. Dummy GUI Application by Codechum Admin A GUI Application is an application that has a user interface that the user can interact with. For this program, we will be simulating this behavior. First, implement another class called Checkbox which implements the Clickable interface which has only one method: public void click(). The Checkbox will have the following properties: private boolean isChecked (defaults to false upon the creation of object) private String text Additionally, it should have the following methods: the implementation of the click() method If the isChecked is currently false, this will set the isChecked to true and will then print the message "Checkbox is checked". If it is currently true, this will set the isChecked to false and will then print the message "Checkbox is unchecked". Note that the messages to be printed should have also print a new line at the end. an override of the toString() method which returns the message: "Checkbox ({text} - Clicked = {true|false})" Examples: Checkbox (Mango - Checked = true) Checkbox (Orange - Checked = false) Then, create the AbstractButton class which is an abstract class and has the following properties: protected String title protected int width protected int height protected boolean isClicked (defaults to false upon creation of object) Additionally, it should have the following methods: getter and setter methods for title, width, and height a constructor with the following signature: public AbstractButton(String title, int width, int height) The AbstractButton class implements the Clickable interface and the Resizable interface which has two methods in order: public void resize(int newWidth, int newHeight) public void resize(int multiplier) Then, implement the NormalButton class which extends from the AbstractButton class and has the following methods: a constructor with the following signature: public NormalButton(title, width, height) the implementation of the click() method This will print the message "Button is clicked", sets the isClicked to true, prints the message "Button is not clicked anymore", and sets the isClicked to false the implementation of the first resize() method which accepts two parameters: This simply sets the new width and height of the button the implementation of the second resize() method which accepts one parameter only: This will multiply the width and height of the button by the multiplier passed. an override of the toString() method which returns the message: "NormalButton (({width}px, {height}px) - Clicked = {true|false})" Examples: NormalButton ((25px, 10px) - Clicked = true) NormalButton ((100px, 100px) - Clicked = false) In the main(), ask the user to input the title, width, and height of the NormalButton and then use these values to instantiate a NormalButton object. Then, ask the user to input the text of the Checkbox and use this to create a Checkbox object. Make sure you test all the methods of these objects. Once done, comment out all your test method calls, call the Tester.test() function call in the main() and pass the NormalButton as its first argument and Checkbox as its second. Sample Output 1 NormalButton Enter title: Click Me Enter width: 5 Enter height: 7 Checkbox Enter text: Mango Enter code: 1 SUCCESS Sample Output 2 NormalButton Enter title: Submit Enter width: 5 Enter height: 4 Checkbox Enter text: Happy Enter code: 2 SUCCESS
1. Dummy GUI Application
by Codechum Admin
A GUI Application is an application that has a user interface that the user can interact with. For this program, we will be simulating this behavior.
First, implement another class called Checkbox which implements the Clickable interface which has only one method: public void click(). The Checkbox will have the following properties:
- private boolean isChecked (defaults to false upon the creation of object)
- private String text
Additionally, it should have the following methods:
- the implementation of the click() method
- If the isChecked is currently false, this will set the isChecked to true and will then print the message "Checkbox is checked". If it is currently true, this will set the isChecked to false and will then print the message "Checkbox is unchecked". Note that the messages to be printed should have also print a new line at the end.
-
an override of the toString() method which returns the message: "Checkbox ({text} - Clicked = {true|false})"
-
Examples:
- Checkbox (Mango - Checked = true)
- Checkbox (Orange - Checked = false)
-
Then, create the AbstractButton class which is an abstract class and has the following properties:
- protected String title
- protected int width
- protected int height
- protected boolean isClicked (defaults to false upon creation of object)
Additionally, it should have the following methods:
- getter and setter methods for title, width, and height
- a constructor with the following signature: public AbstractButton(String title, int width, int height)
The AbstractButton class implements the Clickable interface and the Resizable interface which has two methods in order:
- public void resize(int newWidth, int newHeight)
- public void resize(int multiplier)
Then, implement the NormalButton class which extends from the AbstractButton class and has the following methods:
- a constructor with the following signature: public NormalButton(title, width, height)
- the implementation of the click() method
- This will print the message "Button is clicked", sets the isClicked to true, prints the message "Button is not clicked anymore", and sets the isClicked to false
- the implementation of the first resize() method which accepts two parameters:
- This simply sets the new width and height of the button
- the implementation of the second resize() method which accepts one parameter only:
- This will multiply the width and height of the button by the multiplier passed.
-
an override of the toString() method which returns the message: "NormalButton (({width}px, {height}px) - Clicked = {true|false})"
-
Examples:
- NormalButton ((25px, 10px) - Clicked = true)
- NormalButton ((100px, 100px) - Clicked = false)
-
In the main(), ask the user to input the title, width, and height of the NormalButton and then use these values to instantiate a NormalButton object. Then, ask the user to input the text of the Checkbox and use this to create a Checkbox object.
Make sure you test all the methods of these objects. Once done, comment out all your test method calls, call the Tester.test() function call in the main() and pass the NormalButton as its first argument and Checkbox as its second.
Sample Output 1
Sample Output 2

Trending now
This is a popular solution!
Step by step
Solved in 4 steps

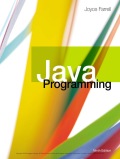
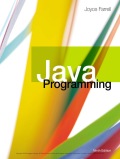