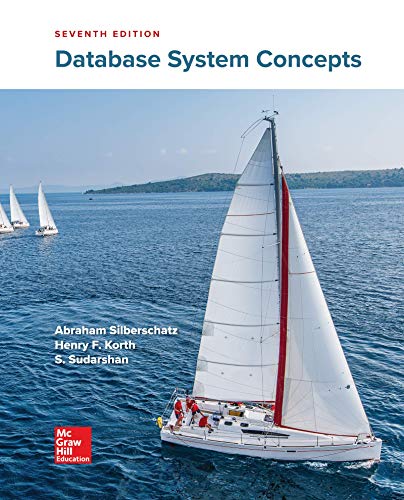
Concept explainers
Implement a program that creates an unsorted list by using a linked list implemented by yourself. NOT allowed to use LinkedList class or any other classes that offers list functions. It is REQUIRED to use an ItemType class and a NodeType struct to solve this. Use C++!
The “data.txt” file has three lines of data
100, 110, 120, 130, 140, 150, 160
100, 130, 160
1@0, 2@3, 3@END
You need to
- create an empty unsorted list
- add the numbers from the first line to list using putItem() function.
- Then print all the current keys to command line in one line using printAll().
- delete the numbers given by the second line in the list by using deleteItem() function.
- Then print all the current keys to command line in one line using printAll()..
- putItem () the numbers in the third line of the data file to the corresponding location in The list. For example, 1@0 means adding number 1 at position 0 of the list.
Then print all the current keys to command line in one line using printAll()..
Implement the following functions by yourself and use them
- putItem(parameter one is the object to be added): append the object at the end of the list.
- putItem(parameter one is the object to be added, parameter two is the position starting From 0): insert the item at the position.
- deleteItem(parameter is the key which is an integer): remove the item
- getItem(parameter is an index value which starts from 0) returns the item object reference
- printAll(parameter is the pointer that points to the beginning of the list), print all keys in Order in the list.
PLEASE make sure the test data file matches exactly how it is displayed up there so I can also run it with the same type of data file! Please show output, and please post solution WITHOUT linked list class! Example output is added in image.
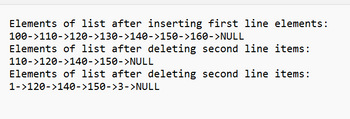

In this question we have to write a C++ program for the linked list which need to be implemented from the basic structure and we will implement the code form the above description
Let's code, hope this helps I tried with all implementations if you still find some queries you may utilize the threaded question.
Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

- Create a program that removes duplicate integer values from a list. For example if a list has these valuesList = [5, 7, 8, 10, 8, 5, 12, 15, 12]. After applying the removal function, you should have List = [5, 7, 8,10, 12, 15]. In your case you will get the numbers of list from the user.arrow_forwardYou are going to implement a program that creates an unsorted list by using a linked list implemented by yourself. NOT allowed to use LinkedList class or any other classes that offers list functions. It is REQUIRED to use an ItemType class and a NodeType struct to solve this homework. The “data.txt” file has three lines of data 100, 110, 120, 130, 140, 150, 160 100, 130, 160 1@0, 2@3, 3@END You need to 1. create an empty unsorted list 2. add the numbers from the first line to list using putItem() function. Then print all the current keys to command line in one line using printAll(). 3. delete the numbers given by the second line in the list by using deleteItem() function. Then print all the current keys to command line in one line using printAll().. 4. putItem () the numbers in the third line of the data file to the corresponding location in the list. For example, 1@0 means adding number 1 at position 0 of the list. Then print all the current keys to command line in one…arrow_forwardCreate a program in C++ using a character linked list. It will insert the letters into the list alphabetically. Ask the user to enter a word. Then, enter the word into the list. Then, print out what is in the list, and clear it out. example: enters: apple prints: aelpp You can do this by writing your own linked list (extra credit), or using the STL list class. -- if you are using the STL list class, do not use the “sort” function.arrow_forward
