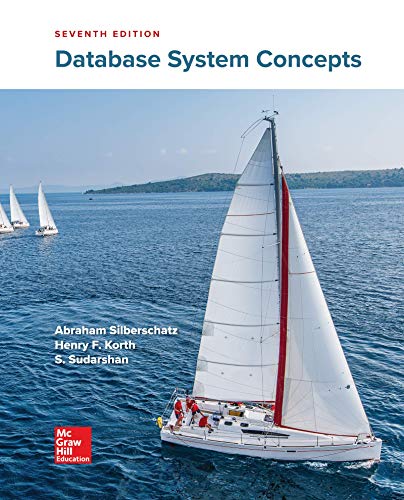
Template below:
include <iostream>
#include <cmath> // Note: Needed for math functions in part (3)
#include <iomanip> // For setprecision
using namespace std;
int main() {
double wallHeight;
double wallWidth;
double wallArea;
cout << "Enter wall height (feet):" << endl;
cin >> wallHeight;
wallWidth = 10.0; // FIXME (1): Prompt user to input wall's width
// Calculate and output wall area
wallArea = 0.0; // FIXME (1): Calculate the wall's area
cout << "Wall area: " << endl; // FIXME (1): Finish the output statement
// FIXME (2): Calculate and output the amount of paint in gallons needed to paint the wall
// FIXME (3): Calculate and output the number of 1 gallon cans needed to paint the wall, rounded up to nearest integer
return 0;
}
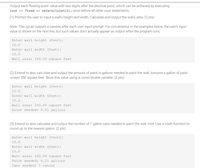

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- #include #include #include "Product.h" using namespace std; int main() { vector productList; Product currProduct; int currPrice; string currName; unsigned int i; Product resultProduct; cin>> currPrice; while (currPrice > 0) { } cin>> currPrice; main.cpp cin>> currName; currProduct.SetPriceAndName (currPrice, currName); productList.push_back(currProduct); resultProduct = productList.at (0); for (i = 0; i < productList.size(); ++i) { Type the program's output Product.h 1 CSE Scanned Product.cpp if (productList.at (i).GetPrice () < resultProduct.GetPrice ()) { resultProduct = productList.at(i); } AM cout << "$" << resultProduct.GetPrice() << " " << resultProduct. GetName() << endl; return 0; Input 10 Cheese 6 Foil 7 Socks -1 Outputarrow_forwardC++ need help with part d to g only Assume the Product structure is declared as follows: struct Product { string description; // Product description int partNum; // Part number double cost; // Product cost }; (c) Declare a dynamic array of Products of size 5 and name it ”items”. Then initilize it with user input values. (d) Write a print function (not as a member of the struct) and pass a pointer to the pointer that points to the array (double pointer) and print all the items of the array in that function. (e) Define a max function (not as a member of the struct) that gets an array of items as an input and returns a pointer to the max element of the array. (f) Declare a 3 by 3 two dimensional dynamic array and populate it. (g) Define an output function that takes a stream object and a pointer to a 2D array as arguments and outputs data members of objects in format of 3*3 table into the given stream. Test your function both with an output file…arrow_forwardC++ Program #include <iostream>#include <cstdlib>#include <ctime>using namespace std; int getData() { return (rand() % 100);} class Node {public: int data; Node* next;}; class LinkedList{public: LinkedList() { // constructor head = NULL; } ~LinkedList() {}; // destructor void addNode(int val); void addNodeSorted(int val); void displayWithCount(); int size(); void deleteAllNodes(); bool exists(int val);private: Node* head;}; // function to check data exist in a listbool LinkedList::exists(int val){ if (head == NULL) { return false; } else { Node* temp = head; while (temp != NULL) { if(temp->data == val){ return true; } temp = temp->next; } } return false;} // function to delete all data in a listvoid LinkedList::deleteAllNodes(){ if (head == NULL) { cout << "List is empty, No need to delete…arrow_forward
