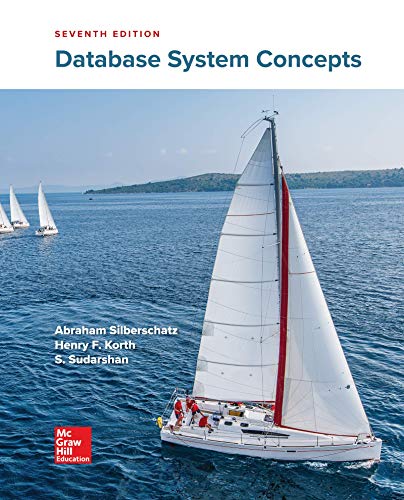
I need help in improving my code. This is an elevator simulator that uses polymorphism and object-oriented programming to simulate the movement of elevators in a building with multiple types of passengers and elevators.
The system has 8 elevators, each of which can be one of 4 types of elevators, with a certain
percentage of requests for each type. Similarly, each passenger can be one of 4 types, with a
different percentage of requests for each type.
The simulation should have 4 types of Passengers:
Standard: This is the most common type of passenger and has a request percentage of 70%. Standard passengers have no special requirements.
VIP: This type of passenger has a request percentage of 10%. VIP passengers are given priority and are more likely to be picked up by express elevators.
Freight: This type of passenger has a request percentage of 15%. Freight passengers have large items that need to be transported and are more likely to be picked up by freight elevators.
Glass: This type of passenger has a request percentage of 5%. Glass passengers have fragile items that need to be transported and are more likely to be picked up by glass elevators.
Passenger {
Should have the variable used in sub classes
initializes Passenger Counter - keeps count of number of passengers
}
Sub-classes (Standarda, Vip, Frieght, Glass) {
Use the polymorphic methods from Passenger class
requestElevator - Uses random to generate a Start floor and End floor.
getPercent - Retrieves the percent from the setting.txt file.
}
This is what I have:
public class Simulation {
SimulatorSettings settings = new SimulatorSettings("settings.txt");
public void InitSimulation() throws FileNotFoundException{
///// Read all parameters from the file and store in the clas
File file = new File("settings.txt");
Scanner scanner = new Scanner(file);
//FileReader freader = new FileReader(file);
while(scanner.hasNextLine()){
String line = scanner.nextLine();
if(line.startsWith("floor="))
{
line= line.replace("floor=", "");
// Convert the value to the wanted type
System.out.println(line);
}
}
settings.setNofloors(55);
Passenger pass1 = new StandardPassenger();
pass1.requestElevator(settings);
ArrayList<Passenger> passengers = null;
for(int i = 0; i < 100; i++){
//Use the percentage from the file
passengers.add(new StandardPassenger());
}
}
}
public class SimulatorSettings {
SimulatorSettings(String fileName){
}
private int nofloors;
public int getNofloors() {
return nofloors;
}
public void setNofloors(int nofloors) {
this.nofloors = nofloors;
}
}
import java.util.Random;
public abstract class Passenger {
public static int passengerCounter = 0;
private String passengerID;
protected int startFloor;
protected int endFloor;
Passenger() {
this.passengerID = "" + passengerCounter;
passengerCounter++;
}
public abstract boolean requestElevator(SimulatorSettings settings);
}
class StandardPassenger extends Passenger {
public StandardPassenger() {
super();
}
@Override
public boolean requestElevator(SimulatorSettings settings) {
Random rand = new Random();
this.startFloor = rand.nextInt(settings.getNofloors());
this.endFloor = rand.nextInt(settings.getNofloors());
while (this.startFloor == this.endFloor) {
this.endFloor = rand.nextInt(settings.getNofloors());
}
return true;
}
}
class VIPPassenger extends Passenger {
public VIPPassenger() {
super();
}
@Override
public boolean requestElevator(SimulatorSettings settings) {
// Request an express elevator
int expressElevator = settings.getNofloors() / 2;
this.startFloor = expressElevator;
this.endFloor = expressElevator;
return true;
}
}
class FreightPassenger extends Passenger {
public FreightPassenger() {
super();
}
@Override
public boolean requestElevator(SimulatorSettings settings) {
Random rand = new Random();
this.startFloor = rand.nextInt(settings.getNofloors());
this.endFloor = rand.nextInt(settings.getNofloors());
while (this.startFloor == this.endFloor) {
this.endFloor = rand.nextInt(settings.getNofloors());
}
return true;
}
}
class GlassPassenger extends Passenger {
public GlassPassenger() {
super();
}
@Override
public boolean requestElevator(SimulatorSettings settings) {
Random rand = new Random();
this.startFloor = rand.nextInt(settings.getNofloors());
this.endFloor = rand.nextInt(settings.getNofloors());
while (this.startFloor == this.endFloor) {
this.endFloor = rand.nextInt(settings.getNofloors());
}
return true;
}
}
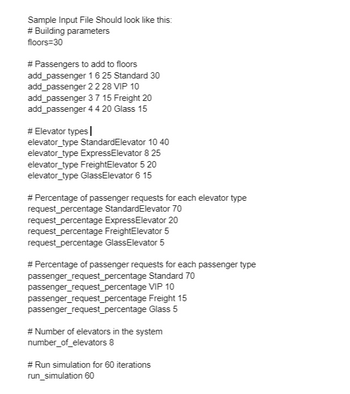

Step by stepSolved in 4 steps with 6 images

