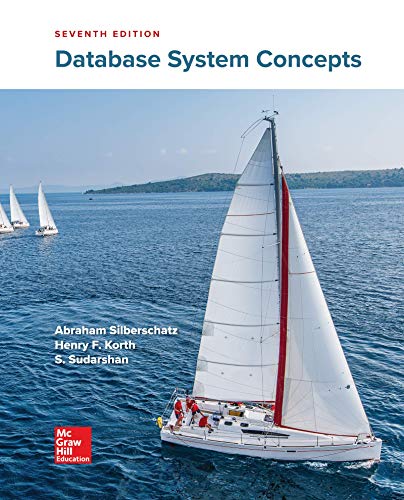
From this code, I wonder how each code process?
#include <iostream>
using namespace std;
int factorial(int n) // function to calculate factorial
{
int i, ans = 1;
for(i = n; i > 0; i--)
ans *= i;
return ans;
}
long int power(int n, int m) // function to calculate power
{
int i;
long int ans = 1;
for(i = m; i > 0; i--)
ans *= n;
return ans;
}
int main()
{
cout << "MATH MENU"; // display math menu
cout << "\n----------------------------------------";
while (1) // infinite loop unless we exit from the
{
cout << "\n1. Calculate n! (n factorial).";
cout << "\n2. Calculate n to the m power.";
cout << "\n3. Exit Program.\n";
int choice;
cout << "\nPlease enter your selection: "; // enter selection
cin >> choice;
if (choice == 1) // for factorial
{
float nf;
cout << "\nEnter an integer value for n (1-9): ";
cin >> nf;
while (nf != (int)nf || nf < 1 || nf > 9) // exceptional cases handled
{ // check for integers and check for numbers in between 1-9
cout << "\nInvalid option. Please re-enter.\n";
cout << "\nEnter an integer value for n (1-9): ";
cin >> nf;
}
cout << "\n" << (int)nf << "! = " << factorial((int)nf) << endl; // display factorial
}
else if (choice == 2)
{
float nf, mf;
cout << "\nEnter an integer value for n (1-9): ";
cin >> nf;
cout << "\nEnter an integer value for m (1-9): ";
cin >> mf;
while (nf != (int)nf || nf < 1 || nf > 9 || mf != (int)mf || mf < 1 || mf > 9)
{ // check as for integers and range in 1-9
cout << "\nInvalid option. Please re-enter.\n";
cout << "\nEnter an integer value for n (1-9): ";
cin >> nf;
cout << "\nEnter an integer value for m (1-9): ";
cin >> mf;
}
cout << "\n" << (int)nf << "^" << (int)mf << " = " << power((int)nf, (int)mf) << endl;
}
else if (choice == 3)
{
break; // exit the program
}
else // retry the loop if invalid option is entered
{
cout << "\nInvalid option. Please re-enter.\n";
}
}
return 0;
}

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 4 images

- 3. (a) A PHP function is defined as follows: function planets ($arr) { if ($arr [1]) { print "Mercury"; } else { for ($i=0; $i <= $arr [2]; $i++) {print $arr [3] [$i]; } } print count ($arr); } Recall that array $arr can be defined using a statement of the form: $arr = array (...); (i) Give an array $arr such that planets ($arr) prints Mercury For this case, what is the output of the instruction print count ($arr); Give an array $arr such that planets ($arr) prints Venus (ii)arrow_forwardQuestion 5 Write the code for the function printRoster, it should print out the contacts in a format similar to the example. You can use a range-based for-loop and auto to simplify the solution, if you wish. #include #include #include #include #include using namespace std; void printRoster(map>>& roster) { // Fill code here int main() { map>> roster; roster["ELCO"]["CS-30"]. emplace_back("Anthony Davis"); roster["ElCo"]"cS-30"j. emplace_back("Talen Horton-Tucker"); roster["ElCo"Ï"BUS-101"].emplace_back("LeBron James"); roster["SMC"]["CHEM-101"].emplace_back("Russell Westbrook"); printRoster(roster); return 0; Here is the sample output: ElCo BUS-101: LeBron James CS-30: Anthony Davis Talen Horton-Tucker SMC CHEM-101: Russell Westbrookarrow_forwardC++ Double Pointer: Can you draw picture of what this means : Food **table? struct Food { int expiration_date; string brand; } Food **table; What is this double pointer saying? I know its a 2D matrix but I don't understand what I'm dealing with here. Does the statement mean that we have an array of pointers of type Food and what is the other * mean? I'm confused.arrow_forward
- C++arrow_forwardFinish the following code: void Divide (int dividend, int divisor, bool& error, float& result) // Set error to indicate if divisor is zero. // If no error, set result to dividend / divisor. { using namespace std; // For debugging cout << "Function Divide entered." << endl; cout << "Dividend = " << dividend << endl; cout << "Divisor = " << divisor << endl; //** // Rest of code goes here. //** // For debugging if (error) cout << "Error = true "; else cout << "Error = false "; cout << "and Result = " << result << endl; cout << "Function Divide terminated." << endl; }arrow_forwardint main(int argc, char **argv) { float *rainfall; float rain_today; // rainfall has been dynamically allocated space for a floating point number. // Both rainfall and rain today have been initialized in hidden code. // Assign the amount in rain_today to the space rainfall points to. return 0; }arrow_forward
- Kindly fix the code:arrow_forward#include #include using namespace std; int main() { double pi = 0; long i; long n; cin >> n; cout << "Enter the value of n: "; cout << endl; if (i % 2 == 0) else pi = pi + pi = pi T - (1/(2* i + 1)); (1/(2*1 + 1)); for (i = 0; i < n; i++) } { pi = 0; pi 4 pi; } cout << endl << "pi = " << pi << endl; return 0;arrow_forwardConsider the function definition: void GetNums(int howMany, float& alpha, float& beta) { int i; beta = 0; for (i = 0; i < howMany; i++) { beta = alpha + beta; } } Describe what happens in MEMORY when the GetNums function is called.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
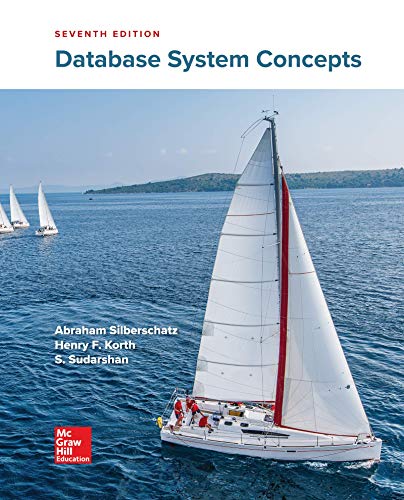
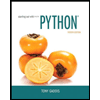
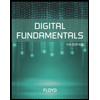
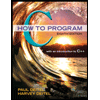
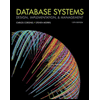
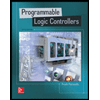