- Main.cpp #include <iostream>#include "Deck.h" int main() { Deck deck; deck.shuffle(); std::cout << "WAR Card Game\n\n"; std::cout << "Dealing cards...\n\n"; Card player1Card = deck.Deal(); Card player2Card = deck.Deal(); std::cout << "Player 1's card: "; player1Card.showCard(); std::cout << std::endl; std::cout << "Player 2's card: "; player2Card.showCard(); std::cout << std::endl; int player1Value = player1Card.getValue(); int player2Value = player2Card.getValue(); if (player1Value > player2Value) { std::cout << "Player 1 wins!" << std::endl; } else if (player1Value < player2Value) { std::cout << "Player 2 wins!" << std::endl; } else { std::cout << "It's a tie!" << std::endl; } return 0;} Card.h #ifndef CARD_H#define CARD_H class Card {public: Card(); Card(char r, char s); int getValue(); void showCard();…arrow_forwardMain.cpp #include <iostream>#include "Deck.h" int main() { Deck deck; deck.shuffle(); std::cout << "WAR Card Game\n\n"; std::cout << "Dealing cards...\n\n"; Card player1Card = deck.Deal(); Card player2Card = deck.Deal(); std::cout << "Player 1's card: "; player1Card.showCard(); std::cout << std::endl; std::cout << "Player 2's card: "; player2Card.showCard(); std::cout << std::endl; int player1Value = player1Card.getValue(); int player2Value = player2Card.getValue(); if (player1Value > player2Value) { std::cout << "Player 1 wins!" << std::endl; } else if (player1Value < player2Value) { std::cout << "Player 2 wins!" << std::endl; } else { std::cout << "It's a tie!" << std::endl; } return 0;} Card.h #ifndef CARD_H#define CARD_H class Card {public: Card(); Card(char r, char s); int getValue(); void showCard();…arrow_forwardC++ complete magic Square #include <iostream> using namespace std; /*int f( int x, int y, int* p, int* q ){if ( y == 0 ){p = 0, q = 0;return 404; // status: Error 404}*p = x * y; // product*q = x / y; // quotient return 200; // status: OK 200} int main(){int p, q;int status = f(10, 2, &p, &q);if ( status == 404 ){cout << "[ERR] / by zero!" << endl;return 0;}cout << p << endl;cout << q << endl; return 0;}*/ /*struct F_Return{int p;int q;int status;}; F_Return f( int x, int y ){F_Return r;if ( y == 0 ){r.p = 0, r.q = 0;r.status = 404;return r;}r.p = x * y;r.q = x / y;r.status = 200;return r;} int main(){F_Return r = f(10, 0);if ( r.status == 404 ){cout << "[ERR] / by zero" << endl;return 0;}cout << r.p << endl;cout << r.q << endl;return 0;}*/ int sumByRow(int *m, int nrow, int ncol, int row){ int total = 0;for ( int j = 0; j < ncol; j++ ){total += m[row * ncol + j]; //m[row][j];}return total; } /*…arrow_forward
- C++ This program does not compile! Spot the error and give the line number. Presume that the header file is error free and // Implementation file for the Rectangle class.1. #include "Rectangle.h" // Needed for the Rectangle class2. #include <iostream> // Needed for cout3. #include <cstdlib> // Needed for the exit function4. using namespace std; //***********************************************************// setWidth sets the value of the member variable width. *//*********************************************************** 5. void Rectangle::setWidth(double w){6. if (w >= 0)7. width = w;8. else{9. cout << "Invalid width\n";10. exit(EXIT_FAILURE);}} //***********************************************************// setLength sets the value of the member variable length. *//*********************************************************** 11. void Rectangle::setLength(double len){12. if (len >= 0)13. length = len;14. else{15. cout << "Invalid length\n";16.