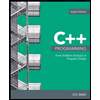
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN: 9781337102087
Author: D. S. Malik
Publisher: Cengage Learning
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Create a C++ linked list class for integers. Appending, inserting, and removing nodes should be class member functions. Add a list destructor. Drive the class.
Add a print function to your linked list class. Display all linked list values. Start with an empty list, add elements, and print the list to test the class.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Question 2: Linked List Implementation You are going to create and implement a new Linked List class. The Java Class name is "StringLinkedList". The Linked List class only stores 'string' data type. You should not change the name of your Java Class. Your program has to implement the following methods for the StringLinked List Class: 1. push(String e) - adds a string to the beginning of the list (discussed in class) 2. printList() prints the linked list starting from the head (discussed in class) 3. deleteAfter(String e) - deletes the string present after the given string input 'e'. 4. updateToLower() - changes the stored string values in the list to lowercase. 5. concatStr(int p1, int p2) - Retrieves the two strings at given node positions p1 and p2. The retrieved strings are joined as a single string. This new string is then pushed to the list using push() method.arrow_forwardPart2: LinkedList implementation 1. Create a linked list of type String Not Object and name it as StudentName. 2. Add the following values to linked list above Jack Omar Jason. 3. Use addFirst to add the student Mary. 4. Use addLast to add the student Emily. 5. Print linked list using System.out.println(). 6. Print the size of the linked list. 7. Use removeLast. 8. Print the linked list using for loop for (String anobject: StudentName){…..} 9. Print the linked list using Iterator class. 10. Does linked list hava capacity function? 11. Create another linked list of type String Not Object and name it as TransferStudent. 12. Add the following values to TransferStudent linked list Sara Walter. 13. Add the content of linked list TransferStudent to the end of the linked list StudentName 14. Print StudentName. 15. Print TransferStudent. 16. What is the shortcoming of using Linked List?arrow_forward@6 The Reference-based Linked Lists: Select all of the following statements that are true. options: As a singly linked list's node references both its predecessor and its successor, it is easily possible to traverse such a list in both directions. According to the terminology introduced in class, the head reference variable in a singly linked list object references the list's first node. According to the terminology introduced in class, in a doubly linked list, each node references both the head and tail node. In a double-ended singly linked list, the tail reference variable provides access to the entire list. In a circular linked list, the last node references the first node.arrow_forward
- python programming Write a function that will insert a new value into the middle of a Linked List. INPUT: The head of the Linked List, the value to insert OUTPUT: Nothing is output RETURNED: Nothing is returnedarrow_forward1. Design your own linked list class to hold a series of integers using C++. The class should have member functions for appending, inserting, and deleting nodes. Don't forget to add a destructor that destroys the list. Demonstrate the class with a driver program. 2. Modify the linked list class you created to add a print member function. The function should display all the values in the linked list. Test the class by starting with an empty list, adding some elements, and then printing the resulting list out.arrow_forwardReference-based Linked Lists: Select all of the following statements that are true. As a singly linked list's node references both its predecessor and its successor, it is easily possible to traverse such a list in both directions. According to the terminology introduced in class, the head reference variable in a singly linked list object references the list's first node. According to the terminology introduced in class, in a doubly linked list, each node references both the head and tail node. In a double-ended singly linked list, the tail reference variable provides access to the entire list. In a circular linked list, the last node references the first node.arrow_forward
- Having trouble with creating the InsertAtEnd function in the ItemNode.h file below. " // TODO: Define InsertAtEnd() function that inserts a node // to the end of the linked list" Given main(), define an InsertAtEnd() member function in the ItemNode class that adds an element to the end of a linked list. DO NOT print the dummy head node. Ex. if the input is: 4 Kale Lettuce Carrots Peanuts ------------------------------------------------------- main.cpp -------------------------------------------------------- #include "ItemNode.h" int main() { ItemNode *headNode; // Create intNode objects ItemNode *currNode; ItemNode *lastNode; string item; int i; int input; // Front of nodes list headNode = new ItemNode(); lastNode = headNode; cin >> input; for (i = 0; i < input; i++) { cin >> item;…arrow_forwardC++ Question You need to write a class called LinkedList that implements the following List operations: public void add(int index, Object item); // adds an item to the list at the given index, that index may be at start, end or after or before the // specific element 2.public void remove(int index); // removes the item from the list that has the given index 3.public void remove(Object item); // finds the item from list and removes that item from the list 4.public List duplicate(); // creates a duplicate of the list // postcondition: returns a copy of the linked list 5.public List duplicateReversed(); // creates a duplicate of the list with the nodes in reverse order // postcondition: returns a copy of the linked list with the nodes in 6.public List ReverseDisplay(); //print list in reverse order 7.public Delete_Smallest(); // Delete smallest element from linked list 8.public List Delete_duplicate(); // Delete duplicate elements from a given linked list.Retain the…arrow_forwardC++ Question You need to write a class called LinkedList that implements the following List operations: public void add(int index, Object item); // adds an item to the list at the given index, that index may be at start, end or after or before the // specific element 2.public void remove(int index); // removes the item from the list that has the given index 3.public void remove(Object item); // finds the item from list and removes that item from the list 4.public List duplicate(); // creates a duplicate of the list // postcondition: returns a copy of the linked list 5.public List duplicateReversed(); // creates a duplicate of the list with the nodes in reverse order // postcondition: returns a copy of the linked list with the nodes in 6.public List ReverseDisplay(); //print list in reverse order 7.public Delete_Smallest(); // Delete smallest element from linked list 8.public List Delete_duplicate(); // Delete duplicate elements from a given linked list.Retain the…arrow_forward
- 6. Suppose that we have defined a singly linked list class that contains a list of unique integers in ascending order. Create a method that merges the integers into a new list. Note the additional requirements listed below. Notes: ● . Neither this list nor other list should change. The input lists will contain id's in sorted order. However, they may contain duplicate values. For example, other list might contain id's . You should not create duplicate id's in the list. Important: this list may contain duplicate id's, and other list may also contain duplicate id's. You must ensure that the resulting list does not contain duplicates, even if the input lists do contain duplicates.arrow_forward1. Write the following member methods, and add the appropriate code in the main application to invoke them: a. count(): returns the number of nodes in the linked listb. displayPreSucc(T val): displays the data values of the predecessor and successor nodes of val in the list. code in java program and in single linked listarrow_forwardin c++ In a linked list made of integer data type, write only an application file that prints thoseintegers that are divisible by 5. Note that you assume that linked list exists, you onlyprints the value of data items that meet the condition (divisible by 5)arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
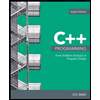
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning