Ex: If the input of the program is: StudentInfo.tsv and the contents of StudentInfo.tsv are: Barrett Bradshaw Charlton Mayo Stern Edan 70 45 59 Reagan 96 97 88 Caius 73 94 80 Tyrese 88 61 36 Brenda 90 86 45 the file report.txt should contain: Barrett Bradshaw Charlton Mayo Stern Edan 70 45 59 F Reagan 96 97 88 A Caius 73 94 80 B Tyrese 88 61 36 D Brenda 90 86 45 с Averages: Midterm1 83.40. Midterm2 76.60. Final 61.60
I need help fixing this java program below:
import java.util.Scanner;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.PrintWriter;
import java.io.IOException;
public class LabProgram {
public static void main(String[] args) throws IOException {
Scanner scnr = new Scanner(System.in);
String fileName;
String[] lastNames, firstNames;
int[] midterm1, midterm2, finalScores;
// Read a file name from the user and read the tsv file
fileName = scnr.next();
try (Scanner fileScanner = new Scanner(new FileInputStream(fileName))) {
int numStudents = Integer.parseInt(fileScanner.nextLine());
lastNames = new String[numStudents];
firstNames = new String[numStudents];
midterm1 = new int[numStudents];
midterm2 = new int[numStudents];
finalScores = new int[numStudents];
for (int i = 0; i < numStudents; i++) {
lastNames[i] = fileScanner.next();
firstNames[i] = fileScanner.next();
midterm1[i] = fileScanner.nextInt();
midterm2[i] = fileScanner.nextInt();
finalScores[i] = fileScanner.nextInt();
}
}
// Compute student grades and exam averages, then output results to a text file
try (PrintWriter outputFile = new PrintWriter(new FileOutputStream("report.txt"))) {
for (int i = 0; i < lastNames.length; i++) {
int average = (midterm1[i] + midterm2[i] + finalScores[i]) / 3;
char letterGrade = getLetterGrade(average);
outputFile.printf("%s %s %d %d %d %c%n", lastNames[i], firstNames[i],
midterm1[i], midterm2[i], finalScores[i], letterGrade);
}
double avgMidterm1 = calculateAverage(midterm1);
double avgMidterm2 = calculateAverage(midterm2);
double avgFinal = calculateAverage(finalScores);
outputFile.printf("Averages: Midterm1 %.2f, Midterm2 %.2f, Final %.2f%n", avgMidterm1, avgMidterm2, avgFinal);
}
}
private static char getLetterGrade(int average) {
if (average >= 90) {
return 'A';
} else if (average >= 80) {
return 'B';
} else if (average >= 70) {
return 'C';
} else if (average >= 60) {
return 'D';
} else {
return 'F';
}
}
private static double calculateAverage(int[] array) {
int sum = 0;
for (int value : array) {
sum += value;
}
return (double) sum / array.length;
}
}
It said this:
Could not find file: report.txt Exception in thread "main" java.lang.NumberFormatException: For input string: "Barrett Edan 70 45 59" at java.base/java.lang.NumberFormatException.forInputString(NumberFormatException.java:65) at java.base/java.lang.Integer.parseInt(Integer.java:652) at java.base/java.lang.Integer.parseInt(Integer.java:770) at LabProgram.main(LabProgram.java:17)


Step by step
Solved in 4 steps with 3 images

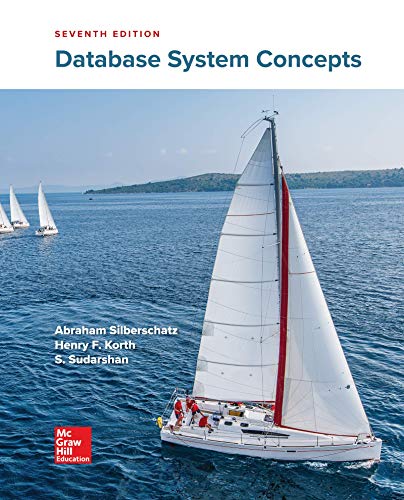
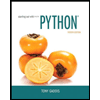
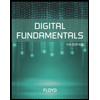
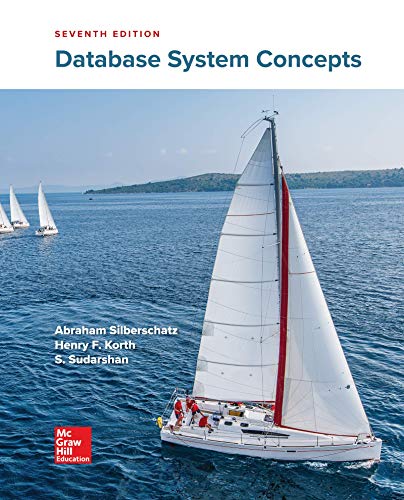
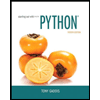
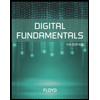
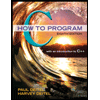
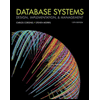
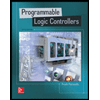