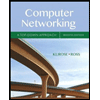
I'm recieving a ton of errors on my Java doc for an assignment I have can anyone help me fix them?
code:
package chapter.pkg6.part.pkg2.assignment;
/**
*
* @author matty
*/
public class Chapter6Part2Assignment {
/**
* @param args the command line arguments
*/
public static void main(String[] args) {
// Method
long cardnumber = 4440967484181607L;
System.out.println("Please enter the card number/n");
System.out.println(cardnumber + " is " + (validitychk(cardnumber) ? "valid" : "invalid"));
// Return true if the card number is valid
public static boolean validitychk(long cnumber) {
return (thesize(cardnumber)>=13&& thesize(cnumber)<= 16)&&(prefixmatch(cardnumber, 4)
|| prefixmatch(cardnumber,5) || prefixmatch(cnumber,37) || prefixmatch(cardnumber, 6))
&& ((sumdoubleeven(cnumber) + sumodd(cnumber)) % 10 == 0);
}
public static int sumdoubleeven(long cardnumber) {
int sum = 0;
String num = cardnumber + "";
for (int i = thesize(cardnumber) - 2; i >= 0; i -= 2)
sum += getDigit(Integer.parseInt(num.charAt(i) + "") * 2);
return sum;
}
public static int getDigit(int cardnumber) {
if (cardnumber < 9)
return cardnumber;
return cardnumber / 10 + cardnumber % 10;
}
public static int sumodd(long cardnumber) {
int sum = 0;
String num = cardnumber + "";
for (int i = thesize(cardnumber) - 1; i >= 0; i -= 2)
sum += Integer.parseInt(num.charAt(i) + "");
return sum;
}
}
I attached an image of the code and the error issue as well.
![### Java Program for Credit Card Validation
This code is a Java program used to validate a credit card number. It uses Luhn's algorithm, which involves checking the size and prefix of the card number.
#### Code Explanation
```java
/**
* Author: [Author Name]
* Param: args the command line arguments
*/
public class Chapter6Part2Assignment {
// Main method
public static void main(String[] args) {
long cardnumber = 4410976841816071L;
System.out.println("Please enter the card number:\n");
System.out.println(cardnumber + " is " + (validitychk(cardnumber) ? "valid" : "invalid"));
// Return true if the card number is valid
}
public static boolean validitychk(long cnumber) {
return (thesize(cnumber) >= 13 && thesize(cnumber) <= 16) && (prefixmatch(cnumber, 4)
|| prefixmatch(cnumber, 5) || prefixmatch(cnumber, 6) || prefixmatch(cnumber, 37))
&& ((sumdoubleeven(cnumber) + sumodd(cnumber)) % 10 == 0);
}
public static int sumdoubleeven(long cardnumber) {
int sum = 0;
String num = cardnumber + "";
for (int i = thesize(cardnumber) - 2; i >= 0; i -= 2) {
sum += getDigit(Integer.parseInt(num.charAt(i) + "") * 2);
}
return sum;
}
public static int getDigit(int cardnumber) {
if (cardnumber < 9)
return cardnumber;
return cardnumber / 10 + cardnumber % 10;
}
public static int sumodd(long cardnumber) {
int sum = 0;
String num = cardnumber + "";
for (int i = thesize(cardnumber) - 1; i >= 0; i -= 2)
sum += Integer.parseInt(num.charAt(i) + "");
return sum;
}
public static boolean prefixmatch(long cardnumber, int d) {
return getprefix(cardnumber, thesize(d)) == d;
}
public static int thesize(long d) {
String num = d + "";
return num.length();](https://content.bartleby.com/qna-images/question/e73c8c7e-1fd5-4385-96bf-6506c467a37a/c1e44586-57f1-4580-8ee0-9225311403c9/6kyby6w_thumbnail.png)


Step by stepSolved in 3 steps with 4 images

- The Delicious class – iterative methods The Delicious class must:• Define a method called setDetails which has the following header: public void setDetails(String name, int price, boolean available) This must find in the ArrayList the Chicken with the given name, change itspricePerKilo to the given price parameter and set whether it is in stock or not. A value of true for the available parameter means the chicken is in stock and a value of false means it is sold out (not in stock). Note that there will only be at most one Chicken object of the given name stored in the ArrayList.• Define a method called removeChicken that takes a single String parameter representing a chicken’s name. This method must remove from the ArrayList the Chicken object (if any) with the given name. The method must return true if a cheese with the given name was found and removed, and false otherwise.The Delicious class – challenge method In the Delicious class complete the findClosestAvailable method: This…arrow_forwardYou have to use comment function to describe what each line does import java.io.BufferedReader; import java.io.FileNotFoundException; import java.io.FileReader; import java.io.IOException; import java.util.ArrayList; import java.util.Arrays; import java.util.List; public class PreferenceData { private final List<Student> students; private final List<Project> projects; private int[][] preferences; private static enum ReadState { STUDENT_MODE, PROJECT_MODE, PREFERENCE_MODE, UNKNOWN; }; public PreferenceData() { super(); this.students = new ArrayList<Student>(); this.projects = new ArrayList<Project>(); } public void addStudent(Student s) { this.students.add(s); } public void addStudent(String s) { this.addStudent(Student.createStudent(s)); } public void addProject(Project p) { this.projects.add(p); } public void addProject(String p) { this.addProject(Project.createProject(p)); } public void createPreferenceMatrix() { this.preferences = new…arrow_forwardpython: class Student:def __init__(self, first, last, gpa):self.first = first # first nameself.last = last # last nameself.gpa = gpa # grade point average def get_gpa(self):return self.gpa def get_last(self):return self.last class Course:def __init__(self):self.roster = [] # list of Student objects def add_student(self, student):self.roster.append(student) def course_size(self):return len(self.roster) # Type your code here if __name__ == "__main__":course = Course()course.add_student(Student('Henry', 'Nguyen', 3.5))course.add_student(Student('Brenda', 'Stern', 2.0))course.add_student(Student('Lynda', 'Robison', 3.2))course.add_student(Student('Sonya', 'King', 3.9)) student = course.find_student_highest_gpa()print('Top student:', student.first, student.last, '( GPA:', student.gpa,')')arrow_forward
- import java.util.Scanner; public class Inventory { public static void main (String[] args) { Scanner scnr = new Scanner(System.in); InventoryNode headNode; InventoryNode currNode; InventoryNode lastNode; String item; int numberOfItems; int i; // Front of nodes list headNode = new InventoryNode(); lastNode = headNode; int input = scnr.nextInt(); for(i = 0; i < input; i++ ) { item = scnr.next(); numberOfItems = scnr.nextInt(); currNode = new InventoryNode(item, numberOfItems); currNode.insertAtFront(headNode, currNode); lastNode = currNode; } // Print linked list currNode = headNode.getNext(); while (currNode != null) { currNode.printNodeData(); currNode…arrow_forward8) Now use the Rectangle class to complete the following tasks: - Create another object of the Rectangle class named box2 with a width of 100 and height of 50. Note that we are not specifying the x and y position for this Rectangle object. Hint: look at the different constructors) Display the properties of box2 (same as step 7 above). - Call the proper method to move box1 to a new location with x of 20, and y of 20. Call the proper method to change box2's dimension to have a width of 50 and a height of 30. Display the properties of box1 and box2. - Call the proper method to find the smallest intersection of box1 and box2 and store it in reference variable box3. - Calculate and display the area of box3. Hint: call proper methods to get the values of width and height of box3 before calculating the area. Display the properties of box3. 9) Sample output of the program is as follow: Output - 1213 Module2 (run) x run: box1: java.awt. Rectangle [x=10, y=10,width=40,height=30] box2: java.awt.…arrow_forwardIn java just add code between the comments public class A6 {/*** In this Java file, you will write three methods that will ensure that the calls in the main methods are* properly executed. You do NOT have to change anything in the main() method. You have to write the methods that* will complete the program.** Pay attention to the method name, the method return type, and the method parameters.*/public static void main(String[] args) {/*Your first method will ask user to enter two values and return the exponent where the firstnumber will be the base and the second will be the exponent. Remember to use a mathematical function.If the user enters value 1 as 2 and value 2 as 3, output of your method should be 8 (2 raised to power 3)*/double calculatedExponent = exponentCalculator();System.out.println(calculatedExponent); //this should print out the exponent value/* Your second method will have two parameters. The first is a String and the second is a number. The methodshould return the…arrow_forward
- InheritanceDemo.java: // StudentName Today's Date public class InheritanceDemo { public static void main(String[] args) { KleenexBox kb1 = new KleenexBox(); KleenexBox kb2 = new KleenexBox(100); Box justABox = new Box(); System.out.println("justABox has area " + BoxArea(justABox)); System.out.println("kb1 has area " + BoxArea(kb1)); System.out.println("kb2 has this many square inches of tissue: " + KleenexArea(kb2)); } public static double BoxArea (Box b) { return b.width * b.length; } public static double KleenexArea (KleenexBox a) { return a.width * a.length * a.numTissues; } } Box.java: // Student Name Today's Date public class Box { public double width; public double length; public String label; } KleenexBox.java: // Student's Name Today's Date public class KleenexBox extends Box { public int numTissues; // A new Kleenex box public KleenexBox() { numTissues = 50; length = 8.0; width = 5.0; } // A new Kleenex box with a specific number of tissues public…arrow_forwardjava 1 error with my code pls help correct my codearrow_forwardimport java.awt.*; public class TestRectangle {public static void main(String[] args) {Rectangle r1 = new Rectangle(5, 4, 10, 17);Rectangle r2 = new Rectangle(10, 10, 20, 3);Rectangle r3 = new Rectangle(0, 1, 12, 15);Rectangle r4 = new Rectangle(10, 10, 20, 3);System.out.println("r1 = " + r1);System.out.println("r2 = " + r2);System.out.println("r3 = " + r3);System.out.println("r2 equals r1? " + r2.equals(r1));System.out.println("r2 equals r2? " + r2.equals(r2));System.out.println("r2 equals r3? " + r2.equals(r3));System.out.println("r2 equals r4? " + r2.equals(r4)); System.out.println("r1 contains (6, 8)? = " + r1.contains(6, 8));System.out.println("r2 contains (6, 8)? = " + r2.contains(6, 8));System.out.println("r3 contains (6, 8)? = " + r3.contains(6, 8));System.out.println("r2 contains (30, 13)? = " + r2.contains(30, 13)); r1.intersect(r3);r2.intersect(r4);System.out.println("r1 intersect r3 = " + r1);System.out.println("r2 intersect r4 = " + r2);…arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
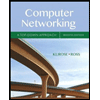
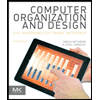
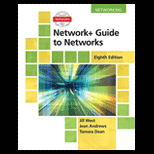
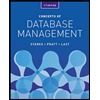
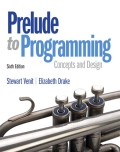
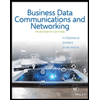