Hi, I am making a elevator simulation and I need help in making the passenger classes using polymorphism. Any help is appreciated. There are 4 types of passengers in the system: Standard: This is the most common type of passenger and has a request percentage of 70%. Standard passengers have no special requirements. VIP: This type of passenger has a request percentage of 10%. VIP passengers are given priority and are more likely to be picked up by express elevators. Freight: This type of passenger has a request percentage of 15%. Freight passengers have large items that need to be transported and are more likely to be picked up by freight elevators. Glass: This type of passenger has a request percentage of 5%. Glass passengers have fragile items that need to be transported and are more likely to be picked up by glass elevators.
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Hi, I am making a elevator simulation and I need help in making the passenger classes using polymorphism. Any help is appreciated.
There are 4 types of passengers in the system:
Standard: This is the most common type of passenger and has a request percentage of 70%.
Standard passengers have no special requirements.
VIP: This type of passenger has a request percentage of 10%. VIP passengers are given priority
and are more likely to be picked up by express elevators.
Freight: This type of passenger has a request percentage of 15%. Freight passengers have large
items that need to be transported and are more likely to be picked up by freight elevators.
Glass: This type of passenger has a request percentage of 5%. Glass passengers have fragile items
that need to be transported and are more likely to be picked up by glass elevators.
This is what I have so far
// Passenger abstract class
public abstract class Passenger {
protected String type;
protected int requestPercentage;
public Passenger(String type, int requestPercentage) {
this.type = type;
this.requestPercentage = requestPercentage;
}
public String getType() {
return this.type;
}
public int getRequestPercentage() {
return this.requestPercentage;
}
public abstract void requestElevator();
}
// Standard passenger class
public class StandardPassenger extends Passenger {
public StandardPassenger() {
super("Standard", 70);
}
@Override
public void requestElevator() {
// perform standard passenger request logic
}
}
// VIP passenger class
public class VIPPassenger extends Passenger {
public VIPPassenger() {
super("VIP", 10);
}
@Override
public void requestElevator() {
// perform VIP passenger request logic
}
}
// Freight passenger class
public class FreightPassenger extends Passenger {
public FreightPassenger() {
super("Freight", 15);
}
@Override
public void requestElevator() {
// perform freight passenger request logic
}
}
// Glass passenger class
public class GlassPassenger extends Passenger {
public GlassPassenger() {
super("Glass", 5);
}
@Override
public void requestElevator() {
// perform glass passenger request logic
}
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps

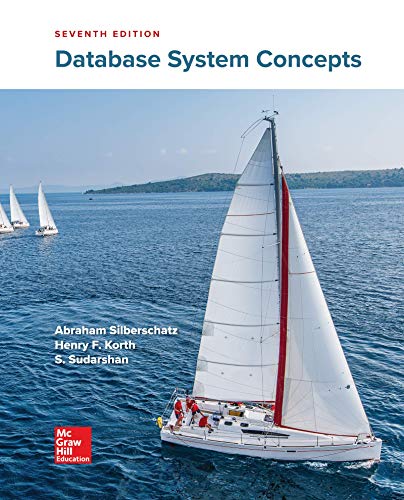
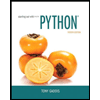
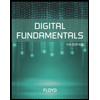
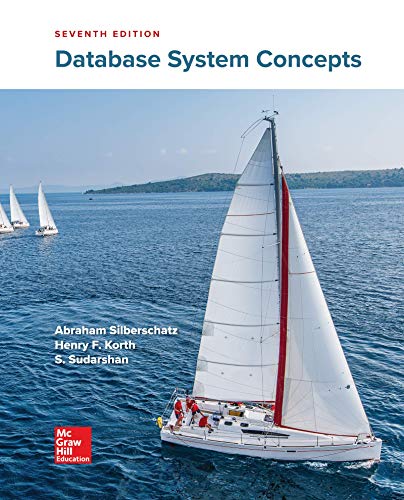
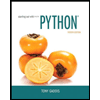
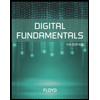
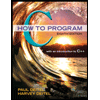
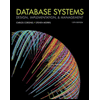
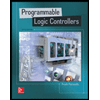