentException("List cannot be null");
}
if(i < 0 || i >= aList.size() || j < 0 || j >= aList.size()) {
throw new ListIndexOutOfBoundsException("Index out of bounds. Index must be between 0 and " + (aList.size() - 1));
}
int temp = aList.get(i);
aList.set(i, aList.get(j));
aList.set(j, temp);
}
}
public class Main {
public static void main(String[] args) {
List<Integer> aList = new ArrayList<>();
aList.add(4);
aList.add(2);
aList.add(9);
aList.add(6);
System.out.println("Before Swapping: " + aList);
System.out.println("Max Value: " + ListUtil.maxValue(aList));
try {
ListUtil.swap(aList, 0, 2);
} catch (ListIndexOutOfBoundsException e) {
System.out.println(e.getMessage());
}
System.out.println("After Swapping: " + aList);
}
}
Use this method swap to write a method that reverses the order of the items in aList.

Step by stepSolved in 3 steps with 1 images

- 44 // create a loop that reads in the next int from the scanner to the intList 45 46 47 // call the ReverseArray function 48 49 50 System.out.print("Your output is: "); 51 // print the output from ReverseArray 52 ww 53 54 55 }arrow_forwardpackage edu.umsl.iterator;import java.util.ArrayList;import java.util.Arrays;import java.util.Collection;import java.util.Iterator;public class Main {public static void main(String[] args) {String[] cities = {"New York", "Atlanta", "Dallas", "Madison"};Collection<String> stringCollection = new ArrayList<>(Arrays.asList(cities));Iterator<String> iterator = stringCollection.iterator();while (iterator.hasNext()) {System.out.println(/* Fill in here */);}}} Rewrite the while loop to print out the collection using an iterator. Group of answer choices iterator.toString() iterator.getClass(java.lang.String) iterator.remove() iterator.next()arrow_forwardimport java.util.HashSet; import java.util.Set; // Define a class named LinearSearchSet public class LinearSearchSet { // Define a method named linearSearch that takes in a Set and an integer target // as parameters public static boolean linearSearch(Set<Integer> set, int target) { // Iterate over all elements in the Set for () { // Check if the current value is equal to the target if () { // If so, return true } } // If the target was not found, return false } // Define the main method public static void main(String[] args) { // Create a HashSet of integers and populate integer values Set<Integer> numbers = new HashSet<>(); // Define the target to search for numbers.add(3); numbers.add(6); numbers.add(2); numbers.add(9); numbers.add(11); // Call the linearSearch method with the set…arrow_forward
- The following code prints out all permutations of the string generate. Insert the missing statement. public class PermutationGeneratorTester { public static void main(String[] args) { PermutationGenerator generator = new PermutationGenerator("generate"); ArrayList<String> permutations = generator.getPermutations(); for (String s : permutations) { ____________________ } } }arrow_forwardstarter code //Provided imports, feel free to use these if neededimport java.util.Collections;import java.util.ArrayList; /** * TODO: add class header */public class Sorts { /** * this helper finds the appropriate number of buckets you should allocate * based on the range of the values in the input list * @param list the input list to bucket sort * @return number of buckets */ private static int assignNumBuckets(ArrayList<Integer> list) { Integer max = Collections.max(list); Integer min = Collections.min(list); return (int) Math.sqrt(max - min) + 1; } /** * this helper finds the appropriate bucket index that a data should be * placed in * @param data a particular data from the input list if you are using * loop iteration * @param numBuckets number of buckets * @param listMin the smallest element of the input list * @return the index of the bucket for which the particular data should…arrow_forwardimport java.util.