how to fix the error in the remove function (c++)
how to fix the error in the remove function (c++)
struct LinkedList {
public:
LinkedList () : head_(0) {};
~LinkedList () { delete_nodes (); };
// returns 0 on success,-1 on failure
int insert (const int &new_item) {
return ((head_ = new Node(new_item, head_)) != 0) ? 0 : -1;
}
// returns 0 on success, -1 on failure
int remove (const int &item_to_remove) {
Node *marker = head_;
Node *temp = 0; // temp points to one behind as we iterate
while (marker != 0) {
if (marker->value() == item_to_remove) {
if (temp == 0) { // marker is the first element in the list
if (marker->next() == 0) {
head_ = 0;
delete marker; // marker is the only element in the list
marker = 0;
} else {
head_ = new Node(marker->value(), marker->next());
delete marker;
marker = marker->next();
}
return 0;
} else {
temp->next (marker->next());
delete temp;
temp = 0;
return 0;
}
}
// marker = 0; // reset the marker
temp = marker;
marker = marker->next();
}
return -1; // failure
}
void print (void) {
Node *marker = head_;
while (marker != 0) {
printf("%d\n", marker->value());
marker = marker->next();
}
}
private:
void delete_nodes (void) {
Node *marker = head_;
while (marker != 0) {
Node *temp = marker;
delete marker;
marker = temp->next();
}
}

Step by step
Solved in 2 steps with 1 images

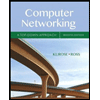
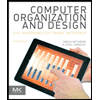
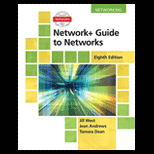
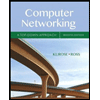
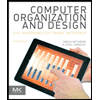
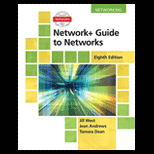
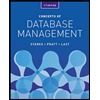
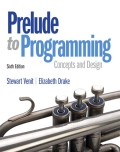
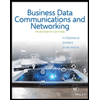