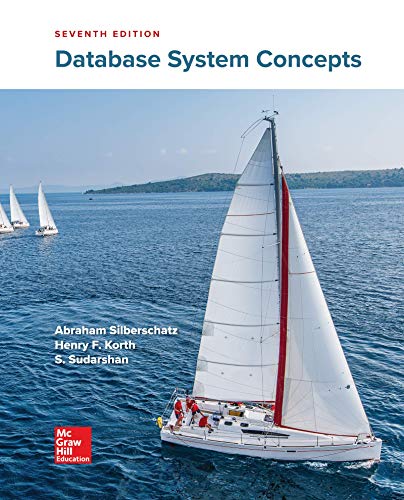
Concept explainers
The last part of this code I need the gallon round to the nearest number, but it still shows wrong after I apply the round(), I don't know how to change it
#include <iostream>
#include <cmath> // Note: Needed for math functions in part(3)
#include <iomanip> // For setprecision
using namespace std;
int main()
{
double wallHeight;
double wallWidth;
double wallArea;
cout << "Enter wall height (feet):" << endl;
cin >> wallHeight;
// FIXME (1): Prompt user to input wall's width
cout << "Enter wall width (feet):" << endl;
cin >> wallWidth;
// Calculate and output wall area
wallArea = wallHeight * wallWidth; // FIXME (1): Calculate the wall's area
cout << fixed << setprecision(2) << "Wall area: " << wallArea
<< " square feet" << endl;
// FIXME (1): Finish the output statement
// FIXME (2): Calculate and output the amount of paint in gallons needed to
//paint the wall
cout << fixed << setprecision(2) << "Paint needed: " << wallArea/350
<< " gallons" << endl; // FIXME (1): Finish the output statement
// FIXME (3): Calculate and output the number of 1 gallon cans needed to
// paint the wall, rounded up to nearest integer
cout << "Cans needed: " << round(wallArea/350) << "can(s)" << endl;
return 0;
}

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 1 images

- please could you help me in this code i need it for my class soon thank you ^^arrow_forwardC++ Programming: I need help writing a void function that prints sales details using 3 for loops. Please see my code below ... not sure how to write the function. But this is what i've got so far. Please leave main alone. What the output should look like is posted below the code. Thanks :) #include <iostream>#include <string>#include <iomanip> using namespace std; int const LOCATION_DIM_SZ = 2;int const TIME_DIM_SZ = 2;int const PRODUCT_DIM_SIZE = 3; string Location[] = { "FL", "TX" };string Period[] = { "Jan", "FEB" };string Product[] = { "iPhone","iPad","MacBookPro" }; /*1*/void printSalesDetails(double sales[LOCATION_DIM_SZ][TIME_DIM_SZ][PRODUCT_DIM_SIZE]) { int i(0), j(0), k(0);int sum = 0; cout << "Sales Details per Location per Period per Product" << endl;cout << "=================================================" << endl;for (i = 0; i < 2; i++){double salesPerLocation = 0;sum = 0;for (j = 0; j < 2; j++){double salesPerPeriod =…arrow_forwardIn C++ Find the five errors. For each error, state the line number, explain the error and show a correction. #include<iostream> #include<iomanip> using namespace std; class colorCode { public: void setRGB(int); //accepts an int parameter and sets the RGB to the value of the parameter void setName(string);//accepts a string parameter and sets the name of the object to the value of the parameter string getName() const;//returns the name of the object int getRGB() const;//returns the RGB of the object void changeColor();// adds 10 to the RGB value private: string name; int RGB; } int main() { colorCode paintCans[10]; int i; for (i = 0; i < 10; i++){ paintCans.setRGB[i] = 0;} paintCans[5].setName(GREEN); paintCan[5].setRGB(192000); cout << paintCans[5].getName << ' ' << paintCans[5].getRGB() << endl; return 0; }arrow_forward