- Write a line (or lines) of code that uses a list that has been previously defined, named word_list, along with a string value entered by the user beforehand, named find, to print out the percent of the occurrence of that word in the list. As an example, if your word_list looked like this: ['the', 'word', 'I', 'am', 'looking', 'for', 'is', 'called', 'my', 'word'] And find was the string 'word' The Example Output would look like this: 20.00% of the list is word Otherwise, if your word_list looked like this: ['another', 'word', 'that', 'is', 'being', 'found', 'is', 'terracotta'] And find was the string 'looking' That Example Output would look like this: 0.00% of the list is lookingarrow_forwardWrite a program in three parts. The first part should create a linked list of 26 nodes. The second part should fill the list with the letters of the alphabet. The third part should print the contents of the linked list. NOT USE malloc, only use <iostream>. Do not use global variables. The use of global constants c++arrow_forwardIn C++arrow_forward
- Suppose names is an ABList containing 10 elements. The call names.add(0, "George") results in: A. an exception being thrown. B. a 10-element list with "George" as the first element. C. an 11-element list with "George" as the first element. D. a single element list containing "George". E. None of these is correct.arrow_forwardThe code fragment above contains a list of integers named my_list and a pair of counter-controlled loops. Once this code has been executed the variables qux and egg will have been assigned values. Let x denote the average those two values. If you were to create a new list that contains every integer in my_list that is greater than than x but also less than than x + 7 then what would be the length of that list? This question is internally recognized as variant 235. Q13) 1 2 3 4 5 6 7 8 none of the abovearrow_forwardInstructions Write a program to test various operations of the class doublyLinkedList. Your program should accept a list of integers from a user and use the doubleLinkedList class to output the following: The list in ascending order. The list in descending order. The list after deleting a number. A message indicating if a number is contained in the list. Output of the list after using the copy constructor. Output of the list after using the assignment operator. An example of the program is shown below: Enter a list of positive integers ending with -999: 83 121 98 23 57 33 -999 List in ascending order: 23 33 57 83 98 121 List in descending order: 121 98 83 57 33 23 Enter item to be deleted: 57 List after deleting 57 : 23 33 83 98 121 Enter item to be searched: 23 23 found in the list. ********Testing copy constructor*********** intList: 23 33 83 98 121 ********Testing assignment operator*********** temp: 23 33 83 98 121 Your program should use the value -999 to denote the end of the…arrow_forward
- Write a function, to be included in an unsorted linked list class, called getLargest, that will return the largest item in the list.arrow_forwardjava Suppose namesis an ABList<String>containing 10elements. The call names.add(0, "George") results in: A. an exception being thrown. B. a 10-element list with "George" as the first element. C. an 11-element list with "George" as the first element. D. a single element list containing "George". E. None of these is correct.arrow_forwardIN PYTHON PLEASE Write a function def sameSet(a, b) that checks whether two lists have the same ele- ments in some order, ignoring duplicates. For example, the two lists 149 16 9 7 4 9 11 and 11 11 7 9 16 4 1 would be considered identical. You will probably need one or more helper functions.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
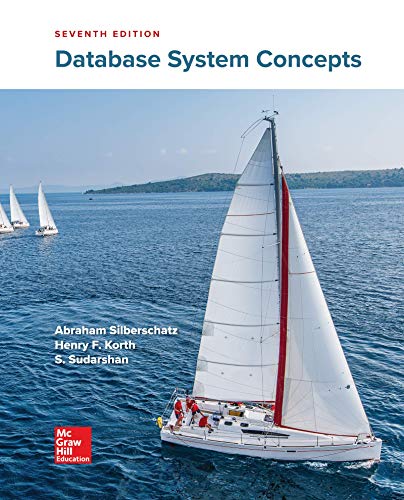
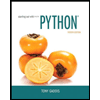
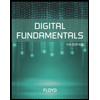
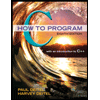
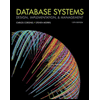
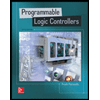