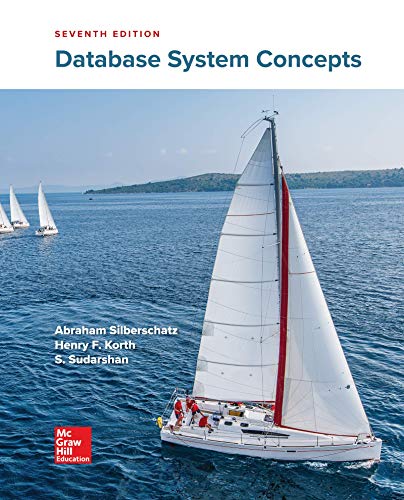
#ifndef NODES_LLOLL_H
#define NODES_LLOLL_H
#include <iostream> // for ostream
namespace CS3358_SP2023_A5P2
{
// child node
struct CNode
{
int data;
CNode* link;
};
// parent node
struct PNode
{
CNode* data;
PNode* link;
};
// toolkit functions for LLoLL based on above node definitions
void Destroy_cList(CNode*& cListHead);
void Destroy_pList(PNode*& pListHead);
void ShowAll_DF(PNode* pListHead, std::ostream& outs);
void ShowAll_BF(PNode* pListHead, std::ostream& outs);
}
#endif
#include "nodes_LLoLL.h"
#include "cnPtrQueue.h"
#include <iostream>
using namespace std;
namespace CS3358_SP2023_A5P2
{
// do breadth-first (level) traversal and print data
void ShowAll_BF(PNode* pListHead, ostream& outs)
{
cnPtrQueue queue;
CNode* currentNode;
queue.push(lloLLPtr->getHead());
while (!queue.empty())
{
currentNode = queue.front();
queue.pop();
if (currentNode->dataIsPtr)
{
queue.push(currentNode->getDownPtr());
}
else
{
std::cout << currentNode->getDownData() << " ";
}
}
std::cout << std::endl;
}
void Destroy_cList(CNode*& cListHead)
{
int count = 0;
CNode* cNodePtr = cListHead;
while (cListHead != 0)
{
cListHead = cListHead->link;
delete cNodePtr;
cNodePtr = cListHead;
++count;
}
cout << "Dynamic memory for " << count << " CNodes freed"
<< endl;
}
void Destroy_pList(PNode*& pListHead)
{
int count = 0;
PNode* pNodePtr = pListHead;
while (pListHead != 0)
{
pListHead = pListHead->link;
Destroy_cList(pNodePtr->data);
delete pNodePtr;
pNodePtr = pListHead;
++count;
}
cout << "Dynamic memory for " << count << " PNodes freed"
<< endl;
}
// do depth-first traversal and print data
void ShowAll_DF(PNode* pListHead, ostream& outs)
{
while (pListHead != 0)
{
CNode* cListHead = pListHead->data;
while (cListHead != 0)
{
outs << cListHead->data << " ";
cListHead = cListHead->link;
}
pListHead = pListHead->link;
}
}
}
![>sh -c make -s
./nodes_LLOLL.cpp:15:18: error: use of undeclared identifier 'lloLLPtr'
queue.push(lloLLPtr->getHead());
./nodes_LLOLL.cpp:22:27: error: no member named 'dataIsPtr' in 'CS3358_SP2023_A5P2::CNode'
if (currentNode->dataIsptr)
NNNNNNNNNNN
./nodes_LLOLL.cpp:24:37: error: no member named 'getDownPtr' in 'CS3358_SP2023_A5P2::CNode'
queue.push(currentNode->get DownPtr());
NNNN
NNNN
./nodes_LLOLL.cpp:28:39: error: no member named 'getDownData' in 'CS3358_SP2023_A5P2::CNode'
std::cout <<< currentNode->getDownData() << " ";
NN
4 errors generated.
