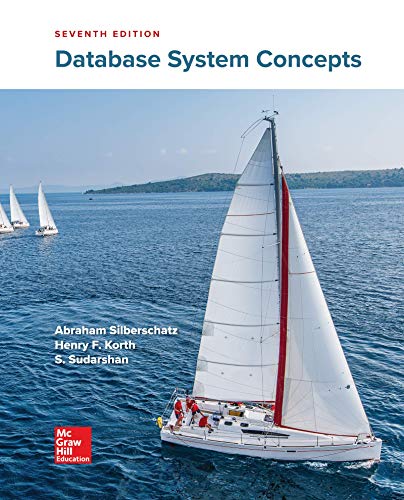
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Question
4f. Provide the output of the following C++ code?
#include <iostream>
using namespace std;
template <typename T = float, int count = 3>
T multIt(T x)
{
for(int ii = 0; ii < count; ii++)
{
x = x * x;
}
return x;
};
int main()
{
float xx = 2.1;
cout << xx << ": " << multIt<>(xx) << endl;
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by stepSolved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- I need help making this C program which uses pointers. #include<stdio.h> //for printf and scanf #include<ctype.h> //for tolower function //function prototypes void Greeting(); //welcome the user to the gas station app void ViewAndGetSelection(char* selectionPtr); //input: the user's selection (input/output parameter) //display the program options and get the users selection //use an input/output parameter for the selection void ProcessSelection(char selection, double* balancePtr); //input: the user's selection by copy (input parameter) //input: the account balance (input/output parameter) //display a message that the selection has been entered //display the balance when the user enters 'b' //allow the user to add money to the account when the user enters 'u' int main() { char choiceInMain; double balanceInMain = 0.00; //call the greeting function //view and get the selection - function call //change the selection to lower or upper case //make sure the user did not enter q…arrow_forwardC++ Given code is #pragma once#include <iostream>#include "ourvector.h"using namespace std; ourvector<int> intersect(ourvector<int> &v1, ourvector<int> &v2) { // TO DO: write this function return {};}arrow_forwardQuestion 1 is already done need help with the others though This is the C code I have so far #include <stdio.h> #include <stdlib.h> struct employees { char name[20]; int ssn[9]; int yearBorn, salary; }; struct employees **emps = new employees()[10]; //Added new statement ---- bartleby // function to read the employee data from the user void readEmployee(struct employees *emp) { printf("Enter name: "); gets(emp->name); printf("Enter ssn: "); for(int i =0; i <9; i++) scanf("%d", &emp->ssn[i]); printf("Enter birth year: "); scanf("%d", &emp->yearBorn); printf("Enter salary: "); scanf("%d", &emp->salary); } // function to create a pointer of employee type struct employees *createEmployee() { // creating the pointer struct employees *emp = malloc(sizeof(struct employees)); // function to read the data readEmployee(emp); // returning the data return emp; } // function to…arrow_forward
- >> IN C PROGRAMMING LANGUAGE ONLY << COPY OF DEFAULT CODE, ADD SOLUTION INTO CODE IN C #include <stdio.h>#include <stdlib.h>#include <string.h> #include "GVDie.h" int RollSpecificNumber(GVDie die, int num, int goal) {/* Type your code here. */} int main() {GVDie die = InitGVDie(); // Create a GVDie variabledie = SetSeed(15, die); // Set the GVDie variable with seed value 15int num;int goal;int rolls; scanf("%d", &num);scanf("%d", &goal);rolls = RollSpecificNumber(die, num, goal); // Should return the number of rolls to reach total.printf("It took %d rolls to get a \"%d\" %d times.