My code does not produce the required output, can anyone let me know what seems to be off? Input: from Node import Node from LinkedList import LinkedList def print_linkedlist(list): print(f'Linked List ------ Nodes ----------------------------------') print(f'head: {list.head.name:<6}', end=' ') node = list.head while node != None: print(f"{node.name:<12}", end=' ') node = node.next print() print(f'tail: {list.tail.name:<6}', end=' ') node = list.head while node != None: print(f"data:{node.data:<7}", end=' ') node = node.next print() print(f' ', end=' ') node = list.head while node != None: if node.next != None: print(f"next:{node.next.name:<7}", end=' ') else: print("next:None") node = node.next print() print(f' ', end=' ') node = list.tail while node != None: if node.prev != None: print(f"prev:{node.prev.name:<7}", end=' ') else: print("prev:None") node = node.prev print() num_list = LinkedList() node_a = Node(14, "node_a") node_b = Node(99, "node_b") node_c = Node(44, "node_c") node_d = Node(95, "node_d") node_e = Node(42, "node_e") node_f = Node(55, "node_f") num_list.append(node_a) print_linkedlist(num_list) num_list.append(node_f) num_list.append(node_e) num_list.prepend(node_b) num_list.insert_after(node_b, node_c) num_list.insert_after(node_c, node_d) num_list.remove(node_f) num_list.remove(node_a) print('List after adding nodes:', end=' ') node = num_list.head while node != None: print(node.data, end=' ') node = node.next print() num_list.remove(node_f) num_list.remove(node_a) print_linkedlist(num_list) print('List after removing nodes:', end=' ') node = num_list.head while node != None: print(node.data, end=' ') node = node.next print() Output: Linked List ------ Nodes ----------------------------------head: node_a node_atail: node_a data:14 next:None prev:None List after adding nodes: 99 44 95 42Linked List ------ Nodes ----------------------------------head: node_b node_b node_c node_d node_etail: node_e data:42 data:95 data:44 data:99 next:node_c next:node_d next:node_e next:None prev:node_d prev:node_c prev:node_b prev:None List after removing nodes: 99 44 95 42 expected output: Linked List ------ Nodes ----------------------------------head: node_b node_b node_c node_d node_e tail: node_e data:99 data:44 data:95 data:42 next:node_c next:node_d next:node_e None prev:None prev:node_b prev:node_c prev:node_d as you can see, my issue is that the prev's are backwards, could it be due to appending incorrectly or something else?
My code does not produce the required output, can anyone let me know what seems to be off?
Input:
Linked List ------ Nodes ----------------------------------
head: node_a node_a
tail: node_a data:14
next:None
prev:None
List after adding nodes: 99 44 95 42
Linked List ------ Nodes ----------------------------------
head: node_b node_b node_c node_d node_e
tail: node_e data:42 data:95 data:44 data:99
next:node_c next:node_d next:node_e next:None
prev:node_d prev:node_c prev:node_b prev:None
List after removing nodes: 99 44 95 42
expected output:
Linked List ------ Nodes ----------------------------------
head: node_b node_b node_c node_d node_e
tail: node_e data:99 data:44 data:95 data:42
next:node_c next:node_d next:node_e None
prev:None prev:node_b prev:node_c prev:node_d
as you can see, my issue is that the prev's are backwards, could it be due to appending incorrectly or something else?
Unlock instant AI solutions
Tap the button
to generate a solution
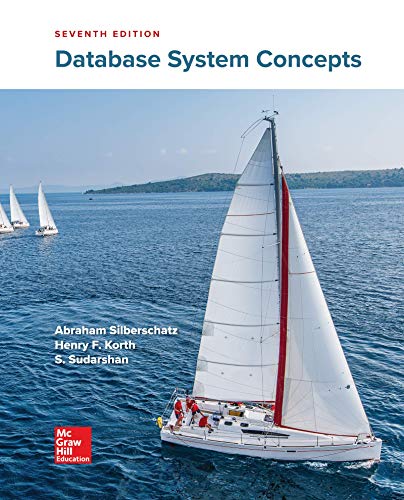
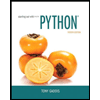
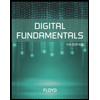
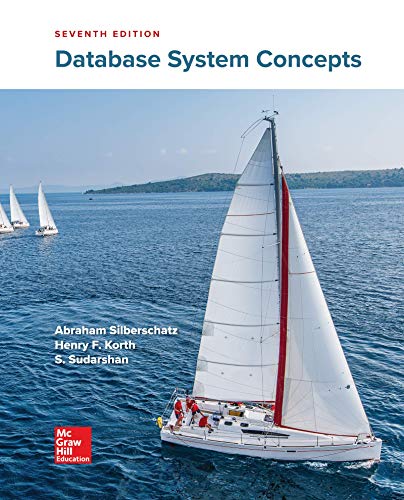
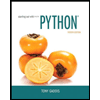
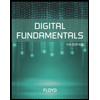
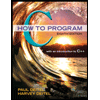
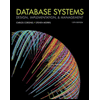
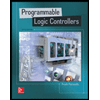