This expands the previous work to enable handling multiple employees. You will do this by maintaining a global array of pointers to employees. 1) Define a global variable Emps that is an array of pointers to employee structs. 2) Change your createEmployee function to add the new employee to the Emps array. It should place it in the first slot that contains a NULL. 3) Write a listEmployees function that will call your display function on each employee in the array. 4) Change main to have a loop that looks for user commands. • If the user types HIRE, you should call createEmployee. • If the user types LIST, you should call listEmployees. • If the user types QUIT, you should exit the loop, which will then cause the program to exit. 5) Write a findEmployee function that takes a string parameter and returns the employee pointer with that name or NULL if no such person exists. Change main to add a FIND name command that will find and display a single employee.
Question 1 is already done need help with the others though
This is the C code I have so far
#include <stdio.h>
#include <stdlib.h>
struct employees
{
char name[20];
int ssn[9];
int yearBorn, salary;
};
struct employees **emps = new employees()[10]; //Added new statement ---- bartleby
// function to read the employee data from the user
void readEmployee(struct employees *emp)
{
printf("Enter name: ");
gets(emp->name);
printf("Enter ssn: ");
for(int i =0; i <9; i++)
scanf("%d", &emp->ssn[i]);
printf("Enter birth year: ");
scanf("%d", &emp->yearBorn);
printf("Enter salary: ");
scanf("%d", &emp->salary);
}
// function to create a pointer of employee type
struct employees *createEmployee()
{
// creating the pointer
struct employees *emp = malloc(sizeof(struct employees));
// function to read the data
readEmployee(emp);
// returning the data
return emp;
}
// function to print the employee data to console
void display(struct employees *e)
{
printf("%s", e->name);
printf(" %d%d%d-%d%d-%d%d%d%d", e->ssn[0], e->ssn[1], e->ssn[2], e->ssn[3], e->ssn[4], e->ssn[5], e->ssn[6], e->ssn[7], e->ssn[8]);
printf(" %d", e->yearBorn);
printf("\n$%d.", e->salary);
}
// function to free the memory of the employee pointer
void releaseEmployee(struct employees *e)
{
free(e);
}
// main method
int main()
{
// creating the employee
struct employees *emp = createEmployee();
// printing the information
display(emp);
// free the memory
releaseEmployee(emp);
return0;
}


Step by step
Solved in 2 steps

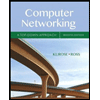
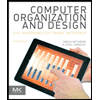
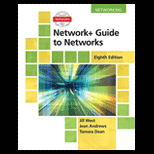
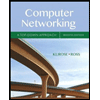
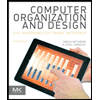
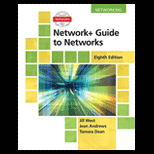
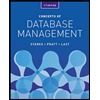
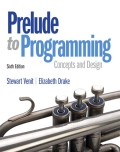
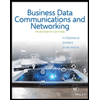