…arrow_forwardC++ Find the errors in the code for Date Class and Source.cpp program and fix them. source.cpp include <iostream>#include "Date.h" // include definition of class Date from Date.h using namespace std; // function main begins program executionint main(){Date date(); // create a Date object for May 6, 1981 // display the values of the three Date data members cout << "Month: " << date.getMonth() << endl;cout « "Day: " << date.getDay() << endl;cout << "Year: " << date.getYear(2017) << endl; cout << "\nOriginal date:" << endl;date = displayDate(); // output the Date// modify the Date setMonth(13);setDay(1);setYear(2005); cout "\nNew date:" << endl;date.displayDate(); // output the modified date } // end main Date.h // class Data definition#include <iostream>using namespace std; //Data constructor that initializes the three data members;class Date{publicdate(m, d, y){setMonth();setDay();setYear();};// set monthvoid…arrow_forward/* Programming concept: structures Program should: be a C program, define a structure to store the year, month, day, and high temperature. write a function get_data that has a parameter that is a pointer to a struct and reads from the user into the struct pointed to by the parameter. write a function print_data that takes the struct pointer as a parameter and prints the data. in main, declare a struct, call the get_data function, call the print_data function. */ #include <stdio.h> int main(int argc, char *argv[]) { return 0;}arrow_forward
- Determine all the output from the following program as it would appear on the screen. void func1(int); void func2(int = 4, int = 5, int = 2); int func3(int &, int, int); %3D int main() { int x = 0, z = 0, y = 2; func1(y); cout << y << endl; func2(x, y, z); func2(); func3(x, y, z); = Z func1(x); cout << x << " " << y << " " << z << endl; return 0; } void func1(int b) { static int a;arrow_forwardC++ Program: #include <iostream>#include <string> using namespace std; const int AIRPORT_COUNT = 12;string airports[AIRPORT_COUNT] = {"DAL","ABQ","DEN","MSY","HOU","SAT","CRP","MID","OKC","OMA","MDW","LAX"}; int main(){ // define stack (or queue ) here string origin; string dest; string citypair; cout << "Loading the CONTAINER ..." << endl; // LOAD THE STACK ( or queue) HERE // Create all the possible Airport combinations that could exist from the list provided. // i.e DALABQ, DALDEN, ...., ABQDAL, ABQDEN ... // DO NOT Load SameSame - DALDAL, ABQABQ, etc .. cout << "Getting data from the CONTAINER ..." << endl;// Retrieve data from the STACK/QUEUE here } Using the attached program (AirportCombos.cpp), create a list of strings to process and place on a STL STACK container. The provided code is meant to be generic. Using the provided 3 char airport codes, create a 6 character string that is the origin &…arrow_forward#ifndef INT_SET_H#define INT_SET_H #include <iostream> class IntSet{public: static const int DEFAULT_CAPACITY = 1; IntSet(int initial_capacity = DEFAULT_CAPACITY); IntSet(const IntSet& src); ~IntSet(); IntSet& operator=(const IntSet& rhs); int size() const; bool isEmpty() const; bool contains(int anInt) const; bool isSubsetOf(const IntSet& otherIntSet) const; void DumpData(std::ostream& out) const; IntSet unionWith(const IntSet& otherIntSet) const; IntSet intersect(const IntSet& otherIntSet) const; IntSet subtract(const IntSet& otherIntSet) const; void reset(); bool add(int anInt); bool remove(int anInt); private: int* data; int capacity; int used; void resize(int new_capacity);}; bool operator==(const IntSet& is1, const IntSet& is2); #endifarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
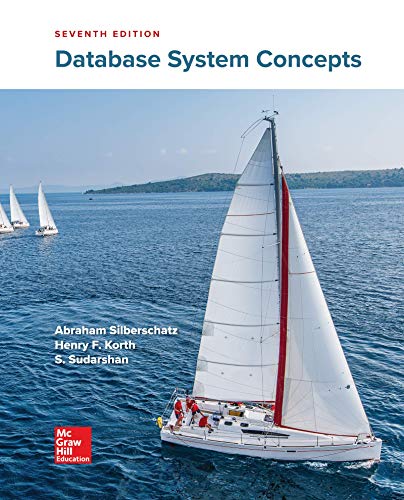
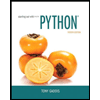
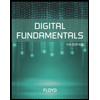
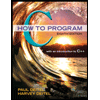
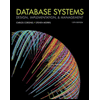
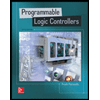