Use the array based list headers for queue. Call your function to test its functionality. Here are the headers: #ifndef H_arrayListType #define H_arrayListType #include using namespace std; template class arrayListType { public: const arrayListType& operator=(const arrayListType&); //Overloads the assignment operator bool isEmpty() const; //Function to determine whether the list is empty //Postcondition: Returns true if the list is empty; // otherwise, returns false. bool isFull() const; //Function to determine whether the list is full //Postcondition: Returns true if the list is full; // otherwise, returns false. int listSize() const; //Function to determine the number of elements in //the list. //Postcondition: Returns the value of length. int maxListSize() const; //Function to determine the maximum size of the list //Postcondition: Returns the value of maxSize. void print() const; //Function to output the elements of the list //Postcondition: Elements of the list are output on the // standard output device. bool isItemAtEqual(int location, const elemType& item) const; //Function to determine whether item is the same as //the item in the list at the position specified //by location. //Postcondition: Returns true if the list[location] // is the same as item; otherwise, // returns false. // If location is out of range, an // appropriate message is displayed. virtualvoid insertAt(int location, const elemType& insertItem) = 0; //Function to insert insertItem in the list at the //position specified by location. //Note that this is an abstract function. //Postcondition: Starting at location, the elements of // the list are shifted down, // list[location] = insertItem; length++; // If the list is full or location is out of // range, an appropriate message is displayed. virtualvoid insertEnd(const elemType& insertItem) = 0; //Function to insert insertItem an item at the end of //the list. Note that this is an abstract function. //Postcondition: list[length] = insertItem; and length++; // If the list is full, an appropriate // message is displayed. void removeAt(int location); //Function to remove the item from the list at the //position specified by location //Postcondition: The list element at list[location] is // removed and length is decremented by 1. // If location is out of range, an // appropriate message is displayed. void retrieveAt(int location, elemType& retItem) const; //Function to retrieve the element from the list at the //position specified by location //Postcondition: retItem = list[location] // If location is out of range, an // appropriate message is displayed. virtualvoid replaceAt(int location, const elemType& repItem) = 0; //Function to replace repItem the elements in the list //at the position specified by location. //Note that this is an abstract function. //Postcondition: list[location] = repItem // If location is out of range, an // appropriate message is displayed. void clearList(); //Function to remove all the elements from the list //After this operation, the size of the list is zero. //Postcondition: length = 0; virtualint seqSearch(const elemType& searchItem) const = 0; //Function to search the list for searchItem. //Note that this is an abstract function. //Postcondition: If the item is found, returns the // location in the array where the item is // found; otherwise, returns -1. virtualvoid remove(const elemType& removeItem) = 0; //Function to remove removeItem from the list. //Note that this is an abstract function. //Postcondition: If removeItem is found in the list, // it is removed from the list and length // is decremented by one. arrayListType(int size = 100); //Constructor //Creates an array of the size specified by the //parameter size. The default array size is 100. //Postcondition: The list points to the array, length = 0, // and maxSize = size; arrayListType (const arrayListType& otherList); //Copy constructor virtual ~arrayListType(); //Destructor //Deallocate the memory occupied by the array. protected: elemType *list; //array to hold the list elements int length; //variable to store the length of the list int maxSize; //variable to store the maximum //size of the list }; template bool arrayListType::isEmpty() const { return (this->length == 0); } template bool arrayListType::isFull() const { return (this->length == this->maxSize); } template int arrayListType::listSize() const { return this->length; } template int arrayListType::maxListSize() const { return this->maxSize; } template void arrayListType::print() const { for (int i = 0; i < this->length; i++) cout << list[i] << " "; cout << endl; } template bool arrayListType::isItemAtEqual(int location, const elemType& item) const { if (location < 0 || location >= this->length) { cout << "The location of the item to be removed " << "is out of range." << endl; returnfalse; } else return (list[location] == item); } #endif
