Exception handling to detect input string vs. int C++ The given program reads a list of single-word first names and ages (ending with -1), and outputs that list with the age incremented. The program fails and throws an exception if the second input on a line is a string rather than an int. At FIXME in the code, add a try/catch statement to catch ios_base::failure, and output 0 for the age. Ex: If the input is: Lee 18 Lua 21 Mary Beth 19 Stu 33 -1 then the output is: Lee 19 Lua 22 Mary 0 Stu 34 #include #include using namespace std; int main() { string inputName; int age; // Set exception mask for cin stream cin.exceptions(ios::failbit); cin >> inputName; while(inputName != "-1") { // FIXME: The following line will throw an ios_base::failure. // Insert a try/catch statement to catch the exception. // Clear cin's failbit to put cin in a useable state. cin >> age; cout << inputName << " " << (age + 1) << endl; cin >> inputName; } return 0; }
Exception handling to detect input string vs. int C++
The given program reads a list of single-word first names and ages (ending with -1), and outputs that list with the age incremented. The program fails and throws an exception if the second input on a line is a string rather than an int. At FIXME in the code, add a try/catch statement to catch ios_base::failure, and output 0 for the age.
Ex: If the input is:
Lee 18 Lua 21 Mary Beth 19 Stu 33 -1
then the output is:
Lee 19 Lua 22 Mary 0 Stu 34
#include <string>
#include <iostream>
using namespace std;
int main() {
string inputName;
int age;
// Set exception mask for cin stream
cin.exceptions(ios::failbit);
cin >> inputName;
while(inputName != "-1") {
// FIXME: The following line will throw an ios_base::failure.
// Insert a try/catch statement to catch the exception.
// Clear cin's failbit to put cin in a useable state.
cin >> age;
cout << inputName << " " << (age + 1) << endl;
cin >> inputName;
}
return 0;
}

The given C++ program is as follows:
#include <string>
#include <iostream>
using namespace std;
int main() {
string inputName;
int age;
// Set exception mask for cin stream
cin.exceptions(ios::failbit);
cin >> inputName;
while(inputName != "-1") {
// FIXME: The following line will throw an ios_base::failure.
// Insert a try/catch statement to catch the exception.
// Clear cin's failbit to put cin in a useable state.
cin >> age;
cout << inputName << " " << (age + 1) << endl;
cin >> inputName;
}
return 0;
}
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

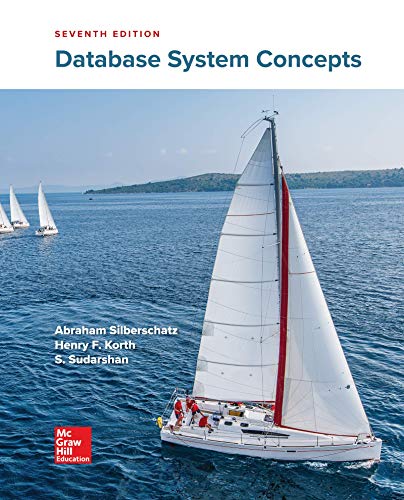
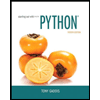
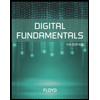
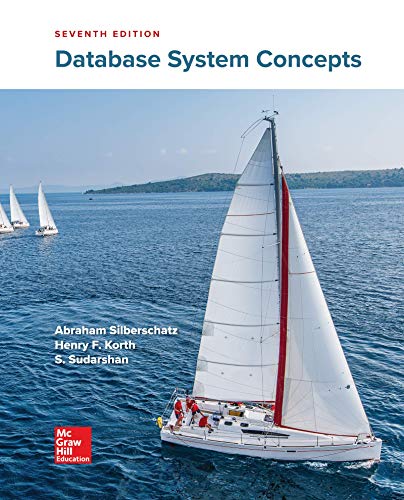
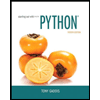
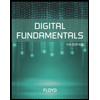
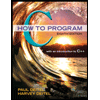
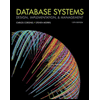
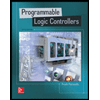