\n", rolls, num, goal); return 0;}arrow_forwardConsider the following C++ code segment: int d = 20; int f(int b) { static int c = 0; c *= b; return c; } int main() { int a; cin >> a; cout << f(a) * d << endl; }For each variable a, b, c, d: identify all type bindings and storage bindings for each binding, determine when the binding occurs identify the scope and lifetimearrow_forwardQuestion 1 is already done need help with the others though This is the C code I have so far #include <stdio.h> #include <stdlib.h> struct employees { char name[20]; int ssn[9]; int yearBorn, salary; }; struct employees **emps = new employees()[10]; //Added new statement ---- bartleby // function to read the employee data from the user void readEmployee(struct employees *emp) { printf("Enter name: "); gets(emp->name); printf("Enter ssn: "); for(int i =0; i <9; i++) scanf("%d", &emp->ssn[i]); printf("Enter birth year: "); scanf("%d", &emp->yearBorn); printf("Enter salary: "); scanf("%d", &emp->salary); } // function to create a pointer of employee type struct employees *createEmployee() { // creating the pointer struct employees *emp = malloc(sizeof(struct employees)); // function to read the data readEmployee(emp); // returning the data return emp; } // function to…arrow_forward
- C++, Please add a function where it can display the median, the maximum number, the minimum number, in an array entered by the user. Code: #include<iostream> using namespace std; class Search_Dynamic_Array { public: Search_Dynamic_Array(); int getArrSize(); void getArray(); void setArrsize(int); void setArray(int*); int getSum(int*); private: int arr_size; int arr[]; }; Search_Dynamic_Array::Search_Dynamic_Array() { //ctor } int Search_Dynamic_Array::getArrSize() { return arr_size; } void Search_Dynamic_Array::getArray() { cout << "The elements of the array are: "; for(int i=0; i<arr_size; i++){ cout << arr[i] << " "; } } void Search_Dynamic_Array::setArrsize(int s) { arr_size=s; } void Search_Dynamic_Array::setArray(int a[]) { for(int i=0; i<arr_size; i++){ arr[i]=a[i]; } } int Search_Dynamic_Array::getSum(int a[]) { int sum; for(int i=0; i<arr_size; i++){ sum +=arr[i]; } return sum; } int main() { int s=1, a[s]; cout << "Enter the size of the…arrow_forwardQuestion 1 is already done need help with the others though This is the C code I have so far #include <stdio.h> #include <stdlib.h> struct employees { char name[20]; int ssn[9]; int yearBorn, salary; }; struct employees **emps = new employees()[10]; //Added new statement ---- bartleby // function to read the employee data from the user void readEmployee(struct employees *emp) { printf("Enter name: "); gets(emp->name); printf("Enter ssn: "); for(int i =0; i <9; i++) scanf("%d", &emp->ssn[i]); printf("Enter birth year: "); scanf("%d", &emp->yearBorn); printf("Enter salary: "); scanf("%d", &emp->salary); } // function to create a pointer of employee type struct employees *createEmployee() { // creating the pointer struct employees *emp = malloc(sizeof(struct employees)); // function to read the data readEmployee(emp); // returning the data return emp; } // function to…arrow_forward#include <iostream> #include <string> #include <cstdlib> using namespace std; template<class T> T func(T a) { cout<<a; return a; } template<class U> void func(U a) { cout<<a; } int main(int argc, char const *argv[]) { int a = 5; int b = func(a); return 0; } Give output for this code.arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
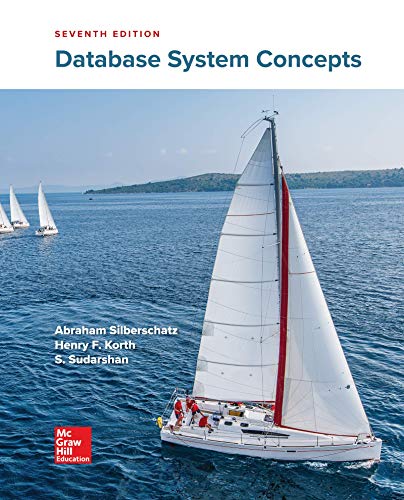
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
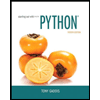
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
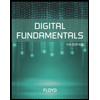
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
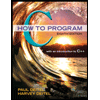
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
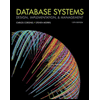
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
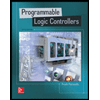
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education