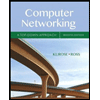
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN: 9780133594140
Author: James Kurose, Keith Ross
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
thumb_up100%
How can I apply this python code in the problem?
def print_multiples(a,b,x):
#Base Case/s
#TODO: Add conditions here for your base case/s
if True :
return 0
#Recursive Case/s
#TODO: Add conditions here for your recursive case/s
else:
return print_multiples(a, b, x)
a = int(input("Please enter a: "))
print(a)
b = int(input("Please enter b: "))
x = int(input("Please enter x: "))
print_multiples(a,b,x)
![Write a program that asks the user to enter three numbers: a, b, and x.
a and b will serve as the lower and upper bounds, respectively, of the range where the multiples of x will be
searched on.
• these 3 numbers must then be used as inputs to your function.
Create a recursive function that takes in 3 inputs and prints the multiples of x in the range [a,b] (inclusive).
Conditions:
• if x is odd, print the multiples in ascending order.
• if x is even, print the multiples in descending order.
Example 1:
Please enter a: 1
Please enter b: 10
Please enter x: 2
10 8 6 4 2
Example 2:
Please enter a: -10
Please enter b: 10
Please enter x: 3
-9 -6 -3 0 3 6 9](https://content.bartleby.com/qna-images/question/5e4b46f5-fd0b-4b69-8fa1-4775c5e817f6/f2ba7c18-d48c-4f3e-afd3-c7af8ca2a353/tj9fm2q_thumbnail.png)
Transcribed Image Text:Write a program that asks the user to enter three numbers: a, b, and x.
a and b will serve as the lower and upper bounds, respectively, of the range where the multiples of x will be
searched on.
• these 3 numbers must then be used as inputs to your function.
Create a recursive function that takes in 3 inputs and prints the multiples of x in the range [a,b] (inclusive).
Conditions:
• if x is odd, print the multiples in ascending order.
• if x is even, print the multiples in descending order.
Example 1:
Please enter a: 1
Please enter b: 10
Please enter x: 2
10 8 6 4 2
Example 2:
Please enter a: -10
Please enter b: 10
Please enter x: 3
-9 -6 -3 0 3 6 9
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by stepSolved in 2 steps with 2 images

Knowledge Booster
Similar questions
- The triangle.cpp ProgramDoes recursion actually work? If you run the triangle.cpp program, you’ll see that itdoes. The program uses recursion to calculate triangular numbers. Enter a value for the term number, n, and the program will display the value of the corresponding triangular number. show the triangle.cpp programarrow_forwardSimple MIPs Recursive Procedure Write a program that calls a recursive procedure. Inside this procedure, add 1 to a counter so you can verify the number of times it executes. Put a number in a MIPS counter that specifies the number of times you want to allow the recursion to continue. You can use a LOOPinstruction (or other conditional statements using MIPS), find a way for therecursive procedure to call itself a fixed number of times.arrow_forwardANSWER SHOULD BE WRITTEN IN JAVA CODE USING RECURSION Problem Statement Given a string as input return true or false depending on whether it satisfies these rules: 1) The string begins with an 'x' 2) Each 'x' is followed by nothing, 'x' or 'yy' 3) Each 'yy' is followed by nothing or 'x'arrow_forward
- in kotlin Write two versions of a recursive palindrome check function. One version should have a block body, and the other should have an expression body. Review the lecture slide about the substring function first. Use this algorithm: if the length of the string is less than 2, return true else if the first and last characters are not equal (!=) return false else return the result of a recursive call with the substring from the second character (the one at index 1) to the second to last character. Use a main that uses a loop to test the palindrome function using this list of strings: val ss = listOf("", "A", "AA", "AB", "AAA", "ABA", "ABB", "AAAA", "AABA", "ABBA", "ABCBA", "ABCAB")arrow_forwardWrite a recursive algorithm with the following prototype: int divide (int x, int y); that returns x/y (integer division). You need not test for divide by 0. THE FUNCTION MUST BE RECURSIVE. (hint: base case should be when xarrow_forwardImplement a recursive is_palindrome(s:str)->bool function, which checks if the given string is a palindrome or not. Examples: >>> is_palindrome('racecar') True >>> is_palindrome('racecars') False please help my homework and put here screenshot. # instruction said not using loopsarrow_forward
- solve q5 only pleasearrow_forwardConvert Newton's method for approximating square roots in Project 1 to a recursive function named newton . (Hint: The estimate of the square root should be passed as a second argument to the function.) An example of the program input and output is shown below: Enter a positive number or enter/return to quit: 2 The program's estimate is 1.4142135623746899 Python's estimate is 1.4142135623730951 Enter a positive number or enter/return to quitarrow_forwardPlease explain the questions related to the code below: //1. Why is 20 printed 3 times and why does it start with 1, but end with 2? //2. What is the base case for this recursive method and why? //3. How would you implement this using a loop? //4. What is each if statement checking? //5. Would a solution to this using loops require more or less code and would it be more or less effecient? //6. Why is recursion a good choice to solve this problem? //7. How can recursion become infinite? //8. When is recursion appropriate? /* Load the code below into a cloud compiler and run each method one at a time by removing the comments for the method. Walk through the code as a group and explore how the code works. Submit the answers to the numbered questions for credit. */ public class DemoRecur { //Simple statement designed to show control flow in recursion public static void printDemo(int x, int max) {…arrow_forward
- Design and implement a recursive program(in java) to determine and print the Nth line of Pascal's triangle, as shown below. Each interior value is the sum of the two values above it. Hint: Use an array to store the values on each line.arrow_forwardWrite a recursive algorithm with the following prototype: int add (int x, int y); that returns x if y is 0; and adds x to y otherwise. THE FUNCTION MUST BE RECURSIVE. (hint: the base case should involve a test for y being 0; recursive case should reduce y towards 0)arrow_forwardPython Question :Which of the following describes the base case in a recursive solution? Group of answer choices the way to return to the main function the case in which the problem is solved through recursion the way to stop the recursion a case in which the problem can be solved without recursionarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
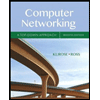
Computer Networking: A Top-Down Approach (7th Edi...
Computer Engineering
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:PEARSON
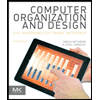
Computer Organization and Design MIPS Edition, Fi...
Computer Engineering
ISBN:9780124077263
Author:David A. Patterson, John L. Hennessy
Publisher:Elsevier Science
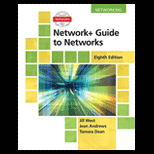
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:9781337569330
Author:Jill West, Tamara Dean, Jean Andrews
Publisher:Cengage Learning
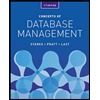
Concepts of Database Management
Computer Engineering
ISBN:9781337093422
Author:Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:Cengage Learning
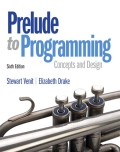
Prelude to Programming
Computer Engineering
ISBN:9780133750423
Author:VENIT, Stewart
Publisher:Pearson Education
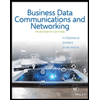
Sc Business Data Communications and Networking, T...
Computer Engineering
ISBN:9781119368830
Author:FITZGERALD
Publisher:WILEY