make: *** [Makefile:10: main] Error 1
exit status 2
Q Û](https://content.bartleby.com/qna-images/question/a3dbb91f-777d-47b2-aa94-40b3e17142a5/effc69ac-b923-47e0-8d19-7672712cece6/b7uz785_thumbnail.png)

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- IN C LANGUAGE True or False: You can not store multiple linked lists in a contiguous block of memory, even if there is space available for new nodes.arrow_forwardListQueue Node Node Node front= next next= next = nul1 rear = data "Thome" data "Abreu" data - "Jones" size - 3 The above is a queue of a waiting list. The ListQueue has a node (front) to record the address of the front element of a queue. It also has another node (rear) to record the address of the tail element of a queue. 4. How do you push a node with data, "Chu" to the above queue? front.next = new Node("Chu", front); a. b. front = new Node ("Chu", front) rear = new Node("Chu", rear) с. d. = new Node ("Chu", rear.next) rear Describe the reason of your choice. Your answer is (a, b, c, or d) Will the push action take time in 0(1) or 0(n)? Next Page Type here to searcharrow_forwardfrom PlaneQueue import PlaneQueue from PlaneNode import PlaneNode if __name__ == "__main__": plane_queue = PlaneQueue() # TODO: Read in arriving flight codes and whether a flight has landed. # Print the queue after every push() or pop() operation. If the user # entered "landed", print which flight has landed. Continue until -1 # is read.arrow_forward
- Please code in C language. Please use the starter code to help you solve the deleted node and the reverse list. Here is the starter code: #include <stdio.h> #include <ctype.h> #include <stdlib.h> #include <string.h> #include "linkedlist.h" // print an error message by an error number, and return // the function does not exit from the program // the function does not return a value void error_message(enum ErrorNumber errno) { char *messages[] = { "OK", "Memory allocaton failed.", "Deleting a node is not supported.", "The number is not on the list.", "Sorting is not supported.", "Reversing is not supported.", "Token is too long.", "A number should be specified after character d, a, or p.", "Token is not recognized.", "Invalid error number."}; if (errno < 0 || errno > ERR_END) errno = ERR_END; printf("linkedlist: %s\n", messages[errno]); } node *new_node(int v) { node *p =…arrow_forwardAn IntNode is a struct defined as: typedef struct IntNode struct { int dataVal; struct IntNode struct* nextNodePtr; } IntNode; Using this linked list diagram: Address of node: 2000 2800 1500 3600 3 65 34 76 head and this code snippet: IntNode * current; current = head; What is the value stored of current->nextNodePtr->nextNodePtr? 3 65 34 76 2000 2800 1500 3600 O O O O O O 0 0arrow_forwardIn this code: #include <iostream> #include <string> using namespace std;// Node struct to store data for each song in the playliststruct Node { string data; Node * next;};// Function to create a new node and return its addressNode * getNewNode(string song) { Node * newNode = new Node(); newNode -> data = song; newNode -> next = NULL; return newNode;}// Function to insert a new node at the head of the linked listvoid insertAtHead(Node ** head, string song) { Node * newNode = getNewNode(song); if ( * head == NULL) { * head = newNode; return;} newNode -> next = * head; * head = newNode;}// Function to insert a new node at the tail of the linked listvoid insertAtTail(Node ** head, string song) { Node * newNode = getNewNode(song); if ( * head == NULL) { * head = newNode; return; } Node * temp = * head; while (temp -> next != NULL) { temp = temp -> next; } temp -> next = newNode;}// Function to remove a node from the linked listvoid removeNode(Node ** head,…arrow_forward