Use the array based list headers for queue. Call your function to test its functionality. Here are the headers: #ifndef H_arrayListType #define H_arrayListType #include using namespace std; template class arrayListType { public: const arrayListType& operator=(const arrayListType&); //Overloads the assignment operator bool isEmpty() const; //Function to determine whether the list is empty //Postcondition: Returns true if the list is empty; // otherwise, returns false. bool isFull() const; //Function to determine whether the list is full //Postcondition: Returns true if the list is full; // otherwise, returns false. int listSize() const; //Function to determine the number of elements in //the list. //Postcondition: Returns the value of length. int maxListSize() const; //Function to determine the maximum size of the list //Postcondition: Returns the value of maxSize. void print() const; //Function to output the elements of the list //Postcondition: Elements of the list are output on the // standard output device. bool isItemAtEqual(int location, const elemType& item) const; //Function to determine whether item is the same as //the item in the list at the position specified //by location. //Postcondition: Returns true if the list[location] // is the same as item; otherwise, // returns false. // If location is out of range, an // appropriate message is displayed. virtualvoid insertAt(int location, const elemType& insertItem) = 0; //Function to insert insertItem in the list at the //position specified by location. //Note that this is an abstract function. //Postcondition: Starting at location, the elements of // the list are shifted down, // list[location] = insertItem; length++; // If the list is full or location is out of // range, an appropriate message is displayed. virtualvoid insertEnd(const elemType& insertItem) = 0; //Function to insert insertItem an item at the end of //the list. Note that this is an abstract function. //Postcondition: list[length] = insertItem; and length++; // If the list is full, an appropriate // message is displayed. void removeAt(int location); //Function to remove the item from the list at the //position specified by location //Postcondition: The list element at list[location] is // removed and length is decremented by 1. // If location is out of range, an // appropriate message is displayed. void retrieveAt(int location, elemType& retItem) const; //Function to retrieve the element from the list at the //position specified by location //Postcondition: retItem = list[location] // If location is out of range, an // appropriate message is displayed. virtualvoid replaceAt(int location, const elemType& repItem) = 0; //Function to replace repItem the elements in the list //at the position specified by location. //Note that this is an abstract function. //Postcondition: list[location] = repItem // If location is out of range, an // appropriate message is displayed. void clearList(); //Function to remove all the elements from the list //After this operation, the size of the list is zero. //Postcondition: length = 0; virtualint seqSearch(const elemType& searchItem) const = 0; //Function to search the list for searchItem. //Note that this is an abstract function. //Postcondition: If the item is found, returns the // location in the array where the item is // found; otherwise, returns -1. virtualvoid remove(const elemType& removeItem) = 0; //Function to remove removeItem from the list. //Note that this is an abstract function. //Postcondition: If removeItem is found in the list, // it is removed from the list and length // is decremented by one. arrayListType(int size = 100); //Constructor //Creates an array of the size specified by the //parameter size. The default array size is 100. //Postcondition: The list points to the array, length = 0, // and maxSize = size; arrayListType (const arrayListType& otherList); //Copy constructor virtual ~arrayListType(); //Destructor //Deallocate the memory occupied by the array. protected: elemType *list; //array to hold the list elements int length; //variable to store the length of the list int maxSize; //variable to store the maximum //size of the list }; template bool arrayListType::isEmpty() const { return (this->length == 0); } template bool arrayListType::isFull() const { return (this->length == this->maxSize); } template int arrayListType::listSize() const { return this->length; } template int arrayListType::maxListSize() const { return this->maxSize; } template void arrayListType::print() const { for (int i = 0; i < this->length; i++) cout << list[i] << " "; cout << endl; } template bool arrayListType::isItemAtEqual(int location, const elemType& item) const { if (location < 0 || location >= this->length) { cout << "The location of the item to be removed " << "is out of range." << endl; returnfalse; } else return (list[location] == item); } #endif
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
Use the array based list headers for queue. Call your function to test its functionality. Here are the headers:
#ifndef H_arrayListType
#define H_arrayListType
#include <iostream>
using namespace std;
template <class elemType>
class arrayListType
{
public:
const arrayListType<elemType>&
operator=(const arrayListType<elemType>&);
//Overloads the assignment operator
bool isEmpty() const;
//Function to determine whether the list is empty
//Postcondition: Returns true if the list is empty;
// otherwise, returns false.
bool isFull() const;
//Function to determine whether the list is full
//Postcondition: Returns true if the list is full;
// otherwise, returns false.
int listSize() const;
//Function to determine the number of elements in
//the list.
//Postcondition: Returns the value of length.
int maxListSize() const;
//Function to determine the maximum size of the list
//Postcondition: Returns the value of maxSize.
void print() const;
//Function to output the elements of the list
//Postcondition: Elements of the list are output on the
// standard output device.
bool isItemAtEqual(int location, const elemType& item) const;
//Function to determine whether item is the same as
//the item in the list at the position specified
//by location.
//Postcondition: Returns true if the list[location]
// is the same as item; otherwise,
// returns false.
// If location is out of range, an
// appropriate message is displayed.
virtualvoid insertAt(int location, const elemType& insertItem) = 0;
//Function to insert insertItem in the list at the
//position specified by location.