*; public class Main{ public static void main(String[] args) { Main m = new Main(); m.go(); } private void go() { List<Stadium> parks = new ArrayList<Stadium>(); parks.add(new Stadium("PNC Park", "Pittsburgh", 38362, true)); parks.add(new Stadium("Dodgers Stadium", "Los Angeles", 56000, true)); parks.add(new Stadium("Citizens Bank Park", "Philadelphia", 43035, false)); parks.add(new Stadium("Coors Field", "Denver", 50398, true)); parks.add(new Stadium("Yankee Stadium", "New York", 54251, false)); parks.add(new Stadium("AT&T Park", "San Francisco", 41915, true)); parks.add(new Stadium("Citi Field", "New York", 41922, false)); parks.add(new Stadium("Angels Stadium", "Los Angeles", 45050, true)); Collections.sort(parks, Stadium.ByKidZoneCityName.getInstance()); for (Stadium s : parks) System.out.println(s); }}…arrow_forward
- JAVA plese Implement the indexOf method in the LinkedIntegerList class public int indexOf(int value); /** * Returns whether the given value exists in the list. * @param value - value to be searched. * @return true if specified value is present in the list, false otherwise. */ } public static void main(String[] args) { // TODO Auto-generated method stub SimpleIntegerListADT myList = null; System.out.println(myList); for(int i=2; i<8; i+=2) { myList.add(i); } System.out.println(myList.indexOf(44));arrow_forwardThis is using Data Structures in Javaarrow_forwardWrite all the code within the main method in the Test Truck class below. Implement the following functionality. a) Constructs two truck objects: one with any make and model you choose and the second object with gas tank capacity 10. b) If an exception occurs, print the stack trace. c) Prints both truck objects that were constructed. import java.lang.IllegalArgumentException ; public class TestTruck { public static void main ( String [] args ) { heres the truck class information A Truck can be described as having a make (string), model (string), gas tank capacity (double), and whether it has a manual transmission (or not). Include the following methods in your class definition. . An overloaded constructor which takes the make and model. This method throws an IllegalArgumentException if the make is "Jeep". An overloaded constructor which takes the gas tank capacity. This method throws an IllegalArgumentException if the capacity of the gas…arrow_forward
- PROBLEM STATEMENT: Return a sublist of a given list from the range of the two integerinputs from, to. public class FindSublistFromRange{public static ArrayList<Integer> solution(ArrayList<Integer> elms, int from, int to){// ↓↓↓↓ your code goes here ↓↓↓↓return new ArrayList<>();} Can you help me with this question The language is Java Please use the code i provided above to answer this questionarrow_forwardCan you please answer this fast and with correct answer. Thankyouarrow_forwardimport java.util.Scanner; public class Playlist { // TODO: Write method to ouptut list of songs public static void main (String[] args) { Scanner scnr = new Scanner(System.in); SongNode headNode; SongNode currNode; SongNode lastNode; String songTitle; int songLength; String songArtist; // Front of nodes list headNode = new SongNode(); lastNode = headNode; // Read user input until -1 entered songTitle = scnr.nextLine(); while (!songTitle.equals("-1")) { songLength = scnr.nextInt(); scnr.nextLine(); songArtist = scnr.nextLine(); currNode = new SongNode(songTitle, songLength, songArtist); lastNode.insertAfter(currNode); lastNode = currNode; songTitle = scnr.nextLine(); } // Print linked list…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
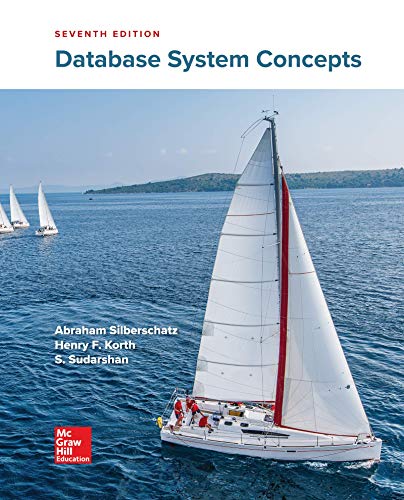
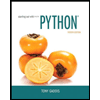
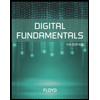
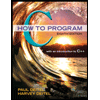
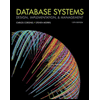
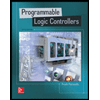