How would I get this code to read multiple files? JAVA import java.io.File; import java.io.FileNotFoundException; import java.util.Scanner; public class BabyName { private int y; private char g; private String n; int getbabyYear() { return y; } String getbabyName() { return n; } void getuserInput() { Scanner ns= new Scanner(System.in); System.out.println("Enter the year: "); y=ns.nextInt(); System.out.println("Enter the gender: "); g=ns.next().charAt(0); System.out.println("Enter the name: "); n=ns.next(); ns.close(); } int findRank() { File file=new File("/Users/apers/OneDrive/Desktop/babyranking/babynameranking_1980s.txt"); try { Scanner nf = new Scanner(file); //declare the variable 'r' for rank int r; //declare the variable 'bn' for boyname and 'gn' for girl name String bN,gN; while(nf.hasNext()) { r=nf.nextInt(); bN=nf.next(); nf.next(); gN=nf.next(); nf.next(); if(g=='M'){ if(bN.equals(n)){ return r; } } if(g=='F'){ if(gN.equals(n)){ return r; } } } nf.close(); } catch (FileNotFoundException e) { e.printStackTrace(); } return -1; } public static void main(String[] args) { BabyName sn=new BabyName(); sn.getuserInput(); int r=sn.findRank(); if(r!=-1){ System.out.println(sn.getbabyName()+" is ranked #"+r+" in year "+sn.getbabyYear()); }else{ System.out.println("The name "+sn.getbabyName()+" is not ranked in year "+sn.getbabyYear()); } } }
How would I get this code to read multiple files?
JAVA
import java.io.File;
import java.io.FileNotFoundException;
import java.util.Scanner;
public class BabyName
{
private int y;
private char g;
private String n;
int getbabyYear()
{
return y;
}
String getbabyName()
{
return n;
}
void getuserInput()
{
Scanner ns= new Scanner(System.in);
System.out.println("Enter the year: ");
y=ns.nextInt();
System.out.println("Enter the gender: ");
g=ns.next().charAt(0);
System.out.println("Enter the name: ");
n=ns.next();
ns.close();
}
int findRank()
{
File file=new File("/Users/apers/OneDrive/Desktop/babyranking/babynameranking_1980s.txt");
try
{
Scanner nf = new Scanner(file);
//declare the variable 'r' for rank
int r;
//declare the variable 'bn' for boyname and 'gn' for girl name
String bN,gN;
while(nf.hasNext())
{
r=nf.nextInt();
bN=nf.next();
nf.next();
gN=nf.next();
nf.next();
if(g=='M'){
if(bN.equals(n)){
return r;
}
}
if(g=='F'){
if(gN.equals(n)){
return r;
}
}
}
nf.close();
}
catch (FileNotFoundException e)
{
e.printStackTrace();
}
return -1;
}
public static void main(String[] args)
{
BabyName sn=new BabyName();
sn.getuserInput();
int r=sn.findRank();
if(r!=-1){
System.out.println(sn.getbabyName()+" is ranked #"+r+" in year "+sn.getbabyYear());
}else{
System.out.println("The name "+sn.getbabyName()+" is not ranked in year "+sn.getbabyYear());
}
}
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

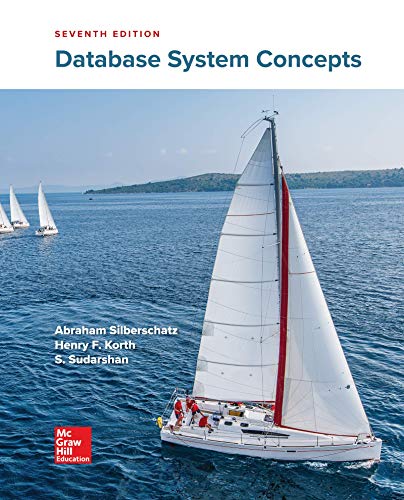
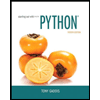
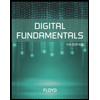
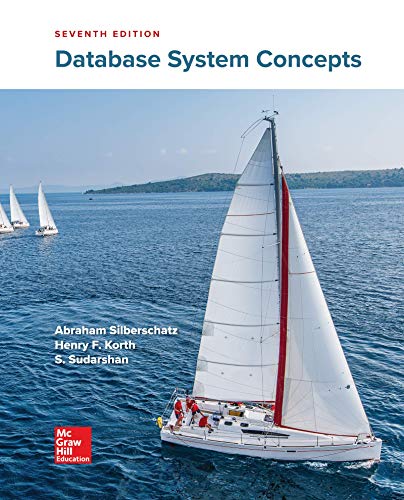
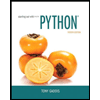
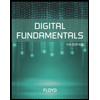
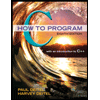
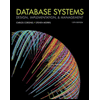
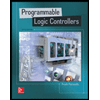