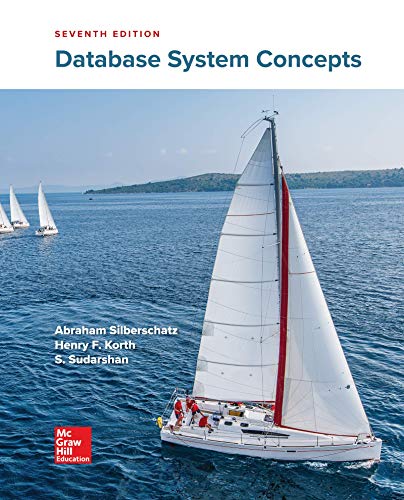
Here is a statement:
class temporary
{
public:
void set(string, double, double);
void print();
double manipulate();
void get(string&, double&, double&);
void setDescription(string);
void setFirst(double);
void setSecond(double);
string getDescription() const;
double getFirst()const;
double getSecond()const;
temporary(string = "", double = 0.0, double = 0.0);
private:
string description;
double first;
double second;
};
How can I write a definition of the member function manipulate that returns a decimal number as follows: If the value of description is "rectangle", it returns first * second; if the value of description is "circle", it returns the area of the circle with radius first; if the value of description is "sphere", it returns the volume of the sphere with radius first; if the value of description is "cylinder", it returns the volume of the cylinder with radius first and height second; otherwise, it returns the value -1.

Algorithm: temporary::manipulate()
Input:
- None
Output:
- Result of the manipulation based on the description:
- If description is "rectangle", return the area of the rectangle (first * second).
- If description is "circle", return the area of the circle with radius first.
- If description is "sphere", return the volume of the sphere with radius first.
- If description is "cylinder", return the volume of the cylinder with radius first and height second.
- Otherwise, return -1 to indicate an invalid description.
Steps:
1. Check the value of the 'description' member variable:
1.1 If 'description' is equal to "rectangle", do the following:
1.1.1 Calculate the area of a rectangle:
result = first * second
1.1.2 Return 'result'
1.2 If 'description' is equal to "circle", do the following:
1.2.1 Calculate the area of a circle:
result = π * first * first (where π is a constant, approximately 3.14159265359)
1.2.2 Return 'result'
1.3 If 'description' is equal to "sphere", do the following:
1.3.1 Calculate the volume of a sphere:
result = (4.0 / 3.0) * π * first * first * first
1.3.2 Return 'result'
1.4 If 'description' is equal to "cylinder", do the following:
1.4.1 Calculate the volume of a cylinder:
result = π * first * first * second
1.4.2 Return 'result'
1.5 Otherwise (for an invalid 'description'):
1.5.1 Return -1 to indicate an invalid description.
Step by stepSolved in 4 steps with 3 images

- The provided file has syntax and/or logical errors. Determine the problem and fix the program.arrow_forwardPython language Write a initializer method for the class Test. It has 2 parameters/field variables: correct and totalarrow_forwardWhat two steps are typically required for creating a reference type object?arrow_forward
- Describe the process through which a method accepts parameters of primitive and reference types.arrow_forwardfunctions return the value of a data member. A. Accessor OB. Member C. Mutator OD. Utilityarrow_forwardWrite in c language please: write a statement that calls the function IncreaseItemQty with parameters computerInfo and addStock. Assign computerInfo with the returned value. #include <stdio.h> typedef struct ProductInfo_struct { char itemName[50]; int itemQty;} ProductInfo; ProductInfo IncreaseItemQty(ProductInfo productToStock, int increaseValue) { productToStock.itemQty = productToStock.itemQty + increaseValue; return productToStock;} int main(void) { ProductInfo computerInfo; int addStock; scanf("%s", computerInfo.itemName); scanf("%d", &computerInfo.itemQty); scanf("%d", &addStock); /* Your code goes here */ printf("Name: %s, stock: %d\n", computerInfo.itemName, computerInfo.itemQty); return 0;} thank you in advancearrow_forward
- class Container {private int val1=1;private void printValue(){final int val2=2;class Containee{public void f(){System.out.println(String.format("Values are: %d and %d."),val1);}}}public static void main(String[] args) {(new Container()).printValue();}} a.The above does not compile because the method f() requires an instance of class Container b.The above does not compile because the class definition in done within the body of the function c.The above code complies with no errors, but does not produce any ouput d.The above code does not compile because the methodf() cannot access the variable val1arrow_forwardWhich function members are mutators?arrow_forwardSolve the picture.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
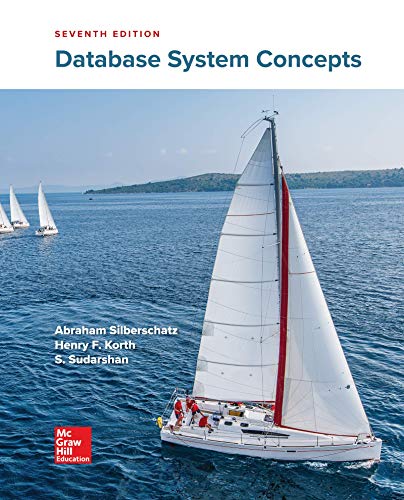
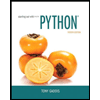
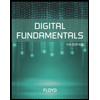
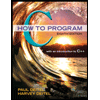
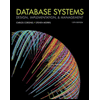
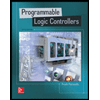