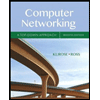
In this lab you will add code to the given java file to produce a Javax.swing GUI very much like the image
below in image
Use the ‘starter’ file, TestGUICounter.java
For this lab, read my pseudocode in the given java files FIRST!!
ANALYZE my code carefully.
Then start coding the solution.
I have given the solution to each lab instructor to help you during the time for your specific lab.
PLEASE FINISH THE JAVA CODE AS GUIDED BY THE Slashed LINES
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
public class TestGUICounter {
static JFrame frame_1;
static JButton start_val, add_1, minus_1, reset_1;
static JLabel label_1, label_num;
static JTextField text_1;
static JPanel panel_center, panel_south;
static int iCounter;
public static void main(String[] args) {
frame_1 = new JFrame();
frame_1.setLayout(new BorderLayout());
panel_center = new JPanel();
panel_south = new JPanel();
panel_center.setLayout(new FlowLayout());
panel_south.setLayout(new FlowLayout());
start_val = new JButton("Start Counter");
add_1 = new JButton("Add 1");
minus_1 = new JButton("Delete 1");
reset_1 = new JButton("Reset");
label_1 = new JLabel("Value is: ");
// setup all buttons to use the "ButtonListener()" class for ActionEvents
// Put the JtextField, and the two JLabel in the 'panel_center'
// and the buttons in the 'panel_south' panels.
// add the two panels to the 'frame_1' in the proper
// area (CENTER, SOUTH, NORTH, EAST, WEST)
frame_1.setSize(450, 200);
frame_1.setVisible(true);
frame_1.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
private static class ButtonListener implements ActionListener {
public void actionPerformed(ActionEvent e) {
// write code here to test which of the three buttons
// have been clicked using 'e.getSource().equals...'
// very similar to the demonstration I did in class lecture
// First, when 'start_val' clicked, then get the value from the 'text_1'
// text box (user 'getText()' method) and set the 'label_num' and the
// iCounter to be that value. Next, in the if 'add_1' clicked, then
// add 1 to the 'iCounter' and update label: 'label_num' and if
// the 'minus_1' is clicked, delete 1 from iCounter and update
// the 'label_num' Then, if 'reset_1' is clicked, set things blank and back to zero.
// HINT: when you get the value from a JTextField, it is a string, so
// you have to convert it to an integer to give the value to 'iCounter'
// and to convert an integer to a string, use 'Integer.parseInt(string variable)'
//
}
}
}
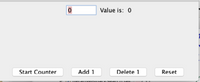

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 3 images

- In JAVAFX. Any and all help is appreciated, please also show the results with your code. Please see the attached image.arrow_forwardComputer Science for Java Write a GUI program in the language(Java) you have chosen for your SOS project. The GUI of your programmust include text, lines, a check box, and radio buttons. While you are recommended to consider theGUI for the SOS game board, it is not required. In this assignment, any GUI program of your own workis acceptable.Attach here (1) the screenshot of your program execution and (2) the source code of your program.arrow_forwardPlease help me with thisarrow_forward
- An interesting GUI component in Java is the Slider. Indeed, sliders allow a user to specify a numeric value within a bounded range.Write a program in Java using sliders to simulate the measurement of the temperature in Celsius using a digital thermometer. The following must be achieved: Design the thermometer using an appropriate shape and include the slider inside the shape with tick marks. Use proper layout managers and containers. The thermometer will measure temperatures between -20 oC and 110 oC upon sliding the GUI slider component. The temperature will be displayed in a text field or label. Using another label, a message will be displayed when the temperature reaches a certain value. For example, at -20 oC, the message “It’s ice cold” is displayed, at +10 oC, the message “It’s quite cold” is displayed, at +35 oC, the message “It’s hot” is displayed, etc.arrow_forwardCreate a JavaFX application that displays a similar picture. You can use your own color palette. You do not have to match sizes exactly. You are free to add more details, but do not forget to print your name at the lower right angle.arrow_forwardthis is my code, but after automatically check it, it shows smt went wrong(image one) image two is the spec for nextTweet and below is my code, since it's the system automatically check the code, I dont really have a textcases. plz debug it and fix it. show the screenshot of the code when u finish. thanks Below is my whole code for TweetBot import java.util.