In blue J add a no argument constructor for the constructor of the code below: public class Book { private String author; private String title; private int pages; private String publisher; //String for phone number because it is too big a number to store in int or long private String phone; //constructor public Book () { } public Book(String author, String title, int pages, String publisher, String phone) { this.author = author; this.title = title; this.pages = pages; this.publisher = publisher; this.phone = phone; } //getters and setters public String getAuthor() { return author; } public void setAuthor(String author) { this.author = author; } public String getTitle() { return title; } public void setTitle(String title) { this.title = title; } public int getPages() { return pages; } public void setPages(int pages) { this.pages = pages; } public String getPublisher() { return publisher; } public void setPublisher(String publisher) { this.publisher = publisher; } public String getPhone() { return phone; } public void setPhone(String phone) { this.phone = phone; } }
In blue J add a no argument constructor for the constructor of the code below:
public class Book
{
private String author;
private String title;
private int pages;
private String publisher;
//String for phone number because it is too big a number to store in int or long
private String phone;
//constructor
public Book ()
{
}
public Book(String author, String title, int pages, String publisher, String phone)
{
this.author = author;
this.title = title;
this.pages = pages;
this.publisher = publisher;
this.phone = phone;
}
//getters and setters
public String getAuthor()
{
return author;
}
public void setAuthor(String author)
{
this.author = author;
}
public String getTitle()
{
return title;
}
public void setTitle(String title)
{
this.title = title;
}
public int getPages()
{
return pages;
}
public void setPages(int pages)
{
this.pages = pages;
}
public String getPublisher()
{
return publisher;
}
public void setPublisher(String publisher)
{
this.publisher = publisher;
}
public String getPhone()
{
return phone;
}
public void setPhone(String phone)
{
this.phone = phone;
}
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 2 images

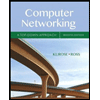
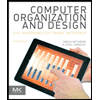
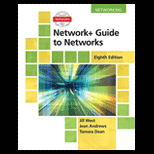
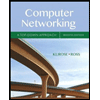
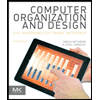
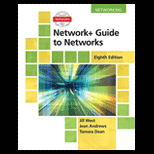
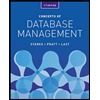
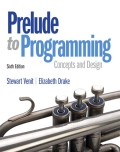
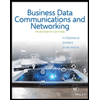