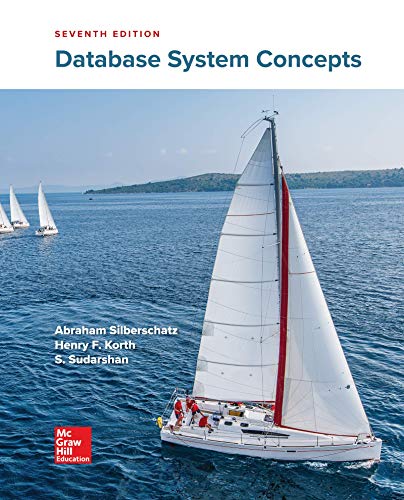
Java coding question below:
*****************************************************
(determining Big O) write a PerformanceTest class and compare the performance of mergesort and bubblesort. Use the following “PerfomanceTest” class example. Instead of the provided simpleLoop,
method, use the mentioned sorting algorithms.
A) Test with an unsorted array (call the random(n) method to create a random array)
B) Test with a sorted array (call the sorted(n) method to create a sorted array)
Example: Consider the time complexity for the following simple loop:
for(int i= 1; i <= n; i++)
k = k+5;
The complexity for this loop is O(n). To see how this
public class PerfomanceTest{
public static void main(String[] args) {
// getTime(100000);
getTime(1000000);
}
public static void getTime(int n) {
//int[] list = random(n);
int[] list = sorted(n);
long startTime = System.currentTimeMillis();
simpleLoop(n);
//bubbleSort(list);
//mergeSort(list);
long endTime = System.currentTimeMillis();
System.out.println("Execution time for n = " + n + " is "
+ (endTime - startTime) + " milliseconds.");
}
private static int[] random(int n) {
int[] list = new int[n];
for (int i = 0; i < n; i++) {
list[i] = (int) (Math.random() * 1000);
}
return list;
}
private static int[] sorted(int n) {
int[] list = new int[n];
for (int i = 0; i < n; i++)
list[i] = i;
return list;
}
private static void simpleLoop(int n){
int k = 0;
for(int i= 1; i <= n; i++)
k = k+5;
}
}
Example Results:
Execution time for n = 1000000 is 6 milliseconds
Execution time for n = 10000000 is 61 milliseconds
Execution time for n = 100000000 is 610 milliseconds
Execution time for n = 1000000000 is 6048 milliseconds
This predicts a linear time complexity for this loop. When the input size increases 10 times, the runtime increases roughly 10 times.

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 9 images

- Implement the design of the Patient class so that the following output is produced:[For BMI, the formula is BMI = weight/height^2, where weight is in kg and height inmeters] Driver Code # Write your code herep1 = Patient("A", 55, 63.0, 158.0)p1.printDetails()print("====================")p2 = Patient("B", 53, 61.0, 149.0)p2.printDetails() Outputarrow_forwardDon't send AI generated answer or plagiarised answer. If I see these things I'll give you multiple downvotes and will report immediately.arrow_forwardimport java.util.Arrays; import java.util.Random; public class Board { /** * Construct a puzzle board by beginning with a solved board and then * making a number of random moves. That some of the possible moves * don't actually change what the board looks like. * * @param moves the number of moves to make when generating the board. */ public Board(int moves) { throw new RuntimeException("Not implemented"); } The board is 5 X 5. You can add classes and imports like rand.arrow_forward
- Using the code in the image provided: Implement a method called summation which adds two integers and returns their sum. INPUT: The first line of input contains an integer a. The Second Line of input containes and integer b. OUTPUT: Print the result which is the sum of a and b.arrow_forwardCan you send output screenshots pleasearrow_forward**Please help fix code, code is not running and not giving output* Question to be answered: Using the code in the image provided: Implement a method called summation which adds two integers and returns their sum. INPUT: The first line of input contains an integer a. The Second Line of input containes and integer b. OUTPUT: Print the result which is the sum of a and b. CODE: import java.util.*;import java.io.*;import java.math.*;class Outcome { /* * Implement method/function with name 'summation' below. * The function accepts following as parameters. * 1. a is of type int. * 2. b is of type int. * return int. */ public static void main(String[] args) { Scanner sc=new Scanner(System.in); int a,b; a=sc.nextInt(); // enter 1st integer b=sc.nextInt(); // enter 2nd integer int sum=summation(a,b); // call function System.out.println(sum); // display message } public static int summation(int a,int b){ //Write your code here int s=0;…arrow_forward
- ***********Instructions******************************************* Complete the following using the attached diceType class as the base: Create a derived class that allows you to reset the number of sides on a die. (Hint: You will need to make one change in diceType.) Overload the << and >> operators. Note: You should test each step in a client program. ****************************************************************** //diceType.h #ifndef H_diceType#define H_diceType class diceType{public:diceType();// Default constructor// Sets numSides to 6 with a random numRolled from 1 - 6 diceType(int);// Constructor to set the number of sides of the dice int roll();// Function to roll a dice.// Randomly generates a number between 1 and numSides// and stores the number in the instance variable numRolled// and returns the number. int getNum() const;// Function to return the number on the top face of the dice.// Returns the value of the instance variable numRolled. private:int…arrow_forward1. Deck.java The Deck class represents a deck of cards. It starts off with 52 cards, but as cards are dealt from the deck. the number of cards becomes smaller. The class has one private instance variable that stores the Cards that are currently in the Deck: private Card] carde; Important Note: The first Card in the array will be considered the card that Is at the top of the deck. Whon cards aro removed from the deck they will be removed from the front of the array. You must implement the flollowing methods for the Deck class: • public Dock) This constructor initializes the Deck with 52 card objacts, representing the 52 cards that are in a standard deck. The cards must be ordered as in the diagram below: Important: The FIRST card in your array should be the Ace of Spades, and the LAST card should be the King of Diamonds. Note that in the picture below, the Ace of Spades is at the TOP of the deck, which is to the left. A234567890 J.0KA234567 25456 10KA2 S45 57|8|90U.0高 • public Deck(Deck…arrow_forwardpublic class utils { * Modify the method below. The method below, myMethod, will be called from a testing function in VPL. * write one line of Java code inside the method that adds one string * to another. It will look something like this: * Assume that 1. String theInput is 2. string mystring is ceorge неllo, пу nane is * we want to update mystring so that it is неllo, пу nane is Ceorge */ public static string mymethod(string theInput){ System.out.println("This method combines two strings."); Systen.out.println("we combined two strings to make mystring); return mystring;arrow_forward
- PLEASE TYPE ONLY IF NOT TYPE THAN DO NOT DO THIS**** PLEASE FOLLOW THE CODE BELOW AND CODE ACCORD TO THAT*** An example of the JFrame subclass with main method is as follows: import java.awt.*; import java.awt.event.*; import javax.swing.*; public class NBAPlayoff extends JFrame { private JTextField txtName; private JTextField txtAge; private NBATeam spurs; private NBAcourtPanel court; private JLabel lMax, lMin, lAvg, lNum; public NBAPlayoff(){ spurs=new NBATeam("Spurs"); court=new NBAcourtPanel(spurs); add(court, BorderLayout.CENTER); JLabel lMax0=new JLabel("Max Age:"); lMax=new JLabel(""); JLabel lMin0=new JLabel("Min Age:"); lMin=new JLabel(""); JLabel lAvg0=new JLabel("Average Age:"); lAvg=new JLabel(""); JLabel lNum0=new JLabel("Number of Players:"); lNum =new JLabel("");…arrow_forwardquestion in java please!arrow_forwardTest your newly created subclasses inside the same TestComputer program you previouslycreated: create two new Laptops and two new Desktops with the data of your choice. UsetoString() method to show all data fields (including the data fields in the super class and sub class)Hint: Make sure to avoid redundancy in your code – no fields implemented in the Parent Classshould be repeated/reimplemented/redefined in children. package project2;class Desktop extends Computer { private int width; private int height; public Desktop() { super(); width = height = 0; } public Desktop(String manufacturer, String diskSize, String manufacturingDate, int numberOfCores, int width, int height) { super(manufacturer, diskSize, manufacturingDate, numberOfCores); this.width = width; this.height = height; } public int getWidth() { return width; } public int getHeight() { return height; } public void setWidth(int width) {…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
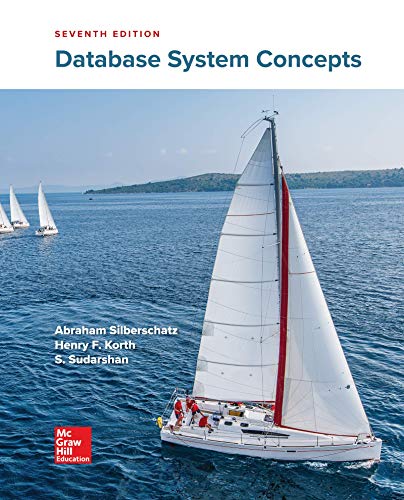
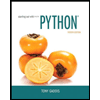
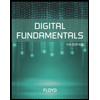
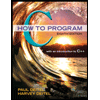
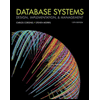
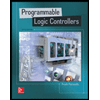