class ADSockDrawer(object): def init _(self): self.drawer = AD() def add_sock (self, color): """Adds a sock of the given color to the drawer. Do it in one line of code. # YOUR CODE HERE self.drawer.update(color) def get_pair (self, color) : """Returns False if there is no pair of the given color, and True if there is. In the latter case, it removes the pair from the drawer. Do it in 5 lines of code or less.""" # YOUR CODE HERE @property def available_colors(self): """Lists the colors for which we have at least two socks available. Do it in 1 line of code."" " # YOUR CODE HERE
class ADSockDrawer(object): def init _(self): self.drawer = AD() def add_sock (self, color): """Adds a sock of the given color to the drawer. Do it in one line of code. # YOUR CODE HERE self.drawer.update(color) def get_pair (self, color) : """Returns False if there is no pair of the given color, and True if there is. In the latter case, it removes the pair from the drawer. Do it in 5 lines of code or less.""" # YOUR CODE HERE @property def available_colors(self): """Lists the colors for which we have at least two socks available. Do it in 1 line of code."" " # YOUR CODE HERE
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question

Transcribed Image Text:### Exercise: Implement the sock drawer class using ADs
class ADSockDrawer(object):
def
init_(self ):
self.drawer = AD()
def add_sock(self, color):
"""Adds a sock of the given color to the drawer.
Do it in one line of code."""
# YOUR CODE HERE
self.drawer.update(color)
def get_pair(self, color):
"""Returns False if there is no pair of the given color, and True
if there is.
In the latter case, it removes the pair from the drawer.
Do it in 5 lines of code or less."""
# YOUR CODE HERE
@property
def available_colors(self):
"""Lists the colors for which we have at least two socks available.
Do it in 1 line of code."""
# YOUR CODE HERE
Expert Solution

Step 1
Source code:
#include <iostream>
#include <vector>
#include <string>
using namespace std;
class Sock{
private:
// Private member intance variables
vector<string> colors;
string hole;
public:
/**
* Default Constructor
*/
Sock(){
}
/**
* Add colors to the sock
*/
void add_color(string color){
colors.push_back(color);
}
/**
* Update Hole yes/no
*/
void set_hole(string hole){
this->hole = hole;
}
/**
* Returns number of Colors
*/
int get_num_colors() const{
return colors.size();
}
/**
* Show colors if no holes
*/
void show_colors(){
if(hole == "yes" || hole == "YES"){
cout << "--Sorry, you can't see the colors because there is a hole in the sock." << endl;
}
else{
cout << "--Sock colors!" << endl;
for(string color : colors)
cout << color << endl;
}
cout << endl;
}
};
class Drawer{
private:
// Private member variables
string name;
int length;
int width;
Sock socks[5];
int maxSocksAllowed;
int currentSize;
public:
/**
* Parameterized Constructor
*/
Drawer(string name, int length, int width){
this->name = name;
this->length = length;
this->width = width;
// Decide maxSocksAllowed, based on area
if(this->length * this->width < 5 ){
maxSocksAllowed = 3;
}
else{
maxSocksAllowed = 5;
}
// set the size
currentSize = 0;
// Print the maxSocksAllowed
cout << name << "'s drawer created. Max number of socks allowed: "
<< maxSocksAllowed << endl;
}
void add_socks(int numSocks){
// Verify if it can be added
if(numSocks > (maxSocksAllowed - currentSize)){
cout << "Sorry " << name
<< ", no space for more socks! Max allowed: " << maxSocksAllowed << endl;
}
else{
// print heading
cout << "Adding " << numSocks << " sock(s) for " << name << "!" << endl;
int index = 0;
while(numSocks > index){
cout << "--Adding sock " << index + 1 << ":" << endl;
cout << "Enter sock colors (on one line): " << endl;
string input;
getline( cin, input);
//create new sock
Sock newSock = Sock();
string color = "";
for (auto x : input)
{
if (x == ' ')
{
newSock.add_color(color);
color = "";
}
else {
color = color + x;
}
}
if(color != ""){
newSock.add_color(color);
}
input = "";
cout << "This sock has " << newSock.get_num_colors() << " color(s). ";
cout << "Does it have a hole in it?" << endl;
getline(cin, input);
// set the hole
newSock.set_hole(input);
// Add it to array
socks[currentSize] = newSock;
currentSize++;
index++;
}
// display remaining spaces
cout << numSocks << " sock(s) added.";
cout << "You still have spaces for " << maxSocksAllowed - currentSize << " sock(s)." << endl; }
}
/**
* Return the sock at the the given index
*/
Sock get_sock(int position){
return socks[position-1];
}
};
int main(int argc,char **argv)
{
Drawer d1("Soheil",2,4);
Drawer d2("Reehan",2,2);
d1.add_socks(2);
d1.add_socks(1);
d1.get_sock(1).show_colors();
d1.get_sock(2).show_colors();
d2.add_socks(4);
return 0;
}
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

Recommended textbooks for you
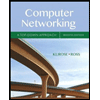
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
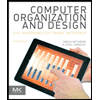
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
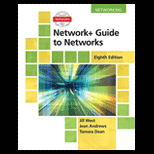
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
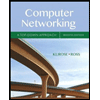
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
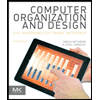
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
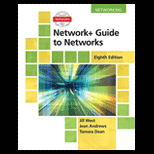
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
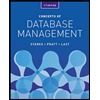
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
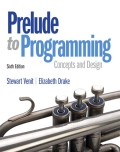
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
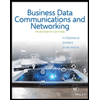
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY