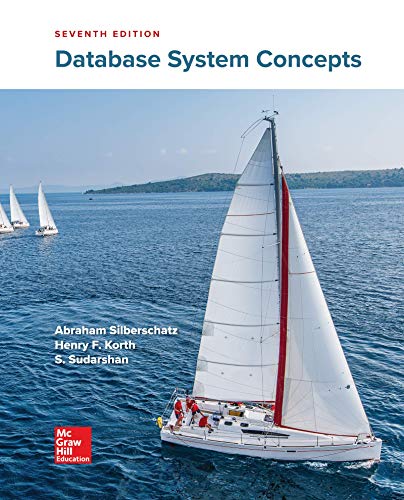
Concept explainers
I need help with my Python code please I am getting errors.
def read_data(filename):
try:
with open(filename, "r") as file:
return file.read()
except Exception as exception:
print(exception)
return None
def extract_data(tags, strings):
data = []
start_tag = f"<{tags}>"
end_tag = f"</{tags}>"
while start_tag in strings:
try:
start_index = strings.find(start_tag) + len(start_tag)
end_index = strings.find(end_tag)
value = strings[start_index:end_index]
if value:
data.append(value)
strings = strings[end_index + len(end_tag):]
except Exception as exception:
print(exception)
return data
def get_names(string):
names = extract_data("name", string)
return names
def get_descriptions(string):
descriptions = extract_data("description", string)
return descriptions
def get_calories(string):
calories = extract_data("calories", string)
return calories
def get_prices(string):
prices = extract_data("price", string)
return prices
def display_menu(names, descriptions, calories, prices):
for i in range(len(names)):
print(f"{names[i]} - {descriptions[i]} - {calories[i]} - {prices[i]}")
def display_stats(calories, prices):
total_items = len(calories)
average_calories = sum(map(int, calories)) / total_items
correct_price = []
for price in prices:
if price:
correct_price.append(float(price[1:]))
else:
correct_price.append(0.0)
average_price = sum(correct_price) / total_items
print(f"{total_items} items - {average_calories:.1f} average calories - ${average_price:.2f} average price")
def main():
filename = "simple.xml"
string = read_data(filename)
if string:
names = get_names(string)
descriptions = get_descriptions(string)
calories = get_calories(string)
prices = get_prices(string)
if names and prices and descriptions and calories:
display_menu(names, descriptions, calories, prices)
display_stats(calories, prices)
main()
![Run CIS 106 Python Tests
failed 6 minutes ago in 11s
Test with pytest
567 Input:
568 No records
569 Output:
570 FAILED .github/workflows/test_final_project.py::test_final_project_missing_fields
incorrect. Expected 'Error: Missing or bad data'.
566 FAILED .github/workflows/test_final_project.py::test_final_project_no_records - AssertionError: Final Project Final Project Error message is missing or
incorrect. Expected 'File is empty' or 'No data'.
571 Input:
572 Missing fields
573 Output:
574 Belgian Waffles Two of our famous Belgian Waffles with plenty of real maple syrup 650 $5.95
575 Strawberry Belgian Waffles - Light Belgian waffles covered with strawberries and whipped cream 900 - $7.95
576
Berry-Berry Belgian Waffles - Light Belgian waffles covered with an assortment of fresh berries and whipped cream 900 $8.95
Homestyle Breakfast Thick slices made from our homemade sourdough bread 600 - $4.50
577
578
5 items 800.0 average calories $6.86 average price
579
Q Search logs
FAILED .github/workflows/test_final_project.py::test_final_project_bad_data AssertionError: Final Project Final Project Error message is missing or
incorrect. Expected 'Error: Missing or bad data'.
Output:
Belgian Waffles
580
Input:
581 Bad data
582
- Two of our famous Belgian Waffles with plenty of real maple syrup
650 $5.95
583
584 Strawberry Belgian Waffles Light Belgian waffles covered with strawberries and whipped cream 900 -
$7.95
585
Berry-Berry Belgian Waffles Light Belgian waffles covered with an assortment of fresh berries and whipped cream - 900 $8.95
French Toast Thick slices made from our homemade sourdough bread 600 - X
586
587
Homestyle Breakfast Two eggs, bacon or sausage, toast, and our ever-popular hash browns 950 - $6.95
588
589
590
591
592
593
594
File "/home/runner/work/CIS106-Jan-Miguel/CIS106-Jan-Miguel/Final Project/Final project.py", line 72, in display_stats
correct_price.append(float (price [1::]))
595
596 ValueError: could not convert string to float:
display_stats (calories, prices)
AssertionError: Final Project Final Project Error message is missing or
Traceback (most recent call last):
File "/home/runner/work/CIS106-Jan-Miguel/CIS106-Jan-Miguel/Final Project/Final project.py", line 92, in <module>
main()
File "/home/runner/work/CIS106-Jan-Miguel/CIS106-Jan-Miguel/Final Project/Final project.py", line 89, in main
22381/jobs/10129275954
6 failed, 45 passed, 35 skipped in 1.76s
(5
2s](https://content.bartleby.com/qna-images/question/df788c22-83be-4a9a-a241-87bf44363afe/d82f4bbd-83d0-4c3f-b228-2b174a90866e/1hkjv15_thumbnail.png)

Step by stepSolved in 4 steps with 3 images

- Question R .Full explain this question and text typing work only We should answer our question within 2 hours takes more time then we will reduce Rating Dont ignore this linearrow_forwardI need some help making this code more simple. 2) AlgorithmtokenList = "{}()[]"initialize stack to emptyGet file name from user: fileNameOpen the file (fileName)read line from fileName into strLinewhile not EOF (end of file)find tokenList items in strLine for each token in strLineif token = "[" thenpush "]" into stackelse if token = "(" thenpush ")" into stackelse if token = "{" thenpush "}" into stackelse if token = "]" OR token = ")" OR token = "}" thenpop stack into poppedTokenif poppedToken not equal tokenprint "Incorrect Grouping Pairs"exitend ifend ifnext tokenend whileif stack is emptyprint "Correct Grouping Pairs"elseprint "Incorrect Grouping Pairs"end ifarrow_forwardIn pythonarrow_forward
- Suppose names is an ABList containing 10 elements. The call names.add(0, "George") results in: A. an exception being thrown. B. a 10-element list with "George" as the first element. C. an 11-element list with "George" as the first element. D. a single element list containing "George". E. None of these is correct.arrow_forwarddef analyze_file (filename: str, pos_words: List [str], neg_words: List [str]) -> Given the name of a file, a list of positive words (all in lowercase), and a list of negative words (all in lowercase), return some interesting data about the file in a tuple, with the first item in the tuple being the positivity score of the contents of this file, and the rest of the item: being whatever else you end up analyzing. (Helper functions are highly encouraged here.) How to calculate positivity score: For every word that is pos itive in the file (based on the words in the list of positive words) add 1 to the score. For every negative word (based on the list of negative words), subtract 1 from the score. Any neutral words (those that do not appear in either list) do not affect the score in any way. passarrow_forwardPYTHON!!! Write a function that will filter course grades that are stored in a list, creating and returning a new list. Your implementation must have the following rules: - The function must accept two (and only two) parameters: The first is: the maximum integer value for any grade to remain in the list (any value above the maximum will be filtered and removed from the list). The second is: the list of grades (you can assume the list contains only integer values) - The function must return a new list and not modify the existing list. - The function must use a list comprehension to filter the existing list and create the new list that is returned. - DO NOT USE THE: built-in-"filter" function.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
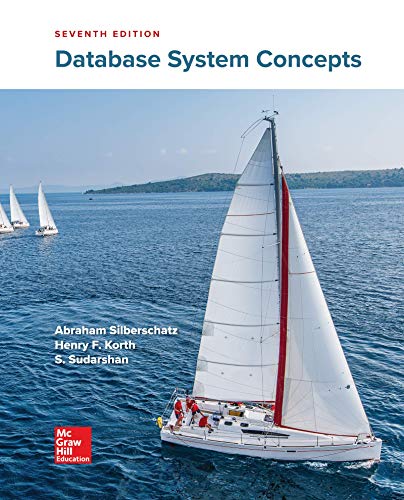
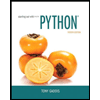
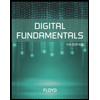
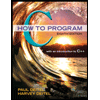
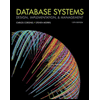
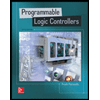