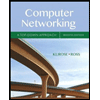
Please help,
#include <stdlib.h> // malloc/free and NULL
// Include the declarations of our string functions
#include "mystring.h"
// search: 'man strlen' to see what function should do
size_t my_strlen(const char* input){
// IMPLEMENT
return 0;
}
// search: 'man strcmp' to see what function should do
int my_strcmp(const char* src1, const char* src2){
// IMPLEMENT
return 0;
}
// search: 'man strchr' to see what function should do
char* my_strchr(const char* src1, int c){
// IMPLEMENT
return NULL;
}
// search: 'man strcat' to see what function should do
// Note: Our 'string append' is going to be slightly different
// than 'strcat'.
// I would like you to return a 'heap allocated' string
// that appends both of src1 and src2 into a new buffer
char* my_string_append(const char* src1, const char* src2){
// IMPLEMENT
return NULL;
}

Here i explain what function do:
==============================================================
1.size_t my_strlen(const char* input){
// IMPLEMENT
return 0;
This function is used to take input and give the length of the string in include file.
2.int my_strcmp(const char* src1, const char* src2){
// IMPLEMENT
return 0;
This function is used to compare both the string.
Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- Nested function classwork 2 Write 'taxIncome', a user-defined function that calculates income tax. y = taxincome (income) defines the principal function. The main function calculates adjusted income as income - 6000. Then y = compute Tax is called. For y = 0.28*Adjusted Income, this function uses 'Adjusted Income'. 'Adjusted Income' was defined in the main function. Use $80,000 income.arrow_forward# dates and times with lubridateinstall.packages("nycflights13") library(tidyverse)library(lubridate)library(nycflights13) Qustion: Create a function called date_quarter that accepts any vector of dates as its input and then returns the corresponding quarter for each date Examples: “2019-01-01” should return “Q1” “2011-05-23” should return “Q2” “1978-09-30” should return “Q3” Etc. Use the flight's data set from the nycflights13 package to test your function by creating a new column called quarter using mutate()arrow_forwardWrite a value-returning function that receives an array of structs of the type defined below and the length of the array as parameters. It should return as the value of the function the sum of the offspring amounts in the array. Note: you do not need a main function or to create an array. Assume the array already exists from main. #include <iostream>using namespace std; // struct declaration for Animal struct Animal { // a string for animal typestring animal_type;// a string for color string color;// an integer for number of offspring. int number_of_offspring;}; // Main function to declare a structure variable of type Animal. int main(){struct Animal A1;return 0;}arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
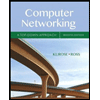
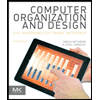
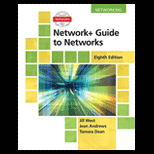
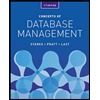
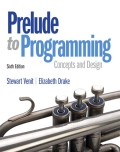
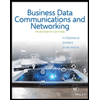