//Note that this is an abstract function.
//Postcondition: Starting at location, the elements of
// the list are shifted down,
// list[location] = insertItem; length++;
// If the list is full or location is out of
// range, an appropriate message is displayed.
virtualvoid insertEnd(const elemType& insertItem) = 0;
//Function to insert insertItem an item at the end of
//the list. Note that this is an abstract function.
//Postcondition: list[length] = insertItem; and length++;
// If the list is full, an appropriate
// message is displayed.
void removeAt(int location);
//Function to remove the item from the list at the
//position specified by location
//Postcondition: The list element at list[location] is
// removed and length is decremented by 1.
// If location is out of range, an
// appropriate message is displayed.
void retrieveAt(int location, elemType& retItem) const;
//Function to retrieve the element from the list at the
//position specified by location
//Postcondition: retItem = list[location]
// If location is out of range, an
// appropriate message is displayed.
virtualvoid replaceAt(int location, const elemType& repItem) = 0;
//Function to replace repItem the elements in the list
//at the position specified by location.
//Note that this is an abstract function.
//Postcondition: list[location] = repItem
// If location is out of range, an
// appropriate message is displayed.
void clearList();
//Function to remove all the elements from the list
//After this operation, the size of the list is zero.
//Postcondition: length = 0;
virtualint seqSearch(const elemType& searchItem) const = 0;
//Function to search the list for searchItem.
//Note that this is an abstract function.
//Postcondition: If the item is found, returns the
// location in the array where the item is
// found; otherwise, returns -1.
virtualvoid remove(const elemType& removeItem) = 0;
//Function to remove removeItem from the list.
//Note that this is an abstract function.
//Postcondition: If removeItem is found in the list,
// it is removed from the list and length
// is decremented by one.
arrayListType(int size = 100);
//Constructor
//Creates an array of the size specified by the
//parameter size. The default array size is 100.
//Postcondition: The list points to the array, length = 0,
// and maxSize = size;
arrayListType (const arrayListType<elemType>& otherList);
//Copy constructor
virtual ~arrayListType();
//Destructor
//Deallocate the memory occupied by the array.
protected:
elemType *list; //array to hold the list elements
int length; //variable to store the length of the list
int maxSize; //variable to store the maximum
//size of the list
};
template <class elemType>
bool arrayListType<elemType>::isEmpty() const
{
return (this->length == 0);
}
template <class elemType>
bool arrayListType<elemType>::isFull() const
{
return (this->length == this->maxSize);
}
template <class elemType>
int arrayListType<elemType>::listSize() const
{
return this->length;
}
template <class elemType>
int arrayListType<elemType>::maxListSize() const
{
return this->maxSize;
}
template <class elemType>
void arrayListType<elemType>::print() const
{
for (int i = 0; i < this->length; i++)
cout << list[i] << " ";
cout << endl;
}
template <class elemType>
bool arrayListType<elemType>::isItemAtEqual(int location,
const elemType& item) const
{
if (location < 0 || location >= this->length)
{
cout << "The location of the item to be removed "
<< "is out of range." << endl;
returnfalse;
}
else
return (list[location] == item);
}
#endif

Transcribed Image Text:Create a function for the queue headers that removes a consecutive list of items. The function will accept 2 parameters, where the first parameter is a value in the list and the second parameter is a whole number. The function will search for the first
parameter, and if the value is in the list it will remove the items following the searched value nth times. Use the following example:
Example 1:
the list has 10 items namely a, s, c, v, m, b, x, p,k, i
the function accepts 2 parameters v and 3.
The resulting link will be a, s, c, x, p,k, I
Example 2:
the list has 10 items namely 0, 9, 8, 7, 6, 5, 4, 3, 2, 1
the function accepts 2 parameters 6 and 4.
The resulting link will be 0, 9, 8, 7, 2, 1
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 1 images

Recommended textbooks for you
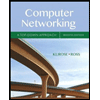
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
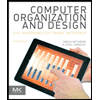
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
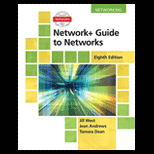
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
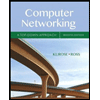
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
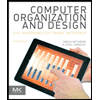
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
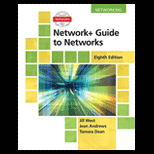
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
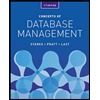
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
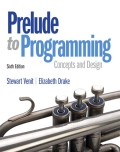
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
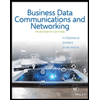
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY