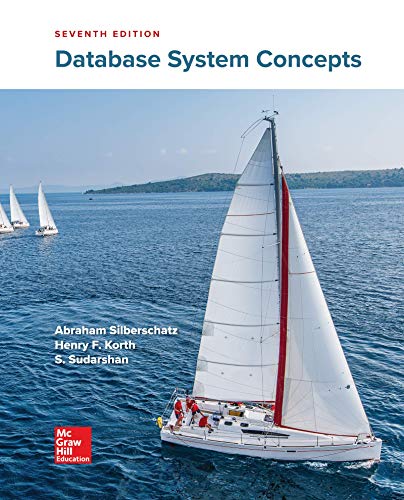
Concept explainers
Consider the following class definition:
class circle { public: void print() const;
void setRadius(double);
void setCenter(double, double);
void getCenter(double&, double&);
double getRadius();
double area();
circle();
circle(double, double, double);
double, double);
private: double xCoordinate;
double yCoordinate;
double radius; }
class cylinder: public circle { public: void print() const;
void setHeight(double);
double getHeight();
double volume();
double area();
cylinder();
cylinder(double, double, private: double height; }
Suppose that you have the declaration: cylinder newCylinder;
Write the definitions of the member functions of the classes circle and cylinder. Identify the member functions of the class cylinder that overrides the member functions of the class circle

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps

- Given the following class definition: class employee{public: employee(); employee(string, int, double); employee(int, double); employee(string); void setData(string, int, double); void print() const; void updatePay(double); int getNumOfServiceYears() const; double getPay() const;private: string name; int numOfServiceYears; double pay;}; Create a class parttime derived from the above class that includes private members payRate and hoursWorked of type double along with a constructor and the definition of a print function that prints out all information from the base and derived class. Provide a test program that would demonstrate the working parttime class. Note: You can assume all code is stored in a single file.arrow_forwardClass Phone{ bool wired() string ageOfInnovation() } Class CellPhone extends Phone{ bool wired(){ return false; } string ageOfInnovation(){ return "1970's" } int thickness(){ return 0; } } Class SmartPhone extends CellPhone{ bool wired(){ return false; } string ageOfInnovation(){ return "1990's" } string popularlyCalled(){ return "mobile" } string mainFeature(){ return "Internet Capability"; } }arrow_forwardpublic class Plant { protected String plantName; protected String plantCost; public void setPlantName(String userPlantName) { plantName = userPlantName; } public String getPlantName() { return plantName; } public void setPlantCost(String userPlantCost) { plantCost = userPlantCost; } public String getPlantCost() { return plantCost; } public void printInfo() { System.out.println(" Plant name: " + plantName); System.out.println(" Cost: " + plantCost); }} public class Flower extends Plant { private boolean isAnnual; private String colorOfFlowers; public void setPlantType(boolean userIsAnnual) { isAnnual = userIsAnnual; } public boolean getPlantType(){ return isAnnual; } public void setColorOfFlowers(String userColorOfFlowers) { colorOfFlowers = userColorOfFlowers; } public String getColorOfFlowers(){ return colorOfFlowers; } @Override public void printInfo(){…arrow_forward
- Public classTestMain { public static void main(String [ ] args) { Car myCar1, myCar2; Electric Car myElec1, myElec2; myCar1 = new Car( ); myCar2 = new Car("Ford", 1200, "Green"); myElec1 = new ElectricCar( ); myElec2 = new ElectricCar(15); } }arrow_forwardPublic Class SavingsAccount { float interest; float FixedDeposit; SeniorAccount(float interest, float FixedDeposit) { this. interest = interest; this.fixedDeposit= FixedDeposit; } float calculateInterest(); { System.out.println(“Calculating Savings Account Interest”); return(FixedDeposit*interest/100); } } Public Class SeniorAccount extends SavingAccount { float seniorInterest; SeniorAccount(float interest, float FixedDeposit) { this.seniorInterest=interest; super(interest, FixedDeposit) } float calculateInterest() { System.out.println(“Calculating Savings Account Interest”); return(FixedDeposit*seniorinterest/10); } } Public static void main(String args[]) { SavingsAccount saving = new SavingsAccount(6,100000); System.out.println(saving.calculateinterest()); SeniorAccount senior=new seniorAccount(10,100000); System.out.println(Senior.calculateInterest()); } Correct the syntax and logical errorarrow_forward2arrow_forward
- [java program] public class ScenarioAnalysis { // Instance variables privateVehicle[] vehicles; // all vehicless being analyzed privatedoublegasPrice; // price of one gallon of gas in dollars privatedoubleelectricityPrice; // price of 1 kWh in cents of a dollar, c$/kWh publicScenarioAnalysis ( doublegasPrice, doubleelectricityPrice ) { this.gasPrice = gasPrice; this.electricityPrice = electricityPrice; } /* * Updates the price of gas * Call computeCO2EmissionsAndCost() whenever there is an update on gas prices */ publicvoidsetGasPrice ( doublegasPrice ) { this.gasPrice = gasPrice; computeCO2EmissionsAndCost(); } /* * Returns the gas price */ publicdoublegetGasPrice () { returngasPrice; } /* * Updates the price of electricity * Call computeCO2EmissionsAndCost() whenever there is an update on electricity prices */ publicvoidsetElectricityPrice ( doubleelectricityPrice ) { this.electricityPrice = electricityPrice; } /* * Returns electricity price */ publicdoublegetElectricityPrice…arrow_forwardBased on Figure 3, create a class named Child that inherits the Father class. Declare an instance name location (String) for class Child. [3 marks]arrow_forwardUML for the following Code: public class ProductOrder { private int numBook = 0; private int numCD = 0; private int numDVD = 0; public ProductOrder(int numBook, int numCD, int numDVD){ this.numBook = numBook; this.numCD = numCD; this.numDVD = numDVD; } public int getBook(){ return numBook; } public void setBook(int numBook){ this.numBook = numBook; } public int getCD(){ return numCD; } public void setCD(int numCD){ this.numCD = numCD; } public int getDVD(){ return numDVD; } public void setDVD(int numDVD){ this.numDVD = numDVD; } public int getProductTotal(){ return getBook() + getCD() + getDVD(); } }arrow_forward
- public class LabProgram { public static void main(String args[]) { Course course = new Course(); String first; // first name String last; // last name double gpa; // grade point average first = "Henry"; last = "Cabot"; gpa = 3.5; course.addStudent(new Student(first, last, gpa)); // Add 1st student first = "Brenda"; last = "Stern"; gpa = 2.0; course.addStudent(new Student(first, last, gpa)); // Add 2nd student first = "Jane"; last = "Flynn"; gpa = 3.9; course.addStudent(new Student(first, last, gpa)); // Add 3rd student first = "Lynda"; last = "Robison"; gpa = 3.2; course.addStudent(new Student(first, last, gpa)); // Add 4th student course.printRoster(); } } // Class representing a student public class Student { private String first; // first name private String last; // last name private double gpa; // grade point average…arrow_forwardQuestion 13 What is outpout? public class Vehicle { public void drive(){ System.out.println("Driving vehicle"); } } public class Plane extends Vehicle { @Override public void drive(){ System.out.println("Flying plane"); } public static void main(String args[]) { Vehicle myVehicle= = new Plane(); myVehicle.drive(); } Driving vehicle syntax error Flying plane Driving vehicle Flying plane }arrow_forwardpublic class StairViewer public static void main(String(] args) // Step 2: Create a rectangle and draw it as the // top step of the stair // location: (20, 10) // size: (20, 20) 18 // Step 3: Create a rectangle and draw it as the 19 // middle step of the stair 20 // location: below the top step, aligned on left // size: (40, 20) 21 22 23 24 // Step 4: Create a rectangle and draw it as the 25 // bottom step of the stair 26 // location: below the middle step, aligned on left // size: (60, 20) 27 28 29 30 } 31 32arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
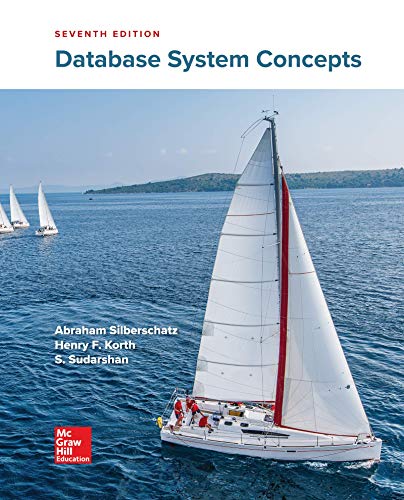
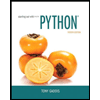
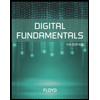
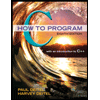
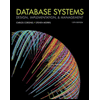
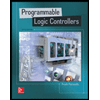