import java.util.Scanner; import java.util.Random; class HotelBooking { // (Same as before) // Additional methods for Display Booking and Cancel Booking public String getGuestName() { return guestName; } public int getBookingID() { return bookingID; } } public class HotelBookingSystem { public static void main(String[] args) { Scanner scanner = new Scanner(System.in); // (Same as before) HotelBooking booking = new HotelBooking(guestName, selectedRoomType, roomPrice, requestedRooms, confirmationCode); // (Same as before) System.out.print("\nDo you want to display booking details? (Y/N): "); char displayChoice = scanner.next().charAt(0); if (displayChoice == 'Y' || displayChoice == 'y') { displayBookingDetails(booking); } System.out.print("\nDo you want to cancel booking? (Y/N): "); char cancelChoice = scanner.next().charAt(0); if (cancelChoice == 'Y' || cancelChoice == 'y') { cancelBooking(booking); } scanner.close(); } // Additional methods for Display Booking and Cancel Booking public static void displayBookingDetails(HotelBooking booking) { System.out.println("\nBooking Details:"); System.out.println("Guest Name: " + booking.getGuestName()); System.out.println("Booking ID: " + booking.getBookingID()); System.out.println("Room Type: " + booking.roomType); System.out.println("Number of Rooms: " + booking.numberOfRooms); System.out.println("Total cost: RM" + booking.calculateTotalCost()); } public static void cancelBooking(HotelBooking booking) { System.out.println("\nBooking for " + booking.getGuestName() + " has been cancelled."); } } add exist to the above an do the flowchart with symbols and the pseudocode
import java.util.Scanner;
import java.util.Random;
class HotelBooking {
// (Same as before)
// Additional methods for Display Booking and Cancel Booking
public String getGuestName() {
return guestName;
}
public int getBookingID() {
return bookingID;
}
}
public class HotelBookingSystem {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
// (Same as before)
HotelBooking booking = new HotelBooking(guestName, selectedRoomType, roomPrice, requestedRooms, confirmationCode);
// (Same as before)
System.out.print("\nDo you want to display booking details? (Y/N): ");
char displayChoice = scanner.next().charAt(0);
if (displayChoice == 'Y' || displayChoice == 'y') {
displayBookingDetails(booking);
}
System.out.print("\nDo you want to cancel booking? (Y/N): ");
char cancelChoice = scanner.next().charAt(0);
if (cancelChoice == 'Y' || cancelChoice == 'y') {
cancelBooking(booking);
}
scanner.close();
}
// Additional methods for Display Booking and Cancel Booking
public static void displayBookingDetails(HotelBooking booking) {
System.out.println("\nBooking Details:");
System.out.println("Guest Name: " + booking.getGuestName());
System.out.println("Booking ID: " + booking.getBookingID());
System.out.println("Room Type: " + booking.roomType);
System.out.println("Number of Rooms: " + booking.numberOfRooms);
System.out.println("Total cost: RM" + booking.calculateTotalCost());
}
public static void cancelBooking(HotelBooking booking) {
System.out.println("\nBooking for " + booking.getGuestName() + " has been cancelled.");
}
}
add exist to the above
an do the flowchart with symbols and the pseudocode

Step by step
Solved in 4 steps with 1 images

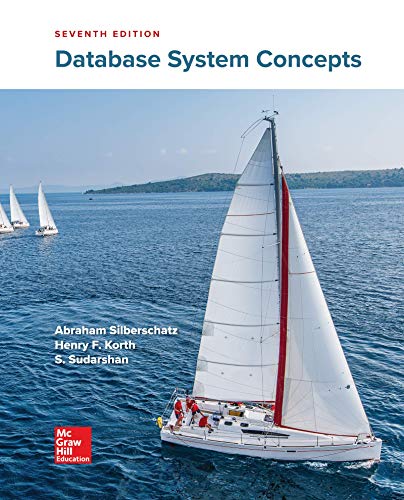
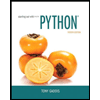
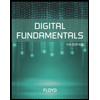
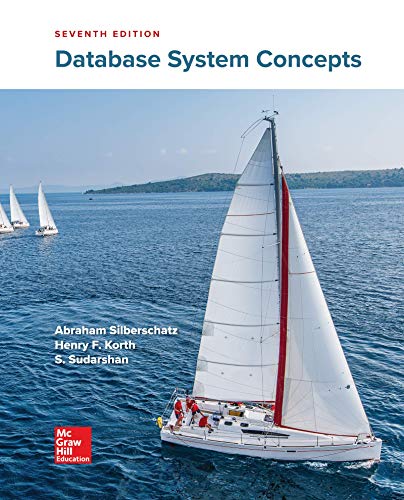
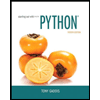
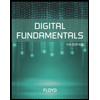
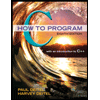
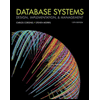
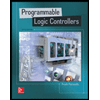