int x //x coord of the center -int y // y coord of the center -int radius -static int count // static variable to keep count of number of circles created + Circle() //default constructor that sets origin to (0,0) and radius to 1 +Circle(int x, int y, int radius) // regular constructor +getX(): int +getY(): int +getRadius(): int +setX(int newX: void +setY(int newY): void +setRadius(int newRadius):void +getArea(): double // returns the area using formula pi*r^2 +getCircumference // returns the circumference using the formula 2*pi*r +toString(): String // return the circle as a string in the form (x,y) : radius +getDistance(Circle other): double // * returns the distance between the center of this circle and the other circle +moveTo(int newX,int newY):void // * move the center of the circle to the new coordinates +intersects(Circle other): bool //* returns true if the center of the other circle lies inside this circle else returns false +resize(double scale):void// * multiply the radius by the scale +resize(int scale):Circle // * returns a new Circle with the same center as this circle but radius multiplied by scale +getCount():int //returns the number of circles created //note that the resize function is an overloaded function. The definitions have different signatures Data file: 0 0 4 0 0 6 - 2 -9 6 4 5 7 7 8 9 Extend the driver class to do the following: Declare a vector of circles Call a function with signature inputData(vector &, string filename) that reads data from a file called dataLab4.txt into the vector. The following c-e are done in this function Use istringstream to create an input string stream called instream. Initialize it with each string that is read from the data file using the getline method. Read the coordinates for the center and the radius from instream to create the circles Include a try catch statement to take care of the exception that would occur if there was a file open error. Display the message “File Open Error” and exit if the exception occurs Display all the circles in this vector using the toString method Use an iterator to iterate through the vector to display these circles Display the count of all the circles in the vector using the getCount method Display the count of all the circles in the vector using the vector size method Clear the vector Create a circle called c using the default constructor Display the current count of all the circles using the getCount method on c Display the current count of all the circles using the vector size method Write functions in your main driver cpp file that perform the actions b-l. Your code should be modular and your main program should consist primarily of function calls
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
-int x //x coord of the center -int y // y coord of the center -int radius -static int count // static variable to keep count of number of circles created |
+ Circle() //default constructor that sets origin to (0,0) and radius to 1 +Circle(int x, int y, int radius) // regular constructor +getX(): int +getY(): int +getRadius(): int +setX(int newX: void +setY(int newY): void +setRadius(int newRadius):void +getArea(): double // returns the area using formula pi*r^2 +getCircumference // returns the circumference using the formula 2*pi*r +toString(): String // return the circle as a string in the form (x,y) : radius +getDistance(Circle other): double // * returns the distance between the center of this circle and the other circle +moveTo(int newX,int newY):void // * move the center of the circle to the new coordinates +intersects(Circle other): bool //* returns true if the center of the other circle lies inside this circle else returns false +resize(double scale):void// * multiply the radius by the scale +resize(int scale):Circle // * returns a new Circle with the same center as this circle but radius multiplied by scale +getCount():int //returns the number of circles created //note that the resize function is an overloaded function. The definitions have different signatures
|
Data file:
0 0 4
0 0 6
- 2 -9 6
4 5 7
7 8 9
- Extend the driver class to do the following:
- Declare a
vector of circles - Call a function with signature inputData(vector<Circle> &, string filename) that reads data from a file called dataLab4.txt into the vector. The following c-e are done in this function
- Use istringstream to create an input string stream called instream. Initialize it with each string that is read from the data file using the getline method.
- Read the coordinates for the center and the radius from instream to create the circles
- Include a try catch statement to take care of the exception that would occur if there was a file open error. Display the message “File Open Error” and exit if the exception occurs
- Display all the circles in this vector using the toString method
- Use an iterator to iterate through the vector to display these circles
- Display the count of all the circles in the vector using the getCount method
- Display the count of all the circles in the vector using the vector size method
- Clear the vector
- Create a circle called c using the default constructor
- Display the current count of all the circles using the getCount method on c
- Display the current count of all the circles using the vector size method
- Declare a
- Write functions in your main driver cpp file that perform the actions b-l. Your code should be modular and your main program should consist primarily of function calls


Step by step
Solved in 3 steps with 2 images

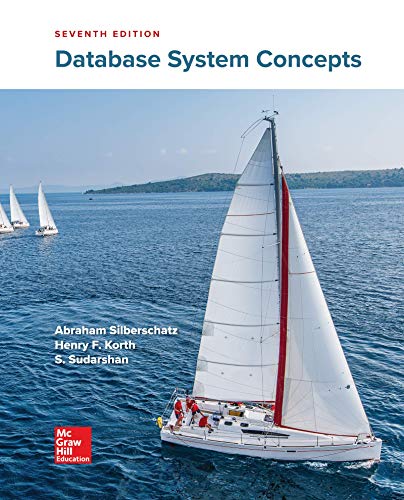
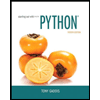
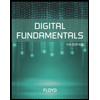
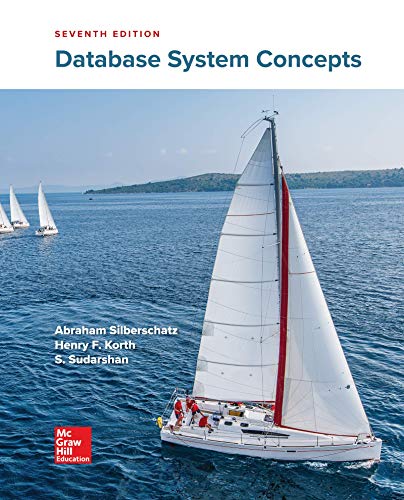
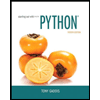
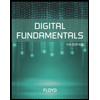
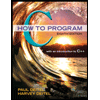
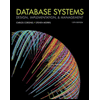
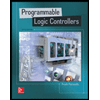