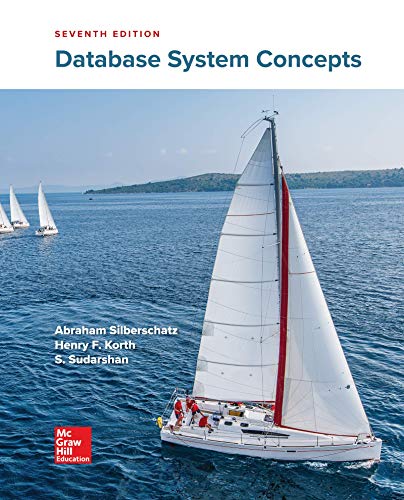
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Note: you must use exceptions and try-catch blocks
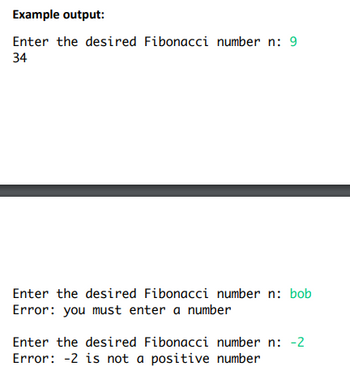
Transcribed Image Text:Example output:
Enter the desired Fibonacci number n: 9
34
Enter the desired Fibonacci number n: bob
Error: you must enter a number
Enter the desired Fibonacci number n: -2
Error: -2 is not a positive number
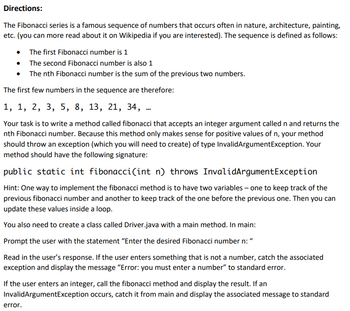
Transcribed Image Text:Directions:
The Fibonacci series is a famous sequence of numbers that occurs often in nature, architecture, painting,
etc. (you can more read about it on Wikipedia if you are interested). The sequence is defined as follows:
• The first Fibonacci number is 1
•
•
The first few numbers in the sequence are therefore:
1, 1, 2, 3, 5, 8, 13, 21, 34,
Your task is to write a method called fibonacci that accepts an integer argument called n and returns the
nth Fibonacci number. Because this method only makes sense for positive values of n, your method
should throw an exception (which you will need to create) of type InvalidArgumentException. Your
method should have the following signature:
public static int fibonacci(int n) throws InvalidArgumentException
Hint: One way to implement the fibonacci method is to have two variables - one to keep track of the
previous fibonacci number and another to keep track of the one before the previous one. Then you can
update these values inside a loop.
You also need to create a class called Driver.java with a main method. In main:
Prompt the user with the statement "Enter the desired Fibonacci number n: "
Read in the user's response. If the user enters something that is not a number, catch the associated
exception and display the message "Error: you must enter a number" to standard error.
The second Fibonacci number is also 1
The nth Fibonacci number is the sum of the previous two numbers.
If the user enters an integer, call the fibonacci method and display the result. If an
InvalidArgumentException occurs, catch it from main and display the associated message to standard
error.
Expert Solution

arrow_forward
Explanation
1) Below is Java program to read a number from user, validate it and display its Fibonacci number.
- It imports scanner to read user inputs
- In main method of program
- Create a scanner object to read user input
- Ask user to enter a number
- Validate the read number using try catch statement
- Read integer
- Check if number is positive
- Call fibonacci function passing number n as parameter
- Display the returned fibonacci number
- Else display it is not a positive number
- On exception, display error message, Error. You must enter a number
- Define method to that calculates the fibonacci number using recursive approach
2) Save program in Driver.java and run
Step by stepSolved in 3 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- A(n) ____ should be used to specify all the exception types when being handled by a single exception handler. list O tuple array O dictarrow_forwardPLZ help with the following IN JAVA If a method throws an exception, and the exception is not caught inside the method, then the method invocation: terminates transfers control to the catch block transfers control to the exception handler none of the abovearrow_forwardException Code Put this code in a try-catch block. You will need to handle exceptions for DividebyZero, Array error, and general error . In your catches, you may simply just print out an error message: x = car[x] / bar[y];arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
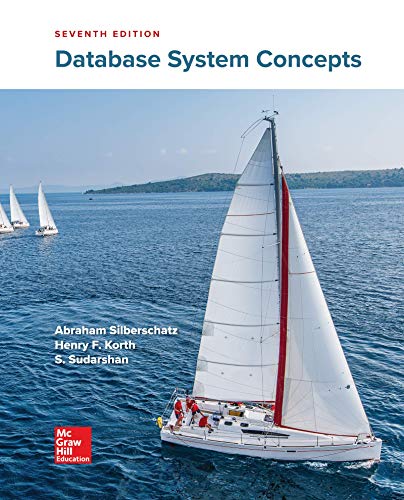
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
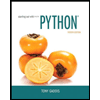
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
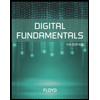
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
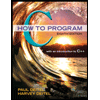
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
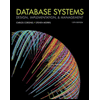
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
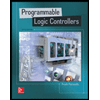
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education