*in java* A contact list is a place where you can store a specific contact with other associated information such as a phone number, email address, birthday, etc. Write a program that first takes as input an integer N that represents the number of word pairs in the list to follow. Word pairs consist of a name and a phone number (both strings), separated by a comma. That list is followed by a name, and your program should output the phone number associated with that name. Assume that the list will always contain less than 20 word pairs. Ex: If the input is: 3 Joe,123-5432 Linda,983-4123 Frank,867-5309 Frank the output is: 867-5309 Your program must define and call the following method. The return value of getPhoneNumber() is the phone number associated with the specific contact name. public static String getPhoneNumber(String[] nameArr, String[] phoneNumberArr, String contactName, int arraySize) Hint: Use two arrays: One for the string names, and the other for the string phone numbers. import java.util.Scanner; public class LabProgram { /* Define your method here */ public static void main(String[] args) { /* Type your code here. */ }
*in java*
A contact list is a place where you can store a specific contact with other associated information such as a phone number, email address, birthday, etc. Write a program that first takes as input an integer N that represents the number of word pairs in the list to follow. Word pairs consist of a name and a phone number (both strings), separated by a comma. That list is followed by a name, and your program should output the phone number associated with that name. Assume that the list will always contain less than 20 word pairs.
Ex: If the input is:
3 Joe,123-5432 Linda,983-4123 Frank,867-5309 Frank
the output is:
867-5309
Your program must define and call the following method. The return value of getPhoneNumber() is the phone number associated with the specific contact name.
public static String getPhoneNumber(String[] nameArr, String[] phoneNumberArr, String contactName, int arraySize)
Hint: Use two arrays: One for the string names, and the other for the string phone numbers.
import java.util.Scanner;
public class LabProgram {
/* Define your method here */
public static void main(String[] args) {
/* Type your code here. */
}
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

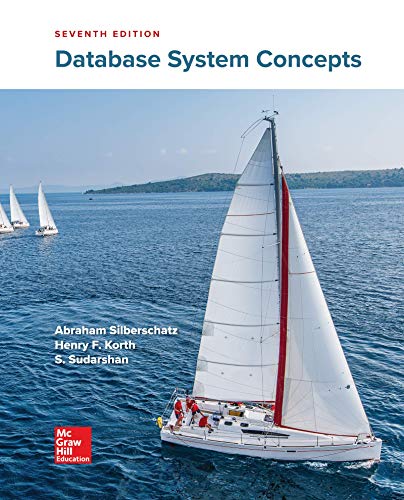
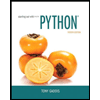
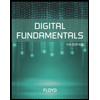
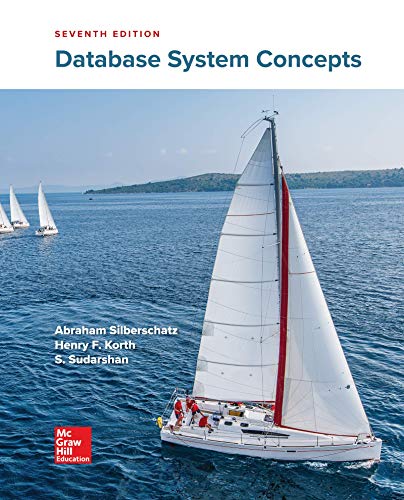
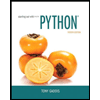
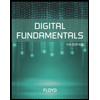
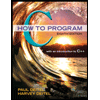
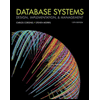
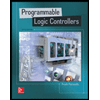