In this assignment, you are required to implement an electronic programming quiz system. User can create questions and preview the quiz.
Description
In this assignment, you are required to implement an electronic programming quiz system. User can create
questions and preview the quiz.
Your Task
You are asked to write a Java program for the programming quiz system. There are two types of questions:
Multiple Choice Question and Ture/False Question. User can create questions using the system; and
preview the quiz, which display all questions in the system one by one. During the preview, the user can
attempt the quiz by entering his/her answers to questions. The system will then immediately check the
answer and calculate. After attempting all questions, the total score will be displayed. A sample run of the
program is shown as below (Green text refers to user input):
Please choose (c)reate a question, (p)review or (e)xit >> c
Enter the type of question (MC or TF) >> MC
Enter the question text >> Each primitive type in Java has a corresponding
class contained in the java.lang package. These classes are called ____
classes.
How many options? 4
Enter Option A (Start with * for correct answer) >> case
Enter Option B (Start with * for correct answer) >> primitive
Enter Option C (Start with * for correct answer) >> *type-wrapper
Enter Option D (Start with * for correct answer) >> show
How many points? 3
Please choose (c)reate a question, (p)review or (e)xit >> c
Enter the type of question (MC or TF) >> MC
Enter the question text >> A(n) ____ variable is known only within the
boundaries of the method.
How many options? 5
Enter Option A (Start with * for correct answer) >> method
Enter Option B (Start with * for correct answer) >> *local
Enter Option C (Start with * for correct answer) >> double
Enter Option D (Start with * for correct answer) >> instance
Enter Option E (Start with * for correct answer) >> global
How many points? 2
Please choose (c)reate a question, (p)review or (e)xit >> c
Enter the type of question (MC or TF) >> TF
Enter the question text >> Java is a free-form programming language.
Answer is True or False? True
How many points? 1
Please choose (c)reate a question, (p)review or (e)xit >> p
Each primitive type in Java has a corresponding class contained in the
java.lang package. These classes are called ____ classes. (3.0 Points)
A: case
B: primitive
C: type-wrapper
D: show
Enter your choice >> A
You are wrong. The correct answer is C.
A(n) ____ variable is known only within the boundaries of the method. (2.0
Points)
A: method
B: local
C: double
D: instance
E: global
Enter your choice >> B
You are correct!
Java is a free-form programming language. (1.0 Points)
True(T) or False(F) >> F
You are wrong. The correct answer is true.
The quiz ends. Your score is 2.0.
Please choose (c)reate a question, (p)review or (e)xit >> c
Enter the type of question (MC or TF) >> MC
Enter the question text >> A(n) ____ constructor is one that requires no
arguments.
How many options? 3
Enter Option A (Start with * for correct answer) >> class
Enter Option B (Start with * for correct answer) >> *default
Enter Option C (Start with * for correct answer) >> explicit
How many points? 2
Please choose (c)reate a question, (p)review or (e)xit >> c
Enter the type of question (MC or TF) >> TF
Enter the question text >> Javascript and Java are the same.
Answer is True or False? False
How many points? 0.5
Please choose (c)reate a question, (p)review or (e)xit >> p
Each primitive type in Java has a corresponding class contained in the
java.lang package. These classes are called ____ classes. (3.0 Points)
A: case
B: primitive
C: type-wrapper
D: show
Enter your choice >> C
You are correct!
A(n) ____ variable is known only within the boundaries of the method. (2.0
Points)
A: method
B: local
C: double
D: instance
E: global
Enter your choice >> B
You are correct!
Java is a free-form programming language. (1.0 Points)
True(T) or False(F) >> T
You are correct!
A(n) ____ constructor is one that requires no arguments. (2.0 Points)
A: class
B: default
C: explicit
Enter your choice >> C
You are wrong. The correct answer is B.
Javascript and Java are the same. (0.5 Points)
True(T) or False(F) >> T
You are wrong. The correct answer is false.
The quiz ends. Your score is 6.0.
Please choose (c)reate a question, (p)review or (e)xit >> e
Goodbye!
Question Class:
- This class represents the generic form of question. It contains the question text (qText) and
the point of a question (point).
- The grade method, which is an abstract method, has a parameter, answer (String). It returns
the points of the question if the answer is correct; zero otherwise.
- The getCorrectAnswer method, which is an abstract method, has no parameter. It returns a
String representing the correct answer to the question.
MCQuestion Class
- It is a subclass of Question.
- It represents a multiple-choice question. A multiple-choice question may have 3-5 options.
- Each element of the instance variable options refers to an option in this question.
- The instance variable answer is a single-character string. It saves the correct answer (“A”,
“B”, “C”, “D” or “E”) to this question.



Step by step
Solved in 2 steps

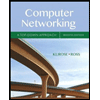
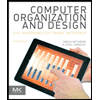
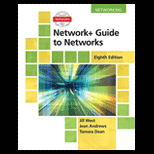
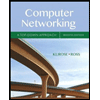
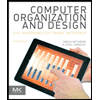
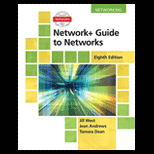
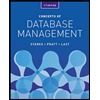
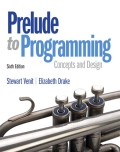
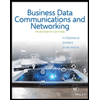