*;import java.io.*; public class TweetBot { private List<String> tweets; private int index; public TweetBot(List<String> tweets){ if(tweets.size() < 1){ throw new IllegalArgumentException("need contain at least one tweet!"); } this.tweets = new ArrayList<>(tweets); } public int numTweets(){ return tweets.size(); } public void addTweet(String tweet) { tweets.add(tweet); if (tweets.size() == 1) { // if the tweet was the first one added, set the index to 0 index = tweets.size() - 1; } } public…arrow_forward
- This is for the game brick breaker use Java to code what's posted down below.arrow_forwardChapter 18 Problem 38.PE Textbook: Introduction to Java programming and datastructure. 11th Edition Y. Daniel Liang Publisher: PEARSON ISBN: 9780134670942 This code is taken from your website does not work. package e38; import javafx.application.Application;import javafx.event.ActionEvent;import javafx.event.EventHandler;import javafx.scene.Scene;import javafx.scene.control.Button; import javafx.stage.Stage;import javafx.geometry.Pos;import javafx.scene.control.Label;import javafx.scene.control.TextField;import javafx.scene.control.Textfield;import javafx.scene.layout.BorderPane;import javafx.scene.layout.HBox;import javafx.scene.layout.Pane;import javafx.scene.shape.Line;import javafx.stage.Stage; public class E38 extends Application { @Override public void start(Stage primaryStage) { // Create a pane TreePane tp = new TreePane(); // Create a textfield TextField tfOrder = new TextField(); // Set the Depth…arrow_forwardUsing JavaFX with Eclipse or Netbeans, please explain using a main class, controller class, and a FXML... Devise a JavaFX GUI with an internal controller class to maintain an array of arbitrary size. Use a starting default of size 20 and populate it with random data (numbers) initialized into the array. The GUI should be such that users can select and perform the actions listed below. Write methods and select appropriate GUI mechanisms to do the following: 1. Display the contents of the array2. Add a, or a group of numbers to the array at any position.3. Delete a number from the array at any position.4. Sort the array and display the original and sorted forms.5. Display the size of the array.6. Search for a number and its occurrences in the array and flag that number’s position(s) by highlighting it in the display.arrow_forward
- In java pls!!!! thank you!arrow_forwardCan you show me where and how to add an image folder (Create a pane to hold the images views) with gif images on this code, to hold image views. code is below import javafx.application.Application;import javafx.scene.Scene;import javafx.scene.image.Image;import javafx.scene.image.ImageView;import javafx.scene.layout.GridPane;import javafx.stage.Stage; public class ex1401 extends Application {@Override public void start(Stage primaryStage) { /** pane to hold four images */GridPane p= new GridPane();p.add(new ImageView(new Image("image/uk.gif")), 0, 0);p.add(new ImageView(new Image("image/ca.gif")), 1, 0);p.add(new ImageView(new Image("image/china.gif")), 0, 1);p.add(new ImageView(new Image("image/us.gif")), 1, 1); Scene s= new Scene(p);primaryStage.setTitle("Exercise_14_01");primaryStage.setScene(s);primaryStage.show(); } }arrow_forwardPlease fix these in the above splution, it is not fully correct. "You're invited" needs to be formatted correctly. With a larger font, using BS classes only. Use Bootstrap classes to make a circular image with a border - around the image only. "Don't tell the cats" color should be set with BS classes.arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
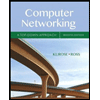
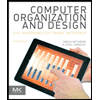
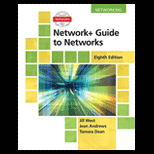
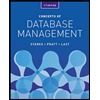
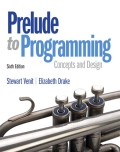
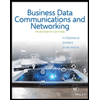