- Please use the below code to write code for median and standard deviation. I have already written for Average, Min, and Max value. Thanks! #include <stdlib.h> #include <stdio.h> #include <pthread.h> int nums; int* vals; double avg; int min, max; void* myAvg(void* param) { avg = 0.0; for(inti = 0; i < nums; ++i) { avg += vals[i]; } avg /= nums; pthread_exit(0); } void* myMin(void* param) { min = vals[0]; for(inti = 1; i < nums; ++i) { if(min > vals[i]) min = vals[i]; } pthread_exit(0); } void* myMax(void* param) { max = vals[0]; for(inti = 1; i < nums; ++i) { if(max < vals[i]) max = vals[i]; } pthread_exit(0); } int main(int argc, char** argv) { int i; nums = (argc - 1); if(nums <= 0) { printf("you have to give atleat one value!\n"); return1; } vals =…arrow_forwardc++ pleasearrow_forwardIt is necessary to offer a description of the function known as GetStdHandle.arrow_forward
- C++ Given code is #pragma once#include <iostream>#include "ourvector.h"using namespace std; ourvector<int> intersect(ourvector<int> &v1, ourvector<int> &v2) { // TO DO: write this function return {};}arrow_forwardFor the following code #include <stdio.h> #include <conio.h> int powerof(int base, int pow); int _tmain(int argc, _TCHAR* argv[]) { int x,y,z; line 3 calledarrow_forwardThere are 5 mistakes in the code below, highlighted by the red tilde. Fix each mistake by rewriting the code to the right. Just rewrite the lines that are incorrect. Hint, you might have to change a line that doesn’t have a tilde.arrow_forward
- The last part of this code I need the "cans" to be a whole number. I don't know how to change it What I have right now below: #include <iostream>#include <cmath> // Note: Needed for math functions in part(3)#include <iomanip> // For setprecisionusing namespace std; int main(){double wallHeight;double wallWidth;double wallArea; cout << "Enter wall height (feet):" << endl;cin >> wallHeight; // FIXME (1): Prompt user to input wall's widthcout << "Enter wall width (feet):" << endl;cin >> wallWidth; // Calculate and output wall areawallArea = wallHeight * wallWidth; // FIXME (1): Calculate the wall's area cout << fixed << setprecision(2) << "Wall area: " << wallArea<< " square feet" << endl;// FIXME (1): Finish the output statement // FIXME (2): Calculate and output the amount of paint in gallons needed to//paint the wallcout << fixed << setprecision(2) << "Paint needed: " <<…arrow_forwardWhich aggregating operations are permitted for struct variables but not array variables, and which are prohibited for array variables?arrow_forward#include <stdio.h>#include <stdlib.h> int cent50 = 0;int cent20 = 0;int cent10 = 0;int cent05 = 0; //Function definitionvoid calculateChange(int change) {if(change > 0) {if(change >= 50) {change -= 50;cent50++;} else if(change >= 20) {change -= 20;cent20++;} else if(change >= 10) {change -= 10;cent10++;} else if(change >= 05) {change -= 05;cent05++;}calculateChange(change);}} //Define the functionvoid printChange() { if(cent50)printf("\n50 Cents : %d coins", cent50); if(cent20)printf("\n20 Cents : %d coins", cent20); if(cent10)printf("\n10 Cents : %d coins", cent10); if(cent05)printf("\n05 Cents : %d coins", cent05);cent50 = 0;cent20 = 0;cent10 = 0;cent05 = 0; } //Function's definitionint TakeChange() { int change;printf("\nEnter the amount : ");scanf("%d", &change);return change; }//main functionint main() {//call the functionint change = TakeChange(); //use while-loop to repeatedly ask for input to the userwhile(change != -1){if((change %…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
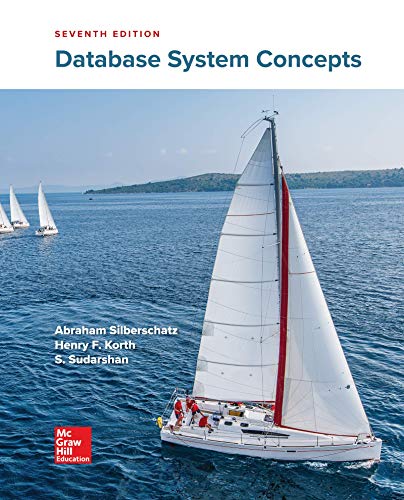
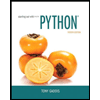
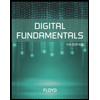
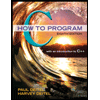
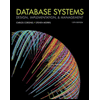
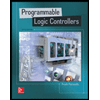