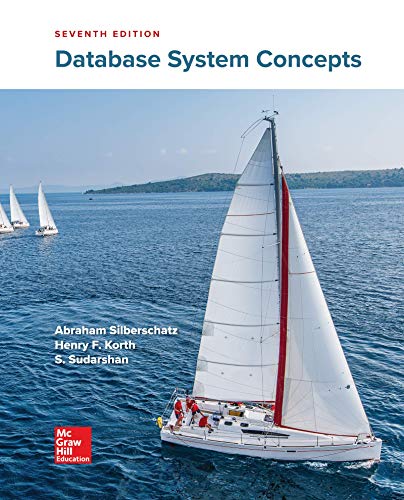
Concept explainers
Create a C#
The Conference class contains fields for the following:
- group - The group name (as a string)
- date - The starting date (as a string)
- attendees - The number of attendees (as an int)
Include properties for each field.
Also, include an IComparable.CompareTo() method so that Conference objects can be sorted in order from least to greatest attendees.
Your output for each conference should match the following:
NAME Conference starts on DATE and has ATTENDEES attendees
Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 2 images

I have an error in the code:
Failed : ConferenceClassIComparableTest.ConferenceTest `Conference` class should implement `IComparable` interface Expected: True But was: False at ConferenceClassIComparableTest.ConferenceTest () [0x00000] in <a9f68112596545e395054a4bf32f46b4>:0
Hello, I've put the code in but i'm getting an error.
Unhandled Exception: System.ArgumentNullException: Value cannot be null. Parameter name: s at System.Int32.Parse (System.String s) [0x00003] in <7b90a8780ac4414295b539b19eea7eea>:0 at ConferencesDemo.Main () [0x00049] in <2548cd4334a74f9da563c01621fa757b>:0 [ERROR] FATAL UNHANDLED EXCEPTION: System.ArgumentNullException: Value cannot be null. Parameter name: s at System.Int32.Parse (System.String s) [0x00003] in <7b90a8780ac4414295b539b19eea7eea>:0 at ConferencesDemo.Main () [0x00049] in <2548cd4334a74f9da563c01621fa757b>:0
I have an error in the code:
Failed : ConferenceClassIComparableTest.ConferenceTest `Conference` class should implement `IComparable` interface Expected: True But was: False at ConferenceClassIComparableTest.ConferenceTest () [0x00000] in <a9f68112596545e395054a4bf32f46b4>:0
Hello, I've put the code in but i'm getting an error.
Unhandled Exception: System.ArgumentNullException: Value cannot be null. Parameter name: s at System.Int32.Parse (System.String s) [0x00003] in <7b90a8780ac4414295b539b19eea7eea>:0 at ConferencesDemo.Main () [0x00049] in <2548cd4334a74f9da563c01621fa757b>:0 [ERROR] FATAL UNHANDLED EXCEPTION: System.ArgumentNullException: Value cannot be null. Parameter name: s at System.Int32.Parse (System.String s) [0x00003] in <7b90a8780ac4414295b539b19eea7eea>:0 at ConferencesDemo.Main () [0x00049] in <2548cd4334a74f9da563c01621fa757b>:0
- in c # i need to Create an application named TurningDemo that creates instances of four classes: Page, Corner, Pancake, and Leaf. Create an interface named ITurnable that contains a single method named Turn(). The classes named Page, Corner, Pancake, and Leaf implement ITurnable. Create each class’s Turn() method to display an appropriate message. For example: The Page’s Turn() method should display You turn a page in a book. The Corner’s Turn() method should display You turn corners to go around the block. The Pancake's Turn() method should display You turn a pancake when it's done on one side. The Leaf's Turn() method should display A leaf turns colors in the fall. i keep getting errors Unit TestIncompletePage class defined Build StatusBuild FailedBuild OutputCompilation failed: 3 error(s), 0 warnings NtTest3c897a9a.cs(12,26): error CS0246: The type or namespace name `ITurnable' could not be found. Are you missing `TurningDemo' using directive?NtTest3c897a9a.cs(23,7): error…arrow_forwardUsing a class throttle, programs can declare objects of the class. As with any other data type, you may declare throttle variables. These variables are called throttle objects, or sometimes throttle _______________. They are declared in the same way as variables of any other type. Group of answer choices instances structures substances casesarrow_forwardin C# i need to Create an application named TurningDemo that creates instances of four classes: Page, Corner, Pancake, and Leaf. Create an interface named ITurnable that contains a single method named Turn(). The classes named Page, Corner, Pancake, and Leaf implement ITurnable. Create each class’s Turn() method to display an appropriate message. For example: The Page’s Turn() method should display You turn a page in a book.The Corner’s Turn() method should display You turn corners to go around the block.The Pancake's Turn() method should display You turn a pancake when it's done on one side.The Leaf's Turn() method should display A leaf turns colors in the fall. but I keep getting errors witch are Build StatusBuild FailedBuild OutputCompilation failed: 1 error(s), 0 warnings TurningDemo.cs(2,0): error CS1525: Unexpected symbol `usingSystem' Unit TestIncomplete Leaf class defined Build Status Build Failed Build OutputCompilation failed: 1 error(s), 0 warnings…arrow_forward
- C# i need to Create an application named TurningDemo that creates instances of four classes: Page, Corner, Pancake, and Leaf. Create an interface named ITurnable that contains a single method named Turn(). The classes named Page, Corner, Pancake, and Leaf implement ITurnable. Create each class’s Turn() method to display an appropriate message. For example: The Page’s Turn() method should display You turn a page in a book.The Corner’s Turn() method should display You turn corners to go around the block.The Pancake's Turn() method should display You turn a pancake when it's done on one side.The Leaf's Turn() method should display A leaf turns colors in the fall i keep getting errors C# i need to Create an application named TurningDemo that creates instances of four classes: Page, Corner, Pancake, and Leaf. Create an interface named ITurnable that contains a single method named Turn(). The classes named Page, Corner, Pancake, and Leaf implement ITurnable. Create each class’s Turn()…arrow_forward// Declare data fields: a String named customerName, // an int named numItems, and // a double named totalCost.// Your code here... // Implement the default contructor.// Set the value of customerName to "no name"// and use zero for the other data fields.// Your code here... // Implement the overloaded constructor that// passes new values to all data fields.// Your code here... // Implement method getTotalCost to return the totalCost.// Your code here... // Implement method buyItem.//// Adds itemCost to the total cost and increments// (adds 1 to) the number of items in the cart.//// Parameter: a double itemCost indicating the cost of the item.public void buyItem(double itemCost){// Your code here... }// Implement method applyCoupon.//// Apply a coupon to the total cost of the cart.// - Normal coupon: the unit discount is subtracted ONCE// from the total cost.// - Bonus coupon: the unit discount is subtracted TWICE// from the total cost.// - HOWEVER, a bonus coupon only applies if the…arrow_forwardPortfolio Instructions: You are working for a financial advisor who creates portfolios of financial securities for his clients. A portfolio is a conglomeration of various financial assets, such as stocks and bonds, that together create a balanced collection of investments. When the financial advisor makes a purchase of securities on behalf of a client, a single transaction can include multiple shares of stock or multiple bonds. It is your job to create an object-oriented application that will allow the financial advisor to maintain the portfolios for his/her clients. You will need to create several classes to maintain this information: Security, Stock, Bond, Portfolio, and Date. The characteristics of stocks and bonds in a portfolio are shown below: Stocks: Bonds: Purchase date (Date) Purchase date (Date) Purchase price (double)…arrow_forward
- A object which overloads the () operator is called a _______. Group of answer choices overload operator function object Overload object constructor objectarrow_forwardCreate a C# program named ConferencesDemo for a hotel that hosts business conferences. Allows a user to enter data about five Conference objects and then displays them in order of attendance from smallest to largest. The Conference class contains fields for the following: group - The group name (as a string) date - The starting date (as a string) attendees - The number of attendees (as an int) Include properties for each field. Also, include an IComparable.CompareTo() method so that Conference objects can be sorted in order from least to greatest attendees. Your output for each conference should match the following: NAME Conference starts on DATE and has ATTENDEES attendeesarrow_forwardin c# i need to Create an application named TurningDemo that creates instances of four classes: Page, Corner, Pancake, and Leaf. Create an interface named ITurnable that contains a single method named Turn(). The classes named Page, Corner, Pancake, and Leaf implement ITurnable. Create each class’s Turn() method to display an appropriate message. For example: The Page’s Turn() method should display You turn a page in a book.The Corner’s Turn() method should display You turn corners to go around the block.The Pancake's Turn() method should display You turn a pancake when it's done on one side.The Leaf's Turn() method should display A leaf turns colors in the fall.i keep getting the errors Unit TestIncompletePage class defined Build StatusBuild FailedBuild OutputCompilation failed: 3 error(s), 0 warnings NtTest4ac148a9.cs(12,26): error CS0246: The type or namespace name `ITurnable' could not be found. Are you missing `TurningDemo' using directive?NtTest4ac148a9.cs(23,7): error CS0246:…arrow_forward
- in C#, Visual Basic Create a Car class that has at least the following properties:• Year• Make• Price• Passengers-holds the number of seat belts available in the car (presumably how many passengers the car can hold). This field must be private since users presumably can’t change this value. Have two constructors: one that initializes everything to some default value for the base price of a car and one that also accepts values for luxury upgrades of: heated seats, sun-roof, and satellite radio. The class has two methods: 1) Get Paint Color() which accepts a standard base color option but allows the user to purchase a pricier customized color 2) Upgrade Costs() which computes the cost of user-selected luxury items and adds it to the price. Create three car objects: Ford (make Explorer), Lexus (make ES) and a Clown Car which overrides the passenger value to allow 20 clowns in the car. The Get Paint Color() and Upgrade Costs() functions needs to be called for each object and data for all…arrow_forwardin C# i need to Create an application named TurningDemo that creates instances of four classes: Page, Corner, Pancake, and Leaf. Create an interface named ITurnable that contains a single method named Turn(). The classes named Page, Corner, Pancake, and Leaf implement ITurnable. Create each class’s Turn() method to display an appropriate message. For example: The Page’s Turn() method should display You turn a page in a book. The Corner’s Turn() method should display You turn corners to go around the block. The Pancake's Turn() method should display You turn a pancake when it's done on one side. The Leaf's Turn() method should display A leaf turns colors in the fall.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
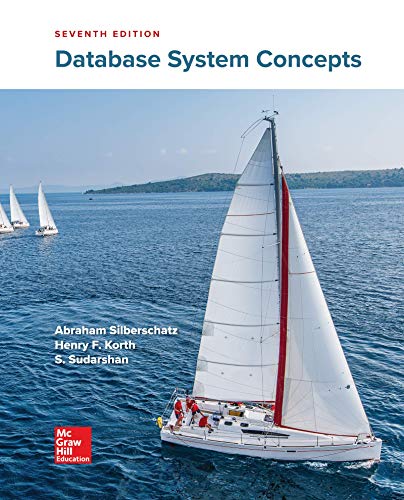
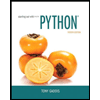
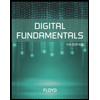
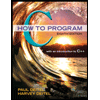
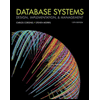
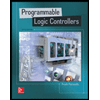