- This assignment is a review of loops. Do not use anything more advanced than a loop, such as programmer-defined functions or arrays or classes. If you know blackjack, you may be tempted to change the specifications below to make the game more "blackjack-like". Please don't. Be sure to follow these specs exactly and don't try to improve on them. You will be penalized if the specs are not met exactly. In the card game named 'blackjack' players get two cards to start with, and then they are asked whether or not they want more cards. Players can continue to take as many cards as they like. Their goal is to get as close as possible to a total of 21 without going over. Face cards have a value of 10. Write a command line game that plays a simple version of blackjack. The program should generate a random number between 1 and 10 each time the player gets a card. Each of the values (1 through 10) must be equally likely. (In other words, this won't be like real black jack where getting a 10 is…arrow_forwardI also am struggling with this project but do not understand this code. The problem I have uses a main.cpp file alongside a dateTypelmp.cpp file. This solution does not solve the task as I understand it to be. The class dateType was designed to implement the date in a program, but the member function setDate and the constructor do not check whether the date is valid before storing the date in the member variables. Function setDate and the constructor need to be rewrit so that the values for the month, day, and year are checked before storing the date into the member variables. A member function should be included, isLeapYear, to check whether a year is a leap year. Input should be format month day year with each separated by a space. Output should resemble the following: Date #: month-day-year If the year is a leap year, print the date and a message indicating it is a leap year, otherwise print a message indicating that it is not a leap year. The header file for the class dateType has…arrow_forwardAssume that CSCourse class has been written, which has the below UML Diagram. You are giyen the CourseManager class and are asked to complete the main method. Write a body of the main method only that creates two objects of type CSCourse, so that: the first CSCourse is named "CS210% and has 32 students and an average GPA of 3.1 the second CSCourse is named "CS211" and has 31 students and an average GPA of 3.4 sum the students in two courses using the getNumStudents() method, and print the sum to the screen. C) cSCourse name : String -numStudents: int -averageGPA double +CSCourse(name String, numStu int) +getNumStudents() int +getName(): String +setAverageGPA(AVGPA: double) : void public class CourseManager{ public static void main(String[] args) [ / Your code goes here // Sample output is "Sum of students: 63".arrow_forward
- B elow for each class you find a UML and description of the public interface. Implementing the public interface as described is madatory. There's freedom on how to implement these classes.The private properties and private methods are under your control.. There are multiple ways of implementing all these classes. Feel free to add private properties and methods. For each object, it's mandatory to create a header file (.h), implementation file (.cpp) and a driver. Blank files are included. The header should have the class definition in it. The implementation file should contain the implementations of the methods laid out in the header fine. And finally the Driver should test/demonstrate all the features of the class. It's best to develop the driver as the class is being written. Check each section to see if there are added additional requirements for the driver. Two test suites are included so that work can be checked. It's important to implement the drivers to test and demonstrate…arrow_forwardDraw the UML diagram for the class. Implement the class. Write a test program that creates an Account object with an account ID of 1122, a balance of $20,000, and an annual interest rate of 4.5%. Use the withdraw method to withdraw $2,500, use the deposit method to deposit $3,000, and print the balance, the monthly interest, and the date when this account was created.arrow_forwardelow for each class you find a UML and description of the public interface. Implementing the public interface as described is madatory. There's freedom on how to implement these classes.The private properties and private methods are under your control.. There are multiple