The TidBit Computer Store (Chapter 3, Project 10) has a credit plan for computer purchases. Inputs are the annual interest rate and the purchase price. Monthly payments are 5% of the listed purchase price, minus the down payment, which must be 10% of the purchase price. Write a GUI-based program that displays labeled fields for the inputs and a text area for the output. The program should display a table, with appropriate headers, of a payment schedule for the lifetime of the loan. Each row of the table should contain the following items: The month number (beginning with 1) The current total balance owed The interest owed for that month The amount of principal owed for that month The payment for that month The balance remaining after payment The amount of interest for a month is equal to ((balance * rate) / 12) / 100. The amount of principal for a month is equal to the monthly payment minus the interest owed. Your program should include separate classes for the model and the view. The model should include a method that expects the two inputs as arguments and returns a formatted string for output by the GUI.
The TidBit Computer Store (Chapter 3, Project 10) has a credit plan for computer purchases. Inputs are the annual interest rate and the purchase price. Monthly payments are 5% of the listed purchase price, minus the down payment, which must be 10% of the purchase price.
Write a GUI-based program that displays labeled fields for the inputs and a text area for the output. The program should display a table, with appropriate headers, of a payment schedule for the lifetime of the loan. Each row of the table should contain the following items:
- The month number (beginning with 1)
- The current total balance owed
- The interest owed for that month
- The amount of principal owed for that month
- The payment for that month
- The balance remaining after payment
The amount of interest for a month is equal to ((balance * rate) / 12) / 100. The amount of principal for a month is equal to the monthly payment minus the interest owed.
Your program should include separate classes for the model and the view. The model should include a method that expects the two inputs as arguments and returns a formatted string for output by the GUI.



Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

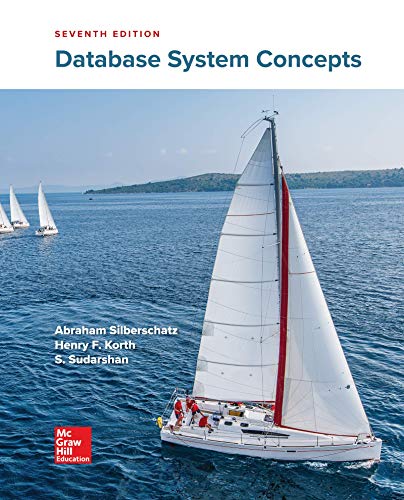
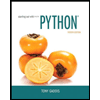
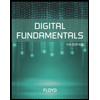
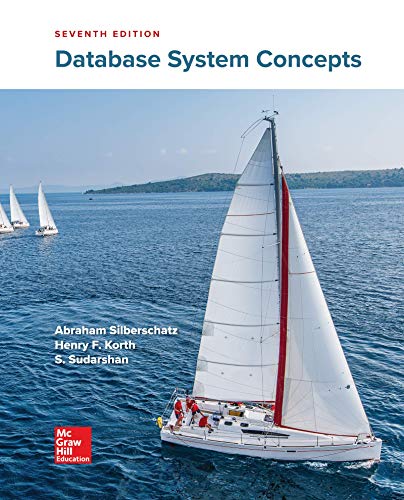
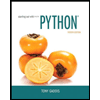
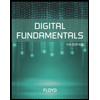
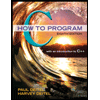
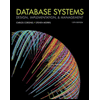
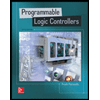