- #ifndef EXTENDEDAVLNODE_H #define EXTENDEDAVLNODE_H #include "AVLNode.h" #include <iostream> class ExtendedAVLNode : public AVLNode { private: int subtreeKeyCount; public: ExtendedAVLNode(int nodeKey) : AVLNode(nodeKey) { subtreeKeyCount = 1; std::cout << "Node created with key: " << nodeKey << std::endl; } int GetSubtreeKeyCount() { return subtreeKeyCount; } void UpdateSubtreeKeyCount() { int leftCount = GetLeft() ? ((ExtendedAVLNode*)GetLeft())->GetSubtreeKeyCount() : 0; int rightCount = GetRight() ? ((ExtendedAVLNode*)GetRight())->GetSubtreeKeyCount() : 0; subtreeKeyCount = 1 + leftCount + rightCount; std::cout << "Updated subtreeKeyCount for node " << GetKey() << ": " << subtreeKeyCount << std::endl; } virtual void SetLeft(BSTNode* newLeftChild) override { std::cout << "Setting left child for node " << GetKey() <<…arrow_forwardAssume that nodeType struct was defined as below. struct nodeType Int Infor nodeType 1ink And nodeType n, F, L, P, "q Given initially the following linked list and new node n. F What are the steps to be executed to get the following linked list? O Flink-linker L-back-backen O linkep-link (p+1)-linken O 61)linken: nolinkep-lirk Om linkep-link polnken polnken n-inkep-link: O Rs not possible O Lbackbacken Fralinkelinknsarrow_forwardstruct insert_into_hash_table { // Function takes a constant Book as a parameter, inserts that book indexed by // the book's ISBN into a hash table, and returns nothing. void operator()(const Book& book) { ///// /// TO-DO (8) ||||. // Write the lines of code to insert the key (book's ISBN) and value // ("book") pair into "my_hash_table". /// END-TO-DO (8) // } std::unordered_map& my_hash_table; };arrow_forward
- #ifndef NODES_LLOLL_H#define NODES_LLOLL_H #include <iostream> // for ostream namespace CS3358_SP2023_A5P2{ // child node struct CNode { int data; CNode* link; }; // parent node struct PNode { CNode* data; PNode* link; }; // toolkit functions for LLoLL based on above node definitions void Destroy_cList(CNode*& cListHead); void Destroy_pList(PNode*& pListHead); void ShowAll_DF(PNode* pListHead, std::ostream& outs); void ShowAll_BF(PNode* pListHead, std::ostream& outs);} #endif #include "nodes_LLoLL.h"#include "cnPtrQueue.h"#include <iostream>using namespace std; namespace CS3358_SP2023_A5P2{ // do breadth-first (level) traversal and print data void ShowAll_BF(PNode* pListHead, ostream& outs) { cnPtrQueue queue; CNode* currentNode; queue.push(pListHead->data); while (!queue.empty()) { currentNode = queue.front(); queue.pop(); if…arrow_forward26. HomeExpert Q&AMy answers Student question Time to preview question: 00:09:49 int QUEUE_IS_EMPTY; int QUEUE_IS_FULL; void generator(){ Object thing = generate(); pthread_mutex_lock(&qlock); if (QUEUE_IS_FULL){ pthread_cond_wait(&queue_not_full); } enqueue(thing); pthread_cond_signal(&queue_not_full); pthread_mutex_unlock(&qlock); } void consumer(){ pthread_mutex_lock(&qlock); Object thing = dequeue(); consume(thing); while (QUEUE_IS_EMPTY){ pthread_cond_wait(&queue_not_empty); } pthread_cond_signal(&queue_not_full); pthread_mutex_unlock(&qlock); } This code generates objects in generator(), and consumes them in consumer(). Objects are placed into a queue to be consumed, and this queue has a limited size. enqueue() adds an item to the queue, and dequeue() removes one. An item may not be enqueued if the queue is full, or dequeued if the queue is empty. You may assume that QUEUE_IS_EMPTY and QUEUE_IS_FULL always correctly…arrow_forward* QueueArrayList.java This file implements QueueInterface.java This file has * only one ArrayList<T> type of attribute to hold queue elements. One default * constructor initializes the ArrayList<T> queue. An enqueue method receives an * object and place the object into the queue. The enqueue method does not throw * overflow exception. A dequeue method returns and removes an object from queue * front. The dequeue method will throw exception with message "Underflow" when * the queue is empty. A size method returns number of elements in the queue. A * toString method returns a String showing size and all elements in the queue. Please help me in javaarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
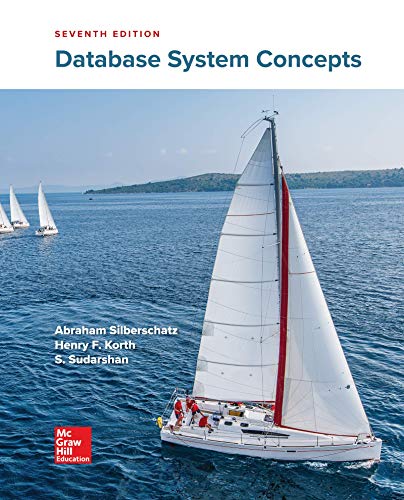
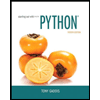
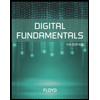
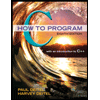
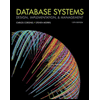
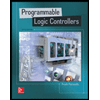