ways of implementing all these classes. Feel free to add private properties and methods. For each object, it's mandatory to create a header file (.h), implementation file (.cpp) and a driver. Blank files are included. The header should have the class definition in it. The implementation file should contain the implementations of the methods laid out in the header fine. And finally the Driver should test/demonstrate all the features of the class. It's best to develop the driver as the class is being written. Check each section to see if there are added additional requirements for the driver. Two test suites are included so that work can be checked. It's important to implement the drivers to test and demonstrate…arrow_forward
- add a class to package that represents your new drivable type. That type should be something that is capable of being driven (speeding up and slowing down) and therefore a perfect candidate for a class that implements the actions in the Drivable interface.arrow_forwardI need to change this code into object oriented code. here's how to objects are supposed to be setup. First you will need to make a Timer object. This object should function like a traditional stopwatch with methods for starting, stopping, resetting, and reporting back times. The design of the object itself is up to you (it should minimally contain methods for the aforementioned ideas), but it must consist of a solitary object that provides interfaces appropriate for being compositionally included as part of a sorting object to facilitate the time keeping portion of this exercise. Make sure you have a properly separated specification and implementation file for your Timer/Stopwatch object. The second object you will make should be a data housing object that has methods that enable client code to read in the formatted contents of data files, house the data in memory, and execute bubble sort, selection sort, and insertion sort algorithms in a timed fashion with the assistance of your…arrow_forwardSolve using OOP in Java.arrow_forward
- Develop a set of classes for a college to use in various student service andpersonnel applications. Classes you need to design include the following:• Person—A Person contains a first name, last name, street address, zip code,and phone number. The class also includes a method that sets each datafield, using a series of dialog boxes and a display method that displays all of aPerson’s information on a single line at the command line on the screenarrow_forwardHi, I am making a elevator simulation and I need help in making the passenger classes using polymorphism. Any help is appreciated. There are 4 types of passengers in the system:Standard: This is the most common type of passenger and has a request percentage of 70%. Standard passengers have no special requirements.VIP: This type of passenger has a request percentage of 10%. VIP passengers are given priority and are more likely to be picked up by express elevators.Freight: This type of passenger has a request percentage of 15%. Freight passengers have large items that need to be transported and are more likely to be picked up by freight elevators.Glass: This type of passenger has a request percentage of 5%. Glass passengers have fragile items that need to be transported and are more likely to be picked up by glass elevators. This is what I have so far // Passenger abstract classpublic abstract class Passenger { protected String type; protected int requestPercentage; public…arrow_forwardDesign a class called Stopwatch. The job of this class is to simulate a stopwatch. It should provide two methods: Start and Stop. We call the start method first, and the stop method next. Then we ask the stopwatch about the duration between start and stop. Duration should be a value in TimeSpan. Display the duration on the console. We should also be able to use a stopwatch multiple times. So we may start and stop it and then start and stop it again. Make sure the duration value each time is calculated properly. We should not be able to start a stopwatch twice in a row (because that may overwrite the initial start time). So the class should throw an InvalidOperationException if its started twice. 1arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
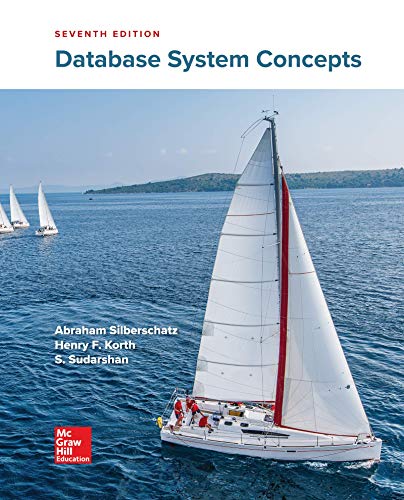
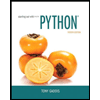
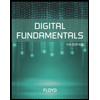
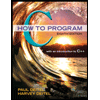
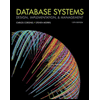
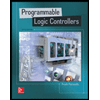